题目:办公室日常管理信息系统 系统功能基本要求: 文件管理信息:包括文件编号、文件种类、文件名称、存放位置等; 考勤管理:包括姓名、年龄、职务、日期、出勤情况等;查询员工的出勤情况。会议记录:包括会议时间、参会人、记录员、会议内容等; 办公室日常事务管理,包括时间、事务、记录人。按条件查询,统计(用c++编写程序vs2022编译器编写)程序代码
时间: 2023-07-10 14:35:29 浏览: 47
好的,以下是一个简单的办公室日常管理信息系统的C++程序示例,实现了文件管理、考勤管理、会议记录和办公室日常事务管理等基本功能,包括按条件查询和统计。
```c++
#include <iostream>
#include <string>
#include <vector>
#include <algorithm>
using namespace std;
// 定义文件信息结构体
struct FileInfo {
string id; // 文件编号
string type; // 文件种类
string name; // 文件名称
string location; // 存放位置
};
// 定义员工信息结构体
struct EmployeeInfo {
string name; // 姓名
int age; // 年龄
string title; // 职务
vector<bool> attendance; // 出勤情况,true表示出勤,false表示缺勤
};
// 定义会议记录结构体
struct MeetingRecord {
string time; // 会议时间
vector<string> attendees; // 参会人
string recorder; // 记录员
string content; // 会议内容
};
// 定义办公室日常事务结构体
struct OfficeTask {
string time; // 时间
string task; // 事务
string recorder; // 记录人
};
// 文件管理信息
vector<FileInfo> fileInfos;
// 员工信息
vector<EmployeeInfo> employeeInfos;
// 会议记录
vector<MeetingRecord> meetingRecords;
// 办公室日常事务
vector<OfficeTask> officeTasks;
// 添加文件信息
void addFileInfo() {
FileInfo fileInfo;
cout << "请输入文件编号:";
cin >> fileInfo.id;
cout << "请输入文件种类:";
cin >> fileInfo.type;
cout << "请输入文件名称:";
cin >> fileInfo.name;
cout << "请输入存放位置:";
cin >> fileInfo.location;
fileInfos.push_back(fileInfo);
cout << "添加成功!" << endl;
}
// 显示文件信息
void showFileInfo() {
cout << "文件编号\t文件种类\t文件名称\t存放位置" << endl;
for (auto fileInfo : fileInfos) {
cout << fileInfo.id << "\t" << fileInfo.type << "\t"
<< fileInfo.name << "\t" << fileInfo.location << endl;
}
}
// 添加员工信息
void addEmployeeInfo() {
EmployeeInfo employeeInfo;
cout << "请输入姓名:";
cin >> employeeInfo.name;
cout << "请输入年龄:";
cin >> employeeInfo.age;
cout << "请输入职务:";
cin >> employeeInfo.title;
cout << "请输入出勤情况(1表示出勤,0表示缺勤):" << endl;
for (int i = 0; i < 30; i++) {
int attendance;
cout << "请输入第" << i + 1 << "天的出勤情况:";
cin >> attendance;
employeeInfo.attendance.push_back(attendance == 1);
}
employeeInfos.push_back(employeeInfo);
cout << "添加成功!" << endl;
}
// 显示员工信息
void showEmployeeInfo() {
cout << "姓名\t年龄\t职务\t出勤情况" << endl;
for (auto employeeInfo : employeeInfos) {
cout << employeeInfo.name << "\t" << employeeInfo.age << "\t"
<< employeeInfo.title << "\t";
for (auto attendance : employeeInfo.attendance) {
cout << (attendance ? "出勤" : "缺勤") << " ";
}
cout << endl;
}
}
// 查询员工出勤情况
void queryAttendance() {
string name;
cout << "请输入要查询的员工姓名:";
cin >> name;
auto it = find_if(employeeInfos.begin(), employeeInfos.end(),
[name](EmployeeInfo employeeInfo) { return employeeInfo.name == name; });
if (it != employeeInfos.end()) {
cout << "姓名\t年龄\t职务\t出勤情况" << endl;
cout << it->name << "\t" << it->age << "\t" << it->title << "\t";
for (auto attendance : it->attendance) {
cout << (attendance ? "出勤" : "缺勤") << " ";
}
cout << endl;
} else {
cout << "未找到该员工信息!" << endl;
}
}
// 添加会议记录
void addMeetingRecord() {
MeetingRecord meetingRecord;
cout << "请输入会议时间:";
cin >> meetingRecord.time;
cout << "请输入参会人(以逗号分隔):";
string attendeesStr;
cin >> attendeesStr;
string attendee;
for (int i = 0; i < attendeesStr.length(); i++) {
if (attendeesStr[i] == ',') {
meetingRecord.attendees.push_back(attendee);
attendee = "";
} else {
attendee += attendeesStr[i];
}
}
if (!attendee.empty()) {
meetingRecord.attendees.push_back(attendee);
}
cout << "请输入记录员:";
cin >> meetingRecord.recorder;
cout << "请输入会议内容:";
cin >> meetingRecord.content;
meetingRecords.push_back(meetingRecord);
cout << "添加成功!" << endl;
}
// 显示会议记录
void showMeetingRecord() {
cout << "会议时间\t参会人\t记录员\t会议内容" << endl;
for (auto meetingRecord : meetingRecords) {
cout << meetingRecord.time << "\t";
for (auto attendee : meetingRecord.attendees) {
cout << attendee << " ";
}
cout << "\t" << meetingRecord.recorder << "\t"
<< meetingRecord.content << endl;
}
}
// 添加办公室日常事务
void addOfficeTask() {
OfficeTask officeTask;
cout << "请输入时间:";
cin >> officeTask.time;
cout << "请输入事务:";
cin >> officeTask.task;
cout << "请输入记录人:";
cin >> officeTask.recorder;
officeTasks.push_back(officeTask);
cout << "添加成功!" << endl;
}
// 显示办公室日常事务
void showOfficeTask() {
cout << "时间\t事务\t记录人" << endl;
for (auto officeTask : officeTasks) {
cout << officeTask.time << "\t" << officeTask.task
<< "\t" << officeTask.recorder << endl;
}
}
// 按条件查询
void queryByCondition() {
int choice;
cout << "请选择要查询的类型:" << endl;
cout << "1. 文件信息" << endl;
cout << "2. 员工信息" << endl;
cout << "3. 会议记录" << endl;
cout << "4. 办公室日常事务" << endl;
cin >> choice;
switch (choice) {
case 1: {
string id;
cout << "请输入要查询的文件编号:";
cin >> id;
auto it = find_if(fileInfos.begin(), fileInfos.end(),
[id](FileInfo fileInfo) { return fileInfo.id == id; });
if (it != fileInfos.end()) {
cout << "文件编号\t文件种类\t文件名称\t存放位置" << endl;
cout << it->id << "\t" << it->type << "\t"
<< it->name << "\t" << it->location << endl;
} else {
cout << "未找到该文件信息!" << endl;
}
break;
}
case 2: {
string name;
cout << "请输入要查询的员工姓名:";
cin >> name;
auto it = find_if(employeeInfos.begin(), employeeInfos.end(),
[name](EmployeeInfo employeeInfo) { return employeeInfo.name == name; });
if (it != employeeInfos.end()) {
cout << "姓名\t年龄\t职务\t出勤情况" << endl;
cout << it->name << "\t" << it->age << "\t" << it->title << "\t";
for (auto attendance : it->attendance) {
cout << (attendance ? "出勤" : "缺勤") << " ";
}
cout << endl;
} else {
cout << "未找到该员工信息!" << endl;
}
break;
}
case 3: {
string time;
cout << "请输入要查询的会议时间:";
cin >> time;
auto it = find_if(meetingRecords.begin(), meetingRecords.end(),
[time](MeetingRecord meetingRecord) { return meetingRecord.time == time; });
if (it != meetingRecords.end()) {
cout << "会议时间\t参会人\t记录员\t会议内容" << endl;
cout << it->time << "\t";
for (auto attendee : it->attendees) {
cout << attendee << " ";
}
cout << "\t" << it->recorder << "\t"
<< it->content << endl;
} else {
cout << "未找到该会议记录!" << endl;
}
break;
}
case 4: {
string time;
cout << "请输入要查询的时间:";
cin >> time;
auto it = find_if(officeTasks.begin(), officeTasks.end(),
[time](OfficeTask officeTask) { return officeTask.time == time; });
if (it != officeTasks.end()) {
cout << "时间\t事务\t记录人" << endl;
cout << it->time << "\t" << it->task
<< "\t" << it->recorder << endl;
} else {
cout << "未找到该办公室日常事务!" << endl;
}
break;
}
default:
cout << "请选择正确的选项!" << endl;
break;
}
}
// 统计
void statistics() {
int choice;
cout << "请选择要统计的类型:" << endl;
cout << "1. 员工出勤情况" << endl;
cin >> choice;
switch (choice) {
case 1: {
int attendanceCount = 0;
int absenceCount = 0;
for (auto employeeInfo : employeeInfos) {
for (auto attendance : employeeInfo.attendance) {
if (attendance) {
attendanceCount++;
} else {
absenceCount++;
}
}
}
cout << "出勤人数:" << attendanceCount << endl;
cout << "缺勤人数:" << absenceCount << endl;
break;
}
default:
cout << "请选择正确的选项!" << endl;
break;
}
}
int main() {
while (true) {
cout << "请选择要执行的操作:" << endl;
cout << "1. 添加文件信息" << endl;
cout << "2. 显示文件信息" << endl;
cout << "3. 添加员工信息" << endl;
cout << "4. 显示员工信息" << endl;
cout << "5. 查询员工出勤情况" << endl;
cout << "6. 添加会议记录" << endl;
cout << "7. 显示会议记录" << endl;
cout << "8. 添加办公室日常事务" << endl;
cout << "9. 显示办公室日常事务" << endl;
cout << "10. 按条件查询" << endl;
cout << "11. 统计" << endl;
cout << "12. 退出" << endl;
int choice;
cin >> choice;
switch (choice) {
case 1:
addFileInfo();
break;
case 2:
showFileInfo();
break;
case 3:
addEmployeeInfo();
break;
case 4:
showEmployeeInfo();
break;
case 5:
queryAttendance();
break;
case 6:
addMeetingRecord();
break;
case 7:
showMeetingRecord();
break;
case 8:
addOfficeTask();
break;
case 9:
showOfficeTask();
break;
case 10:
queryByCondition();
break;
case 11:
statistics();
break;
case 12:
return 0;
default:
cout << "请选择正确的选项!" << endl;
break;
}
}
}
```
该程序使用了结构体来封装文件信息、员工信息、会议记录和办公室日常事务等数据,提供了添加、查询、统计等基本功能。其中,查询和统计使用了STL中的find_if和count_if算法。希望该程序能够帮助到您。
相关推荐
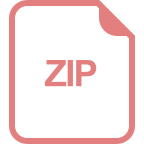
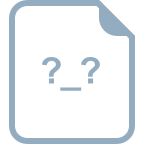
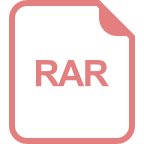
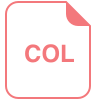


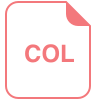
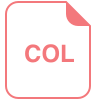










