重写下列代码中的addStudent function throw a runtime_error if the number of students exceeds the capacity:#include<iostream> #include"course.h" using namespace std; Course::Course(const string& courseName, int capacity) { numberOfStudents = 0; this->courseName = courseName; this->capacity = capacity; students = new string[capacity]; } Course::~Course(){ delete[]students; } string Course::getCourseName() const { return courseName; } void Course::addStudent(const string& name) { students[numberOfStudents] = name; numberOfStudents++; } string* Course::getStudent()const { return students; } int Course::getNumberOfStudents()const { return numberOfStudents; } int main() { Course course1("Data Structures", 10); Course course2("Database System", 15); course1.addStudent("s1mple"); course1.addStudent("kennys"); course1.addStudent("somedieyoung"); course2.addStudent("niko"); course2.addStudent("pinkfloyd"); cout << "Number of c1 students:" << course1.getNumberOfStudents() << endl; string* students = course1.getStudent(); for (int i = 0; i < course1.getNumberOfStudents(); i++) { cout << students[i] << "," << endl;; } cout << "Number of c2 students:" << course2.getNumberOfStudents() << endl; students = course2.getStudent(); for (int i = 0; i < course2.getNumberOfStudents(); i++) { cout << students[i] << ","; } return 0; }
时间: 2024-01-23 20:02:32 浏览: 86
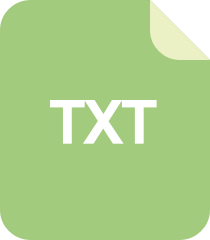
#include"iostream.h"

void Course::addStudent(const string& name) {
if (numberOfStudents >= capacity) {
throw runtime_error("Exceeds capacity!");
}
students[numberOfStudents] = name;
numberOfStudents++;
}
在上述代码中,我们在addStudent函数中添加了一个条件判断,如果学生人数超出了容量,则会抛出一个runtime_error类型的异常,并且携带一个错误信息("Exceeds capacity!")。
在main函数中,我们可以使用try-catch语句来捕获并处理可能抛出的异常,如下所示:
```
try {
course1.addStudent("s1mple");
course1.addStudent("kennys");
course1.addStudent("somedieyoung");
course1.addStudent("coldzera"); // Throws an exception
}
catch (runtime_error& e) {
cerr << "Error: " << e.what() << endl;
}
```
在上述代码中,我们在addStudent函数中添加了一个新的学生("coldzera"),这会导致抛出一个异常,因为学生人数已经超出了容量。在try块中,我们尝试添加这个学生,并通过catch块捕获并处理了异常,输出了相应的错误信息。
阅读全文
相关推荐













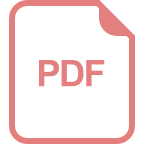
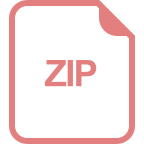