python二维DFT 的平移和旋转代码
时间: 2023-06-09 19:06:20 浏览: 83
我理解您的问题是关于二维 DFT 中平移和旋转的代码实现。以下是一个简单的 Python 代码实现:
平移:
假设我们有一个输入矩阵 `input_mat`,其大小为 `(rows, cols)`,要将其向右移动 `shift_x` 个像素,向下移动 `shift_y` 个像素。我们可以首先计算平移后的位置坐标,然后将 `input_mat` 中的值复制到对应位置上,最后计算出移位后的 DFT:
```
import numpy as np
def shift2d(input_mat, shift_x, shift_y):
rows, cols = input_mat.shape
x = np.arange(cols)
y = np.arange(rows)
xv, yv = np.meshgrid(x, y, indexing='ij')
shifted_xv = (xv + shift_x) % cols
shifted_yv = (yv + shift_y) % rows
output_mat = np.zeros_like(input_mat)
output_mat[shifted_yv, shifted_xv] = input_mat[yv, xv]
return np.fft.fft2(output_mat)
```
旋转:
假设我们有一个输入矩阵 `input_mat`,其大小为 `(rows, cols)`,要将其逆时针旋转 `angle` 度。我们可以首先计算出旋转后的位置坐标,然后将 `input_mat` 中的值复制到对应位置上,最后计算出旋转后的 DFT:
```
import numpy as np
def rotate2d(input_mat, angle):
rows, cols = input_mat.shape
x = np.arange(cols)
y = np.arange(rows)
xv, yv = np.meshgrid(x, y, indexing='ij')
rotated_xv = (xv - cols / 2) * np.cos(angle) - (yv - rows / 2) * np.sin(angle) + cols / 2
rotated_yv = (xv - cols / 2) * np.sin(angle) + (yv - rows / 2) * np.cos(angle) + rows / 2
output_mat = np.zeros_like(input_mat)
output_mat[rotated_yv, rotated_xv] = input_mat[yv, xv]
return np.fft.fft2(output_mat)
```
这里假设旋转中心为 `(cols / 2, rows / 2)`,可以根据实际情况进行调整。
阅读全文
相关推荐
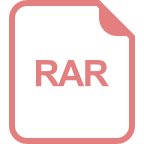




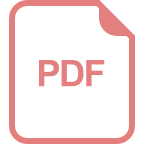
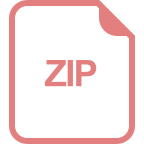
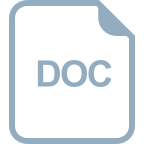
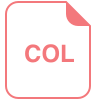
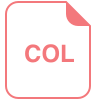
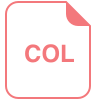

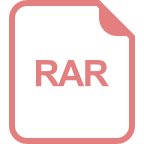
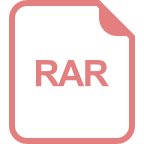
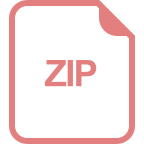