给上面这段代码优化一下
时间: 2023-05-29 08:07:45 浏览: 221
1. 使用 const 声明不会被修改的变量,可以提高代码的可读性和性能。
2. 使用模板来实现通用性更好的函数。
3. 使用 std::vector 来替代数组,可以更方便地进行动态内存管理。
4. 使用 std::size_t 来代替 int 类型,避免可能的越界错误。
5. 使用 range-based for 循环来遍历容器,可以使代码更加简洁易读。
6. 使用 auto 关键字来自动推导变量类型,增强代码的简洁性。
优化后的代码如下:
```c++
#include <iostream>
#include <vector>
template<typename T>
void printVector(const std::vector<T>& v)
{
for (const auto& item : v)
{
std::cout << item << " ";
}
std::cout << std::endl;
}
int main()
{
std::vector<int> v{ 1, 2, 3, 4, 5 };
printVector(v);
return 0;
}
```
相关问题
优化上面这段代码
可以考虑将重复的部分提取出来,并且使用更加语义化的变量名,如下所示:
```
# 声明变量
chatbot1 = "CSDN"
chatbot2 = "CSDN ChitGPT"
# 输出对话信息
print(f"您与{chatbot2}进行对话,{chatbot1}与之无关。")
```
这样代码更加简洁易懂,也更具可读性。
优化一下上面这段shell代码
```
#!/bin/bash
for file in $(find . -name "*.txt"); do
echo "Processing file: $file"
sed -i 's/old/new/g' $file
done
```
优化后的代码:
```
#!/bin/bash
find . -name "*.txt" -print0 | while IFS= read -r -d '' file; do
echo "Processing file: $file"
sed -i 's/old/new/g' "$file"
done
```
改进说明:
1. 使用 `-print0` 和 `read -r -d ''` 结合,可以正确处理文件名中包含空格或特殊字符的情况;
2. 将 `$file` 放在双引号中,可以避免路径中包含空格或特殊字符时出错。
阅读全文
相关推荐
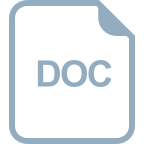
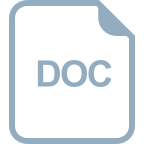
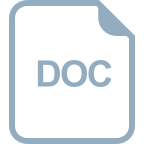













