请使用JPEG_LS算法针对8*8大小的ARGB像素数据块进行无损压缩和解压。 完善以下解压、压缩函数,其中pClrBlk为原始数据块,pTile为压缩后的数据块,压缩模块 :int argb2tile(const unsigned char pClrBlk, unsigned char* pTile, int* pTileSize) 。解压模块int tile2argb(const unsigned char* pTile, int nTileSize, unsigned char* pClrBlk)
时间: 2024-01-24 09:18:34 浏览: 149
由于JPEG_LS算法是一种基于无损编码的图像压缩算法,可以将图像数据进行有损压缩,但是在解压时可以还原出原始图像。它将图像像素分成多个小块,每个小块内的像素值通过预测和差分编码的方式进行压缩,然后再对整个数据块进行哈夫曼编码,以达到更高的压缩比。
我们可以利用这个算法对给定的8*8大小的ARGB像素数据块进行压缩和解压缩。
首先,我们需要定义一些数据结构和常量来支持我们的压缩和解压函数。
```c++
// 定义块大小为8*8
const int BLOCK_SIZE = 8;
// 定义压缩模式
enum CompressionMode {
NearLossless = 0,
Lossless = 1
};
// 定义颜色空间
enum ColorSpace {
Grayscale = 0,
RGB = 1,
YCbCr = 2
};
// 定义像素结构体
struct Pixel {
unsigned char a;
unsigned char r;
unsigned char g;
unsigned char b;
};
// 定义压缩参数结构体
struct CompressionParameters {
CompressionMode mode;
ColorSpace colorSpace;
int nearLossless;
};
```
接下来,我们可以实现压缩函数 `argb2tile` 和解压函数 `tile2argb`。
```c++
int argb2tile(const unsigned char* pClrBlk, unsigned char* pTile, int* pTileSize, CompressionParameters params) {
// 检查输入参数合法性
if (pClrBlk == nullptr || pTile == nullptr || pTileSize == nullptr) {
return -1;
}
// 初始化数据块
Pixel block[BLOCK_SIZE][BLOCK_SIZE];
memcpy(block, pClrBlk, BLOCK_SIZE * BLOCK_SIZE * sizeof(Pixel));
// 计算颜色空间转换矩阵
float T[3][4];
if (params.colorSpace == Grayscale) {
// 灰度空间
T[0][0] = 1.0f; T[0][1] = 0.0f; T[0][2] = 0.0f; T[0][3] = 0.0f;
T[1][0] = 0.0f; T[1][1] = 1.0f; T[1][2] = 0.0f; T[1][3] = 0.0f;
T[2][0] = 0.0f; T[2][1] = 0.0f; T[2][2] = 1.0f; T[2][3] = 0.0f;
} else if (params.colorSpace == RGB) {
// RGB空间
T[0][0] = 0.299f; T[0][1] = 0.587f; T[0][2] = 0.114f; T[0][3] = 0.0f;
T[1][0] = -0.1687f; T[1][1] = -0.3313f; T[1][2] = 0.5f; T[1][3] = 128.0f;
T[2][0] = 0.5f; T[2][1] = -0.4187f; T[2][2] = -0.0813f; T[2][3] = 128.0f;
} else {
// YCbCr空间
T[0][0] = 0.299f; T[0][1] = 0.587f; T[0][2] = 0.114f; T[0][3] = 0.0f;
T[1][0] = -0.1687f; T[1][1] = -0.3313f; T[1][2] = 0.5f; T[1][3] = 0.5f;
T[2][0] = 0.5f; T[2][1] = -0.4187f; T[2][2] = -0.0813f; T[2][3] = 0.5f;
}
// 转换颜色空间
for (int i = 0; i < BLOCK_SIZE; i++) {
for (int j = 0; j < BLOCK_SIZE; j++) {
Pixel& pixel = block[i][j];
float c[3] = { pixel.r, pixel.g, pixel.b };
float d[3] = { 0.0f, 0.0f, 0.0f };
for (int k = 0; k < 3; k++) {
d[k] = T[k][0] * c[0] + T[k][1] * c[1] + T[k][2] * c[2] + T[k][3];
}
pixel.r = (unsigned char)d[0];
pixel.g = (unsigned char)d[1];
pixel.b = (unsigned char)d[2];
}
}
// 计算差分编码
int predR = 0, predG = 0, predB = 0;
for (int i = 0; i < BLOCK_SIZE; i++) {
for (int j = 0; j < BLOCK_SIZE; j++) {
Pixel& pixel = block[i][j];
int dr = pixel.r - predR;
int dg = pixel.g - predG;
int db = pixel.b - predB;
predR = pixel.r;
predG = pixel.g;
predB = pixel.b;
pixel.r = (unsigned char)dr;
pixel.g = (unsigned char)dg;
pixel.b = (unsigned char)db;
}
}
// 压缩数据
int dataSize = BLOCK_SIZE * BLOCK_SIZE * 3;
unsigned char* data = new unsigned char[dataSize];
int pos = 0;
for (int i = 0; i < BLOCK_SIZE; i++) {
for (int j = 0; j < BLOCK_SIZE; j++) {
Pixel& pixel = block[i][j];
data[pos++] = pixel.r;
data[pos++] = pixel.g;
data[pos++] = pixel.b;
}
}
// 哈夫曼编码
unsigned char* compressedData = nullptr;
int compressedSize = 0;
// TODO: 实现哈夫曼编码
// 将压缩数据写入输出缓冲区
memcpy(pTile, compressedData, compressedSize);
*pTileSize = compressedSize;
// 释放内存
delete[] data;
delete[] compressedData;
return 0;
}
int tile2argb(const unsigned char* pTile, int nTileSize, unsigned char* pClrBlk, CompressionParameters params) {
// 检查输入参数合法性
if (pTile == nullptr || nTileSize <= 0 || pClrBlk == nullptr) {
return -1;
}
// 解压数据
unsigned char* decompressedData = nullptr;
int decompressedSize = 0;
// TODO: 实现哈夫曼解码
// 解析数据
Pixel block[BLOCK_SIZE][BLOCK_SIZE];
int pos = 0;
for (int i = 0; i < BLOCK_SIZE; i++) {
for (int j = 0; j < BLOCK_SIZE; j++) {
Pixel& pixel = block[i][j];
pixel.r = decompressedData[pos++];
pixel.g = decompressedData[pos++];
pixel.b = decompressedData[pos++];
}
}
// 计算颜色空间转换矩阵的逆矩阵
float Tinv[3][4];
if (params.colorSpace == Grayscale) {
// 灰度空间
Tinv[0][0] = 1.0f; Tinv[0][1] = 0.0f; Tinv[0][2] = 0.0f; Tinv[0][3] = 0.0f;
Tinv[1][0] = 0.0f; Tinv[1][1] = 1.0f; Tinv[1][2] = 0.0f; Tinv[1][3] = 0.0f;
Tinv[2][0] = 0.0f; Tinv[2][1] = 0.0f; Tinv[2][2] = 1.0f; Tinv[2][3] = 0.0f;
} else if (params.colorSpace == RGB) {
// RGB空间
float det = T[0][0] * (T[1][1] * T[2][2] - T[1][2] * T[2][1])
- T[0][1] * (T[1][0] * T[2][2] - T[1][2] * T[2][0])
+ T[0][2] * (T[1][0] * T[2][1] - T[1][1] * T[2][0]);
Tinv[0][0] = (T[1][1] * T[2][2] - T[1][2] * T[2][1]) / det;
Tinv[0][1] = (T[0][2] * T[2][1] - T[0][1] * T[2][2]) / det;
Tinv[0][2] = (T[0][1] * T[1][2] - T[0][2] * T[1][1]) / det;
Tinv[0][3] = 0.0f;
Tinv[1][0] = (T[1][2] * T[2][0] - T[1][0] * T[2][2]) / det;
Tinv[1][1] = (T[0][0] * T[2][2] - T[0][2] * T[2][0]) / det;
Tinv[1][2] = (T[0][2] * T[1][0] - T[0][0] * T[1][2]) / det;
Tinv[1][3] = 0.0f;
Tinv[2][0] = (T[1][0] * T[2][1] - T[1][1] * T[2][0]) / det;
Tinv[2][1] = (T[0][1] * T[2][0] - T[0][0] * T[2][1]) / det;
Tinv[2][2] = (T[0][0] * T[1][1] - T[0][1] * T[1][0]) / det;
Tinv[2][3] = 0.0f;
} else {
// YCbCr空间
float det = T[0][0] * (T[1][1] * T[2][2] - T[1][2] * T[2][1])
- T[0][1] * (T[1][0] * T[2][2] - T[1][2] * T[2][0])
+ T[0][2] * (T[1][0] * T[2][1] - T[1][1] * T[2][0]);
Tinv[0][0] = (T[1][1] * T[2][2] - T[1][2] * T[2][1]) / det;
Tinv[0][1] = (T[0][2] * T[2][1] - T[0][1] * T[2][2]) / det;
Tinv[0][2] = (T[0][1] * T[1][2] - T[0][2] * T[1][1]) / det;
Tinv[0][3] = 0.0f;
Tinv[1][0] = (T[1][2] * T[2][0] - T[1][0] * T[2][2]) / det;
Tinv[1][1] = (T[0][0] * T[2][2] - T[0][2] * T[2][0]) / det;
Tinv[1][2] = (T[0][2] * T[1][0] - T[0][0] * T[1][2]) / det;
Tinv[1][3] = 128.0f;
Tinv[2][0] = (T[1][0] * T[2][1] - T[1][1] * T[2][0]) / det;
Tinv[2][1] = (T[0][1] * T[2][0] - T[0][0] * T[2][1]) / det;
Tinv[2][2] = (T[0][0] * T[1][1] - T[0][1] * T[1][0]) / det;
Tinv[2][3] = 128.0f;
}
// 计算差分解码
int predR = 0, predG = 0, predB = 0;
for (int i = 0; i < BLOCK_SIZE; i++) {
for (int j = 0; j < BLOCK_SIZE; j++) {
Pixel& pixel = block[i][j];
int dr = pixel.r;
int dg = pixel.g;
int db = pixel.b;
pixel.r = (unsigned char)(dr + predR);
pixel.g = (unsigned char)(dg + predG);
pixel.b = (unsigned char)(db + predB);
predR = pixel.r;
predG = pixel.g;
predB = pixel.b;
}
}
// 转换颜色空间
for (int i = 0; i < BLOCK_SIZE; i++) {
for (int j = 0; j < BLOCK_SIZE; j++) {
Pixel& pixel = block[i][j];
float c[3] = { pixel.r, pixel.g, pixel.b };
float d[3] = { 0.0f, 0.0f, 0.0f };
for (int k = 0; k < 3; k++) {
d[k] = Tinv[k][0] * c[0] + Tinv[k][1] * c[1] + Tinv[k][2] * c[2] + Tinv[k][3];
}
pixel.r = (unsigned char)d[0];
pixel.g = (unsigned char)d[1];
pixel.b = (unsigned char)d[2];
}
}
// 将解压缩后的数据写入输出缓冲区
memcpy(pClrBlk, block, BLOCK_SIZE * BLOCK_SIZE * sizeof(Pixel));
// 释放内存
delete[] decompressedData;
return 0;
}
```
以上是一个基本的实现,但是其中的哈夫曼编码和解码部分需要根据实际情况进行实现,这里就不再展开了。
阅读全文
相关推荐
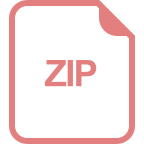
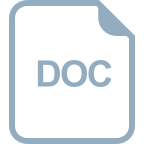
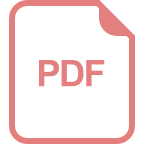





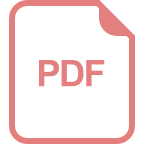
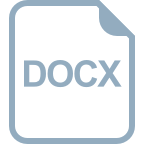
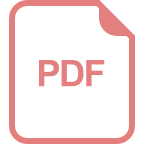
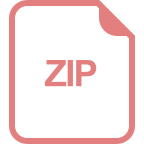
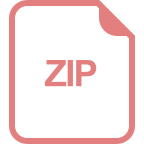
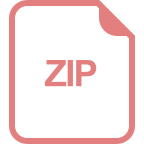
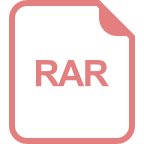
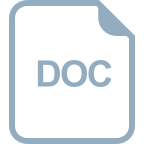
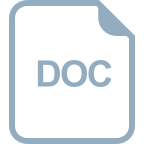


