CShap 是否为二叉搜索树 源代码
时间: 2024-05-14 12:14:55 浏览: 18
以下是C#实现二叉搜索树的代码:
```
using System;
namespace BinarySearchTree
{
class Node
{
public int data;
public Node left;
public Node right;
public Node(int value)
{
data = value;
left = null;
right = null;
}
}
class BinarySearchTree
{
Node root;
public BinarySearchTree()
{
root = null;
}
public void Insert(int value)
{
Node newNode = new Node(value);
if (root == null)
{
root = newNode;
}
else
{
Node current = root;
Node parent;
while (true)
{
parent = current;
if (value < current.data)
{
current = current.left;
if (current == null)
{
parent.left = newNode;
break;
}
}
else
{
current = current.right;
if (current == null)
{
parent.right = newNode;
break;
}
}
}
}
}
public bool IsBST()
{
return IsBSTUtil(root, int.MinValue, int.MaxValue);
}
private bool IsBSTUtil(Node node, int min, int max)
{
if (node == null)
return true;
if (node.data < min || node.data > max)
return false;
return (IsBSTUtil(node.left, min, node.data - 1) && IsBSTUtil(node.right, node.data + 1, max));
}
}
class Program
{
static void Main(string[] args)
{
BinarySearchTree bst = new BinarySearchTree();
bst.Insert(50);
bst.Insert(30);
bst.Insert(20);
bst.Insert(40);
bst.Insert(70);
bst.Insert(60);
bst.Insert(80);
if (bst.IsBST())
Console.WriteLine("The tree is a BST");
else
Console.WriteLine("The tree is not a BST");
Console.ReadLine();
}
}
}
```
在这个实现中,我们定义了 `IsBST()` 方法来检查树是否是二叉搜索树。这个方法调用了 `IsBSTUtil()` 方法,该方法递归地检查每个节点是否符合二叉搜索树的性质。如果一个节点的值不在它的允许范围内,那么这个方法会返回 false,否则它会递归地检查该节点的左子树和右子树。在递归的过程中,我们需要传递每个节点允许的最小值和最大值,以确保每个节点都符合二叉搜索树的性质。
相关推荐
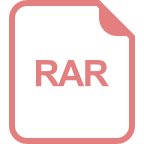














