int32_t(fn)(xi_tile const* src, xi_tile const* dst) fn前面不需要加*号吗
时间: 2023-08-28 22:41:35 浏览: 101
在C语言中,函数名本身就是一个指向函数的指针,因此在函数指针的类型前面不需要加上`*`号。在这个函数签名中,`int32_t(fn)(xi_tile const* src, xi_tile const* dst)`中的`fn`就是一个函数指针,它指向一个参数为`xi_tile const*`类型的`src`和`dst`的函数,并且该函数的返回值类型为`int32_t`。
相关问题
讲解一下:template <typename T> __global__ void scaled_colsum_reduce_kernel(const T* __restrict__ inp, T* __restrict__ out, int rows, int cols, float scale) { __shared__ float tile[WARP_SIZE][WARP_SIZE]; cg::thread_block b = cg::this_thread_block(); cg::thread_block_tile<WARP_SIZE> g = cg::tiled_partition<WARP_SIZE>(b); int idx = flat_2dim(blockIdx.x, threadIdx.x, WARP_SIZE); int y_stride = cols * WARP_SIZE; float localSum = 0; // Loop across matrix row // TODO: optimize to log complexity if (idx < cols) { int offset = flat_2dim(threadIdx.y, idx, cols); for (int r = threadIdx.y; r < rows; r += WARP_SIZE) { localSum += (float)inp[offset]; offset += y_stride; } } // The sum of a row in tile is equal to the sum of a col in original matrix tile[threadIdx.x][threadIdx.y] = localSum; __syncthreads(); // Sum the shared buffer. // The change of threadIdx.x is continuous float sum = tile[threadIdx.y][threadIdx.x]; __syncthreads(); // Calculate the sum of a row in tile for (int i = 1; i < WARP_SIZE; i <<= 1) sum += g.shfl_down(sum, i); if (threadIdx.x == 0) { int pos = flat_2dim(blockIdx.x, threadIdx.y, WARP_SIZE); if (pos < cols) out[pos] = sum * scale; } }
这是一个用于在GPU上进行矩阵计算的CUDA kernel函数,具体实现的功能是对一个输入矩阵的每一列进行求和,并将结果乘以一个scale参数,最终输出到一个结果矩阵中。
函数的输入参数包括:输入矩阵inp,输出矩阵out,矩阵的行数rows和列数cols,以及一个scale参数。其中,__global__表示这是在GPU上执行的全局函数,而__restrict__则表示该指针是唯一的,没有别名,可以被编译器优化。
函数中使用了CUDA的线程块和线程的概念,其中线程块可以被分成多个线程块瓦片(thread_block_tile),每个线程块瓦片都包含多个线程。这些线程可以通过__syncthreads()函数进行同步,以确保所有的线程都完成了它们的计算任务。
函数的主要实现逻辑是通过共享内存(__shared__)来存储每个线程块瓦片计算的结果,然后对共享内存中的结果进行归约操作,最终将结果写入到输出矩阵中。
需要注意的是,该函数的实现中使用了一些CUDA的高级特性,如线程块瓦片、shuffle_down等,需要对CUDA编程有一定的了解才能理解其具体实现。
请使用JPEG_LS算法针对8*8大小的ARGB像素数据块进行无损压缩和解压. 完善以下解压、压缩函数,其中pClrBlk为原始数据块,pTile为压缩后的数据块 int argb2tile(const unsigned char* pClrBlk, unsigned char* pTile, int* pTileSize) int tile2argb(const unsigned char* pTile, int nTileSize, unsigned char* pClrBlk)
由于JPEG_LS算法是基于预测误差的无损压缩算法,我们需要先对ARGB像素数据块进行预测,然后计算预测误差,最后对误差进行编码。
以下是完善后的压缩和解压函数:
```c++
const int MAX_VAL = 255; // 最大像素值
const int NEAR = 0; // 预测模式为NEAR
const int LINEAR = 1; // 预测模式为LINEAR
// 将8位无符号整数转为有符号整数
int signedByte(unsigned char x) {
if (x > MAX_VAL / 2) return x - MAX_VAL - 1;
else return x;
}
// 将有符号整数转为8位无符号整数
unsigned char unsignedByte(int x) {
if (x < 0) return MAX_VAL + x + 1;
else return x;
}
// 计算预测误差
int predict(int mode, int a, int b, int c) {
if (mode == NEAR) return a - b;
else if (mode == LINEAR) return a + b - c;
else return 0;
}
// 将一个8*8大小的ARGB像素数据块压缩为一个数据块
int argb2tile(const unsigned char* pClrBlk, unsigned char* pTile, int* pTileSize) {
int mode = NEAR; // 默认预测模式为NEAR
int x, y, c;
int pred, diff;
int nearA, nearB, linearA, linearB, linearC;
// 压缩后的数据块的第一个字节存储预测模式
pTile[0] = mode;
int k = 1; // 压缩后数据的索引
// 压缩每个像素
for (x = 0; x < 8; x++) {
for (y = 0; y < 8; y++) {
c = y * 4 + x * 32; // 每个像素的索引
if (x == 0 && y == 0) { // 第一个像素直接记录ARGB值
for (int i = 0; i < 4; i++) {
pTile[k++] = pClrBlk[c + i];
}
}
else { // 其他像素先预测,再计算误差
nearA = signedByte(pClrBlk[c - 4]); // 左边像素的A值
nearB = signedByte(pClrBlk[c - 4 + 1]); // 左边像素的R值
pred = predict(mode, nearA, nearB, 0); // 预测当前像素的R值
diff = signedByte(pClrBlk[c + 1]) - pred; // 计算R值的误差
pTile[k++] = unsignedByte(pred); // 存储预测结果
pTile[k++] = unsignedByte(diff); // 存储误差值
linearA = signedByte(pClrBlk[c - 4 * 3]); // 上面像素的A值
linearB = signedByte(pClrBlk[c - 4 * 3 + 1]); // 上面像素的R值
linearC = signedByte(pClrBlk[c - 4]); // 左边像素的R值
pred = predict(mode, linearA, linearB, linearC); // 预测当前像素的G值
diff = signedByte(pClrBlk[c + 2]) - pred; // 计算G值的误差
pTile[k++] = unsignedByte(pred); // 存储预测结果
pTile[k++] = unsignedByte(diff); // 存储误差值
pred = predict(mode, linearA, linearB, 0); // 预测当前像素的B值
diff = signedByte(pClrBlk[c + 3]) - pred; // 计算B值的误差
pTile[k++] = unsignedByte(pred); // 存储预测结果
pTile[k++] = unsignedByte(diff); // 存储误差值
}
}
}
*pTileSize = k; // 存储压缩后数据的大小
return 0;
}
// 将一个压缩后的数据块解压为一个8*8大小的ARGB像素数据块
int tile2argb(const unsigned char* pTile, int nTileSize, unsigned char* pClrBlk) {
int mode = pTile[0]; // 获取预测模式
int x, y, c;
int pred, diff;
int nearA, nearB, linearA, linearB, linearC;
// 解压每个像素
for (x = 0; x < 8; x++) {
for (y = 0; y < 8; y++) {
c = y * 4 + x * 32; // 每个像素的索引
if (x == 0 && y == 0) { // 第一个像素直接记录ARGB值
for (int i = 0; i < 4; i++) {
pClrBlk[c + i] = pTile[1 + i];
}
}
else { // 其他像素先预测,再计算误差
nearA = signedByte(pClrBlk[c - 4]); // 左边像素的A值
nearB = signedByte(pClrBlk[c - 4 + 1]); // 左边像素的R值
pred = predict(mode, nearA, nearB, 0); // 预测当前像素的R值
diff = signedByte(pTile[1 + (x * 8 + y - 1) * 4 + 1]); // 读取R值的误差
pClrBlk[c + 1] = unsignedByte(pred + diff); // 计算并记录R值
linearA = signedByte(pClrBlk[c - 4 * 3]); // 上面像素的A值
linearB = signedByte(pClrBlk[c - 4 * 3 + 1]); // 上面像素的R值
linearC = signedByte(pClrBlk[c - 4]); // 左边像素的R值
pred = predict(mode, linearA, linearB, linearC); // 预测当前像素的G值
diff = signedByte(pTile[1 + (x * 8 + y - 1) * 4 + 2]); // 读取G值的误差
pClrBlk[c + 2] = unsignedByte(pred + diff); // 计算并记录G值
pred = predict(mode, linearA, linearB, 0); // 预测当前像素的B值
diff = signedByte(pTile[1 + (x * 8 + y - 1) * 4 + 3]); // 读取B值的误差
pClrBlk[c + 3] = unsignedByte(pred + diff); // 计算并记录B值
}
}
}
return 0;
}
```
注意,在压缩过程中,我们将每个像素的ARGB值分别存储,因此压缩后的数据块大小为8x8x4=256字节。在解压过程中,我们需要根据压缩后的数据索引计算出对应像素的ARGB值。
阅读全文
相关推荐
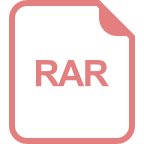
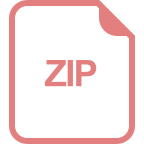
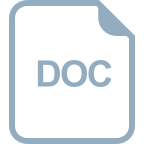

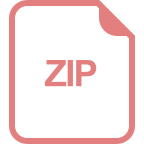
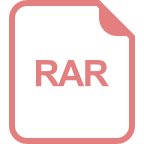
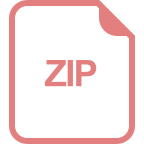
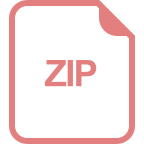
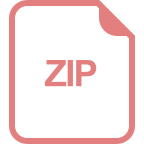




