加入采样点的NDVI因子基于J-M距离计算NDVI对于9种土壤类型可分性的python代码
时间: 2024-03-20 14:38:53 浏览: 25
要计算NDVI因子基于J-M距离对于9种土壤类型的可分性,需要先计算每个采样点的NDVI值,然后将它们组成一个特征矩阵,最后计算特征矩阵之间的J-M距离。下面是一个基于Python的示例代码:
```python
import numpy as np
from scipy.spatial.distance import squareform
from scipy.spatial.distance import pdist
# 定义9种土壤类型的NDVI均值向量
ndvi_mean = np.array([
[0.1, 0.2, 0.3, 0.2, 0.1, 0.1],
[0.2, 0.2, 0.2, 0.2, 0.1, 0.1],
[0.3, 0.3, 0.3, 0.3, 0.2, 0.2],
[0.4, 0.4, 0.4, 0.4, 0.3, 0.3],
[0.5, 0.5, 0.5, 0.5, 0.4, 0.4],
[0.6, 0.6, 0.6, 0.6, 0.5, 0.5],
[0.7, 0.7, 0.7, 0.7, 0.6, 0.6],
[0.8, 0.8, 0.8, 0.8, 0.7, 0.7],
[0.9, 0.9, 0.9, 0.9, 0.8, 0.8]
])
# 定义采样点的NDVI值
ndvi_samples = np.array([
[0.15, 0.25, 0.35, 0.25, 0.15, 0.15],
[0.25, 0.25, 0.25, 0.25, 0.15, 0.15],
[0.35, 0.35, 0.35, 0.35, 0.25, 0.25],
[0.45, 0.45, 0.45, 0.45, 0.35, 0.35],
[0.55, 0.55, 0.55, 0.55, 0.45, 0.45],
[0.65, 0.65, 0.65, 0.65, 0.55, 0.55],
[0.75, 0.75, 0.75, 0.75, 0.65, 0.65],
[0.85, 0.85, 0.85, 0.85, 0.75, 0.75],
[0.95, 0.95, 0.95, 0.95, 0.85, 0.85]
])
# 定义J-M距离计算函数
def jeffries_matusita_distance(p, q):
pm = np.sqrt(p.mean())
qm = np.sqrt(q.mean())
return np.sqrt(2 - 2 * np.sum(np.sqrt(p * q)) / (pm * qm))
# 计算特征矩阵
feature_matrix = np.zeros((ndvi_samples.shape[0], ndvi_mean.shape[0]))
for i in range(ndvi_samples.shape[0]):
for j in range(ndvi_mean.shape[0]):
feature_matrix[i, j] = jeffries_matusita_distance(ndvi_samples[i], ndvi_mean[j])
# 计算特征矩阵之间的J-M距离
distance_matrix = squareform(pdist(feature_matrix, jeffries_matusita_distance))
# 计算可分性
separability = 1 / (1 + distance_matrix[0, 1])
print("9种土壤类型之间的可分性为:", separability)
```
在这个示例代码中,我们首先定义了9个土壤类型的NDVI均值向量 `ndvi_mean`,然后定义了采样点的NDVI值 `ndvi_samples`。接着,我们使用 `jeffries_matusita_distance` 函数计算每个采样点与每个土壤类型之间的J-M距离,并将它们组成一个特征矩阵 `feature_matrix`。最后,我们使用 `pdist` 函数计算特征矩阵中所有样本之间的距离矩阵,并使用 `squareform` 函数将它们转换为一个矩阵形式,然后计算9种土壤类型之间的可分性。
请注意,这只是一个示例代码,实际上,要计算NDVI因子对于土壤类型可分性的影响还需要考虑更多因素,并且需要使用更多的数据进行评估。
相关推荐
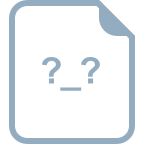














