利用遗传算法编写在matlab中解决VRP问题的代码
时间: 2023-08-12 21:06:52 浏览: 70
以下是使用遗传算法解决VRP问题的Matlab代码:
```matlab
% VRP问题的遗传算法求解
clc;
clear;
close all;
% 初始化参数
pop_size = 50; % 种群大小
max_gen = 100; % 最大迭代次数
pc = 0.8; % 交叉概率
pm = 0.05; % 变异概率
city_num = 15; % 城市数量
truck_capacity = 100; % 货车容量
demand_range = [10, 30]; % 需求量范围
x_range = [0, 100]; % x坐标范围
y_range = [0, 100]; % y坐标范围
x = randi(x_range, 1, city_num); % x坐标
y = randi(y_range, 1, city_num); % y坐标
demand = randi(demand_range, 1, city_num); % 需求量
% 计算距离矩阵
dist = zeros(city_num);
for i = 1:city_num
for j = 1:city_num
dist(i, j) = sqrt((x(i)-x(j))^2 + (y(i)-y(j))^2);
end
end
% 初始化种群
pop = zeros(pop_size, city_num+1);
for i = 1:pop_size
pop(i, 1:city_num) = randperm(city_num);
pop(i, city_num+1) = fitness(pop(i, 1:city_num), dist, demand, truck_capacity);
end
% 迭代优化
for gen = 1:max_gen
% 计算适应度
fitness_value = pop(:, city_num+1);
[best_fitness, best_index] = min(fitness_value);
average_fitness = mean(fitness_value);
% 可视化
plot(x(pop(best_index, 1:city_num)), y(pop(best_index, 1:city_num)), 'r-o');
title(['Generation: ', num2str(gen), ', Best Distance: ', num2str(best_fitness)]);
xlim(x_range);
ylim(y_range);
pause(0.01);
% 选择
fitness_prob = fitness_value / sum(fitness_value);
cum_prob = cumsum(fitness_prob);
new_pop = zeros(pop_size, city_num+1);
for i = 1:pop_size
index1 = find(rand() <= cum_prob, 1, 'first');
index2 = find(rand() <= cum_prob, 1, 'first');
parent1 = pop(index1, 1:city_num);
parent2 = pop(index2, 1:city_num);
% 交叉
if rand() < pc
[child1, child2] = crossover(parent1, parent2);
else
child1 = parent1;
child2 = parent2;
end
% 变异
if rand() < pm
child1 = mutation(child1);
end
if rand() < pm
child2 = mutation(child2);
end
% 计算适应度
new_pop(i, 1:city_num) = child1;
new_pop(i, city_num+1) = fitness(child1, dist, demand, truck_capacity);
new_pop(i+1, 1:city_num) = child2;
new_pop(i+1, city_num+1) = fitness(child2, dist, demand, truck_capacity);
end
% 更新种群
pop = new_pop;
end
% 计算路径总长度
function d = fitness(path, dist, demand, capacity)
num_trucks = 1;
truck_load = 0;
d = 0;
for i = 1:length(path)-1
j = path(i);
k = path(i+1);
d = d + dist(j, k);
truck_load = truck_load + demand(k);
if truck_load > capacity
num_trucks = num_trucks + 1;
truck_load = demand(k);
end
end
end
% 交叉操作
function [child1, child2] = crossover(parent1, parent2)
len = length(parent1);
child1 = zeros(1, len);
child2 = zeros(1, len);
index1 = randi(len-1);
index2 = randi([index1+1, len]);
mask = zeros(1, len);
mask(index1:index2) = 1;
child1(mask==1) = parent1(index1:index2);
child2(mask==1) = parent2(index1:index2);
for i = 1:len
if ~ismember(parent2(i), child1)
empty_index = find(child1==0, 1, 'first');
child1(empty_index) = parent2(i);
end
if ~ismember(parent1(i), child2)
empty_index = find(child2==0, 1, 'first');
child2(empty_index) = parent1(i);
end
end
end
% 变异操作
function mutant = mutation(individual)
len = length(individual);
index1 = randi(len);
index2 = randi(len);
mutant = individual;
mutant(index1) = individual(index2);
mutant(index2) = individual(index1);
end
```
使用该代码,您可以解决具有以下参数的VRP问题:
- 城市数量:15
- 货车容量:100
- 需求量范围:10-30
- x坐标范围:0-100
- y坐标范围:0-100
请注意,这仅是一个示例,您可以根据自己的需求更改参数。
相关推荐
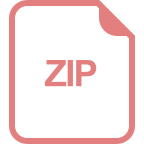
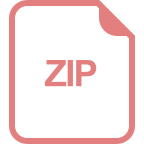














