bouncy castle 使用SM4加解密,请写出示例程序
时间: 2024-03-21 20:41:20 浏览: 11
以下是使用Bouncy Castle库进行SM4加解密的示例程序:
```java
import org.bouncycastle.crypto.BlockCipher;
import org.bouncycastle.crypto.engines.SM4Engine;
import org.bouncycastle.crypto.modes.CBCBlockCipher;
import org.bouncycastle.crypto.params.KeyParameter;
import org.bouncycastle.crypto.params.ParametersWithIV;
import org.bouncycastle.util.encoders.Hex;
import java.nio.charset.StandardCharsets;
import java.security.SecureRandom;
public class SM4Example {
public static void main(String[] args) {
// 待加密的数据
String plaintext = "Hello, world!";
byte[] plaintextBytes = plaintext.getBytes(StandardCharsets.UTF_8);
// 生成随机密钥和IV
byte[] key = new byte[16];
byte[] iv = new byte[16];
SecureRandom random = new SecureRandom();
random.nextBytes(key);
random.nextBytes(iv);
// 初始化SM4算法引擎和CBC模式
BlockCipher engine = new SM4Engine();
CBCBlockCipher cipher = new CBCBlockCipher(engine);
// 将密钥和IV转换为参数
KeyParameter keyParam = new KeyParameter(key);
ParametersWithIV params = new ParametersWithIV(keyParam, iv);
// 加密数据
cipher.init(true, params);
byte[] ciphertextBytes = new byte[cipher.getOutputSize(plaintextBytes.length)];
int len = cipher.processBytes(plaintextBytes, 0, plaintextBytes.length, ciphertextBytes, 0);
try {
cipher.doFinal(ciphertextBytes, len);
} catch (Exception e) {
e.printStackTrace();
}
String ciphertext = Hex.toHexString(ciphertextBytes);
System.out.println("加密后的数据:" + ciphertext);
// 解密数据
cipher.init(false, params);
byte[] decryptedBytes = new byte[cipher.getOutputSize(ciphertextBytes.length)];
len = cipher.processBytes(ciphertextBytes, 0, ciphertextBytes.length, decryptedBytes, 0);
try {
cipher.doFinal(decryptedBytes, len);
} catch (Exception e) {
e.printStackTrace();
}
String decryptedText = new String(decryptedBytes, StandardCharsets.UTF_8);
System.out.println("解密后的数据:" + decryptedText);
}
}
```
该示例程序使用Bouncy Castle库的SM4引擎和CBC模式对数据进行加解密,并将结果输出到控制台。需要注意的是,SM4算法使用128位密钥和128位IV,因此在示例程序中生成了随机的16字节密钥和IV。
相关推荐
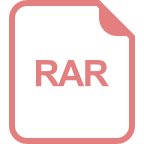














