python将图片像素输出为txt
时间: 2023-08-11 14:05:53 浏览: 378
可以使用Python中的Pillow库来将图片像素输出为txt,具体步骤如下:
1. 安装Pillow库:在命令行中输入`pip install pillow`进行安装。
2. 导入Pillow库:在Python代码中导入Pillow库,代码如下:
```python
from PIL import Image
```
3. 打开图片:使用Pillow库中的`open()`函数打开要处理的图片,代码如下:
```python
img = Image.open('image.jpg')
```
4. 转换为灰度图像:使用Pillow库中的`convert()`函数将图片转换为灰度图像,代码如下:
```python
img_gray = img.convert('L')
```
5. 获取像素值:使用Pillow库中的`getdata()`函数获取灰度图像的像素值,代码如下:
```python
pixels = img_gray.getdata()
```
6. 将像素值输出为txt文件:使用Python中的文件操作函数将像素值输出为txt文件,代码如下:
```python
with open('pixels.txt', 'w') as f:
for pixel in pixels:
f.write(str(pixel) + '\n')
```
这段代码将像素值逐行输出到名为`pixels.txt`的txt文件中。
完整代码示例:
```python
from PIL import Image
# 打开图片
img = Image.open('image.jpg')
# 转换为灰度图像
img_gray = img.convert('L')
# 获取像素值
pixels = img_gray.getdata()
# 将像素值输出为txt文件
with open('pixels.txt', 'w') as f:
for pixel in pixels:
f.write(str(pixel) + '\n')
```
注意:这种方法只适用于小型图片,对于大型图片可能会导致内存溢出,需要采用分块读取像素值的方法。
阅读全文
相关推荐
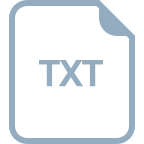




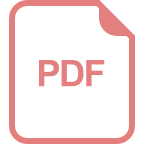
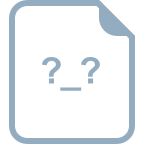
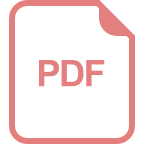
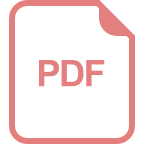
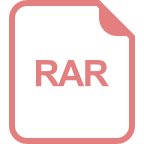
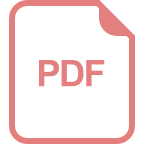
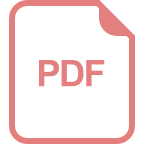
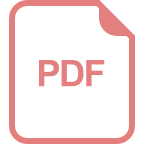
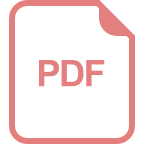
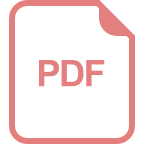
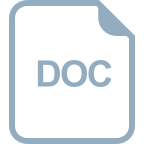

