C语言实现页面置换算法: 最近最久未使用算法(LRU) : 淘汰最近最久未被使用的页面。
时间: 2024-03-03 15:49:08 浏览: 83
好的,我来给您讲一下C语言实现最近最久未使用算法(LRU)的方法。
LRU算法是一种常见的页面置换算法,其核心思想是淘汰最近最久未被使用的页面。在实现LRU算法时,我们需要维护一个页面访问的历史记录,即一个访问时间的队列。每次访问一个页面时,我们就将该页面的访问时间插入到队列的末尾。当需要淘汰一个页面时,我们就选择队列头部的页面进行淘汰,因为它是最久未被使用的页面。
下面是一个简单的C语言实现LRU算法的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define CACHE_SIZE 3 // 缓存大小为3
typedef struct node {
int data;
struct node *prev;
struct node *next;
} Node;
Node *head = NULL;
Node *tail = NULL;
int cache[CACHE_SIZE];
int is_cache_full() {
int i;
for (i = 0; i < CACHE_SIZE; i++) {
if (cache[i] == -1) {
return 0;
}
}
return 1;
}
void display_cache() {
int i;
printf("Cache content: ");
for (i = 0; i < CACHE_SIZE; i++) {
if (cache[i] != -1) {
printf("%d ", cache[i]);
}
}
printf("\n");
}
void move_to_tail(Node *node) {
if (node == tail) {
return;
}
if (node == head) {
head = head->next;
} else {
node->prev->next = node->next;
node->next->prev = node->prev;
}
node->prev = tail;
node->next = NULL;
tail->next = node;
tail = node;
}
int get_least_recently_used_page() {
int i;
int min_time = head->data;
int min_index = 0;
for (i = 0; i < CACHE_SIZE; i++) {
if (cache[i] != -1 && cache[i] < min_time) {
min_time = cache[i];
min_index = i;
}
}
return min_index;
}
void add_page_to_cache(int page) {
if (is_cache_full()) {
int index = get_least_recently_used_page();
cache[index] = -1;
Node *node_to_remove = head;
head = head->next;
head->prev = NULL;
free(node_to_remove);
}
int i;
for (i = 0; i < CACHE_SIZE; i++) {
if (cache[i] == -1) {
cache[i] = page;
Node *new_node = (Node *) malloc(sizeof(Node));
new_node->data = i;
new_node->prev = tail;
new_node->next = NULL;
if (head == NULL) {
head = new_node;
} else {
tail->next = new_node;
}
tail = new_node;
break;
}
}
}
void access_page(int page) {
int i;
for (i = 0; i < CACHE_SIZE; i++) {
if (cache[i] == page) {
move_to_tail(tail);
return;
}
}
add_page_to_cache(page);
}
int main() {
int i;
for (i = 0; i < CACHE_SIZE; i++) {
cache[i] = -1;
}
access_page(1);
display_cache();
access_page(2);
display_cache();
access_page(3);
display_cache();
access_page(4);
display_cache();
access_page(2);
display_cache();
access_page(5);
display_cache();
access_page(4);
display_cache();
access_page(1);
display_cache();
return 0;
}
```
在上面的示例代码中,我们使用了一个双向链表来维护页面的访问时间队列。每当访问一个页面时,我们将它对应的缓存位置中的值更新为当前时间,并将它加入到访问时间队列的末尾。当需要淘汰一个页面时,我们选择队列头部的页面进行淘汰。
当然,这只是一个简单的示例代码,实际上LRU算法的实现还需要考虑一些细节问题。例如,如果多个页面的访问时间相同,我们需要使用一些其他的策略来决定淘汰哪个页面。此外,LRU算法还可以使用哈希表来加速页面查找的过程。
阅读全文
相关推荐
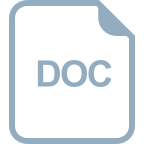
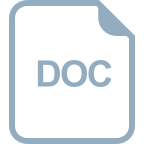
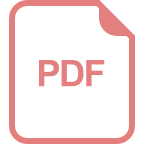
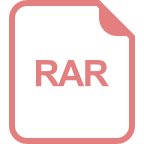
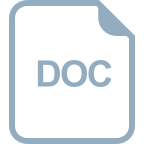
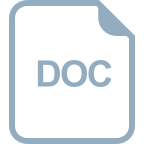
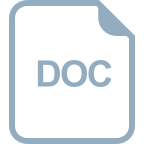
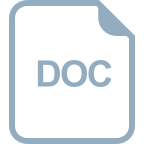
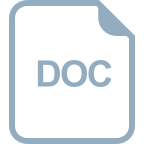
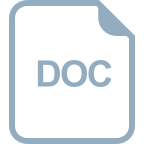





