像素格式是 ARGB_8888,代表什么
时间: 2023-04-10 12:04:50 浏览: 158
ARGB_8888 是一种像素格式,其中 A 表示 Alpha 通道,R 表示红色通道,G 表示绿色通道,B 表示蓝色通道,8888 表示每个通道使用 8 位来表示,因此总共需要 32 位来表示一个像素。这种像素格式通常用于图像处理和游戏开发中。
相关问题
安卓,已知byte[]像素数组,怎么转为bitmap
可以使用以下代码将 byte[] 像素数组转换为 Bitmap:
```
byte[] pixels = ...; // 像素数组
int width = ...; // 图像宽度
int height = ...; // 图像高度
Bitmap bitmap = Bitmap.createBitmap(width, height, Bitmap.Config.ARGB_8888);
bitmap.copyPixelsFromBuffer(ByteBuffer.wrap(pixels));
```
注意,在上述代码中,我们假设像素数组的格式为 ARGB_8888,也就是说,每个像素由 4 个字节表示,分别代表透明度、红色、绿色和蓝色通道。
如果像素数组的格式不是 ARGB_8888,则需要使用其他的 Bitmap.Config 值来替换 ARGB_8888。例如,如果像素数组的格式是 RGB_565,则应将 ARGB_8888 替换为 RGB_565。
希望这能帮到你!
假设txt文件里面是16进制的像素信息,帮我分到ARGB四个矩阵里面
如果你的txt文件中包含16进制的像素信息,并且你想将其分成ARGB四个矩阵,请使用以下代码:
```matlab
filename = 'example.txt'; % 替换成你的txt文件路径
fileID = fopen(filename,'r');
data = fscanf(fileID,'%x');
fclose(fileID);
% 假设每个像素的信息占据4个十六进制数
num_pixels = numel(data) / 4;
data = reshape(data, 4, num_pixels)'; % 转置矩阵,使得每行代表一个像素
% 拆分成ARGB四个矩阵
A = bitshift(bitand(data, hex2dec('FF000000')), -24);
R = bitshift(bitand(data, hex2dec('00FF0000')), -16);
G = bitshift(bitand(data, hex2dec('0000FF00')), -8);
B = bitand(data, hex2dec('000000FF'));
% 显示结果
disp(A);
disp(R);
disp(G);
disp(B);
```
在这个例子中,我们首先从txt文件中读取16进制的像素信息,并将其存储在`data`变量中。然后,我们根据假设每个像素信息占据4个十六进制数,计算出像素的数量,并将`data`重塑为一个矩阵,其中每行代表一个像素。
接下来,我们使用位运算和位掩码操作来从每个像素的十六进制值中提取出ARGB四个分量。最后,我们将每个分量存储在对应的矩阵变量中,并使用`disp`函数显示结果。
请注意,这段代码假设每个像素的十六进制值存储为32位整数,并且按照ARGB的顺序排列。如果你的情况有所不同,请相应地修改代码。
相关推荐
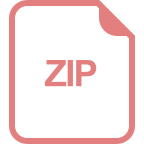
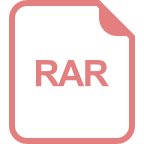












