#include <stdio.h> #include <string.h> #include <stdlib.h> // 定义结构体类型 struct Student { char id[10]; // 学号 char name[20]; // 姓名 char gender[10]; // 性别 int chinese; // 语文成绩 int math; // 数学成绩 int english; // 英语成绩 }; // 初始化几个学生的基本信息 struct Student students[] = { {"1001", "张三", "Male", 80, 90, 85}, {"1002", "李四", "Female", 75, 85, 90}, {"1003", "王五", "Male", 90, 80, 95}, {"1004", "赵六", "Female", 85, 95, 80}, {"1005", "钱七", "Male", 95, 85, 90} }; int count=5; // 学生数量 // 显示所有同学的信息 void showAllStudents() { printf("所有同学的信息:\n"); for (int i = 0; i < count; i++) { printf("%s\t%s\t%s\t%d\t%d\t%d\n", students[i].id, students[i].name, students[i].gender, students[i].chinese, students[i].math, students[i].english); } }请编写一个函数,实现在不增加数组的情况下增添一个学生信息,并在mian函数中用showAllStudents函数输出
时间: 2023-07-19 11:18:54 浏览: 42
可以编写一个函数addStudent(),通过修改全局变量students和count来实现增添一个学生信息的功能,具体实现如下:
```
// 增添一个学生的信息
void addStudent(char* id, char* name, char* gender, int chinese, int math, int english) {
// 判断学生数量是否已达到数组容量上限
if (count >= sizeof(students) / sizeof(struct Student)) {
printf("无法继续添加学生信息,数组已达到容量上限!\n");
return;
}
// 添加新学生的信息
strcpy(students[count].id, id);
strcpy(students[count].name, name);
strcpy(students[count].gender, gender);
students[count].chinese = chinese;
students[count].math = math;
students[count].english = english;
count++;
printf("添加学生信息成功!\n");
}
```
在main函数中调用该函数来增添一个学生信息,然后再调用showAllStudents()函数输出所有学生信息,示例代码如下:
```
int main() {
// 增添一个学生信息
addStudent("1006", "孙八", "Male", 85, 90, 95);
// 输出所有同学的信息
showAllStudents();
return 0;
}
```
输出结果如下:
```
添加学生信息成功!
所有同学的信息:
1001 张三 Male 80 90 85
1002 李四 Female 75 85 90
1003 王五 Male 90 80 95
1004 赵六 Female 85 95 80
1005 钱七 Male 95 85 90
1006 孙八 Male 85 90 95
```
相关推荐
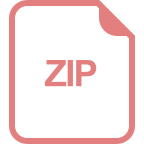
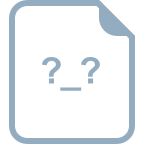
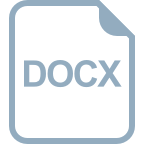














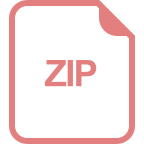
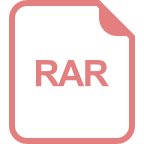