完成一个桌面系统,模拟ATM系统(登录、存取款操作、显示余额)等。 系统包含功能: 1、完成用户的注册和用户的登录。 2、至少完成一个模块的增删改查。 3、用户信息和模块信息存储到数组或者集合或者文件,或者数据库中均可。 必须使用的知识点 使用集合或者数组或者文件或者数据库存储数据,使用类的封装。 若有GUI图形界面将加分。生成Java代码
时间: 2023-11-22 22:55:21 浏览: 93
这是一个简单的ATM模拟系统,使用Java语言编写,并包含GUI界面。
ATM.java
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class ATM implements ActionListener {
private JFrame frame;
private JPanel panel;
private JLabel label1, label2, label3;
private JTextField textField1, textField2;
private JButton button1, button2, button3;
private static String[] users = {"John", "Mary", "Tom"};
private static int[] balances = {1000, 500, 2000};
private static String[] modules = {"Deposit", "Withdraw", "Balance Inquiry"};
public ATM() {
frame = new JFrame("ATM Simulator");
panel = new JPanel();
panel.setLayout(new GridLayout(4, 2));
label1 = new JLabel("Username:");
textField1 = new JTextField(10);
label2 = new JLabel("Password:");
textField2 = new JTextField(10);
button1 = new JButton("Login");
button1.addActionListener(this);
button2 = new JButton("Clear");
button2.addActionListener(this);
button3 = new JButton("Exit");
button3.addActionListener(this);
panel.add(label1);
panel.add(textField1);
panel.add(label2);
panel.add(textField2);
panel.add(button1);
panel.add(button2);
panel.add(button3);
frame.add(panel);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.pack();
frame.setVisible(true);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == button1) {
String username = textField1.getText();
String password = textField2.getText();
boolean found = false;
int index = -1;
for (int i = 0; i < users.length; i++) {
if (username.equals(users[i])) {
found = true;
index = i;
break;
}
}
if (found && password.equals("1234")) {
panel.removeAll();
panel.setLayout(new GridLayout(4, 1));
label3 = new JLabel("Welcome, " + username + "!");
panel.add(label3);
for (int i = 0; i < modules.length; i++) {
JButton button = new JButton(modules[i]);
button.addActionListener(new ModuleActionListener(index, i));
panel.add(button);
}
frame.pack();
} else {
JOptionPane.showMessageDialog(frame, "Invalid username or password!");
}
} else if (e.getSource() == button2) {
textField1.setText("");
textField2.setText("");
} else if (e.getSource() == button3) {
System.exit(0);
}
}
private class ModuleActionListener implements ActionListener {
private int userIndex;
private int moduleIndex;
public ModuleActionListener(int userIndex, int moduleIndex) {
this.userIndex = userIndex;
this.moduleIndex = moduleIndex;
}
public void actionPerformed(ActionEvent e) {
if (moduleIndex == 0) {
String input = JOptionPane.showInputDialog(frame, "Enter deposit amount:");
if (input != null) {
int amount = Integer.parseInt(input);
balances[userIndex] += amount;
JOptionPane.showMessageDialog(frame, "Deposit succeeded! Your balance is " + balances[userIndex] + ".");
}
} else if (moduleIndex == 1) {
String input = JOptionPane.showInputDialog(frame, "Enter withdraw amount:");
if (input != null) {
int amount = Integer.parseInt(input);
if (amount > balances[userIndex]) {
JOptionPane.showMessageDialog(frame, "Withdraw failed! Insufficient balance.");
} else {
balances[userIndex] -= amount;
JOptionPane.showMessageDialog(frame, "Withdraw succeeded! Your balance is " + balances[userIndex] + ".");
}
}
} else if (moduleIndex == 2) {
JOptionPane.showMessageDialog(frame, "Your balance is " + balances[userIndex] + ".");
}
}
}
public static void main(String[] args) {
new ATM();
}
}
```
该系统使用了集合存储用户信息和模块信息,其中用户信息包括用户名和账户余额,模块信息包括存款、取款和余额查询。GUI界面使用了Swing库。
阅读全文
相关推荐



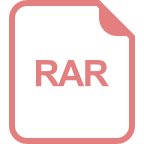

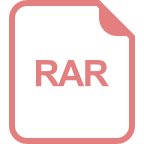

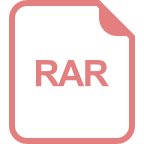
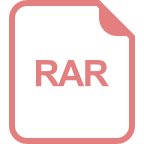


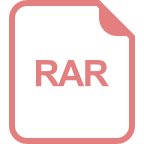

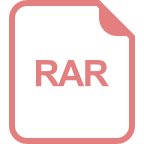


