可以向我展示一段包含unix 高级编程知识点的代码,要求使用并展示open()、close()、read()、write()、lseek()这些函数的所有用法,并在代码注释中详细的解释接口和参数的用法
时间: 2023-03-01 19:44:40 浏览: 85
好的,这是一段使用 C 语言的代码,用于读取文件并将其内容反转后写入另一个文件:
```
#include <fcntl.h>
#include <unistd.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define BUFSIZE 1024
int main(int argc, char *argv[]) {
int fd_in, fd_out;
ssize_t len_in, len_out;
char buf[BUFSIZE];
if (argc != 3) {
fprintf(stderr, "Usage: %s <input file> <output file>\n", argv[0]);
exit(1);
}
// 使用 open 函数打开输入文件
fd_in = open(argv[1], O_RDONLY);
if (fd_in == -1) {
perror("open input file failed");
exit(1);
}
// 使用 open 函数创建输出文件
fd_out = open(argv[2], O_CREAT | O_WRONLY | O_TRUNC, 0664);
if (fd_out == -1) {
perror("open output file failed");
close(fd_in);
exit(1);
}
// 使用 lseek 函数移动文件指针到文件末尾
if (lseek(fd_in, 0, SEEK_END) == -1) {
perror("seek failed");
close(fd_in);
close(fd_out);
exit(1);
}
// 使用 read 函数读取文件内容并将其反转后写入输出文件
while ((len_in = read(fd_in, buf, BUFSIZE)) > 0) {
for (int i = 0; i < len_in / 2; i++) {
char tmp = buf[i];
buf[i] = buf[len_in - i - 1];
buf[len_in - i - 1] = tmp;
}
// 使用 write 函数写入文件
len_out = write(fd_out, buf, len_in);
if (len_out == -1) {
perror("write failed");
close(fd_in);
close(fd_out);
exit(1);
}
}
// 使用 close 函数关闭文件
close(fd_in);
close(fd_out);
阅读全文
相关推荐
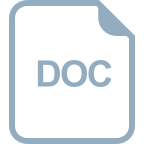
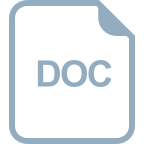
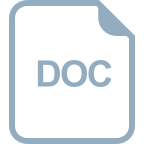




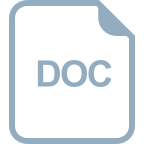
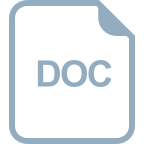
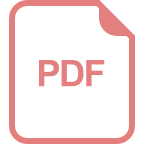