C语言编写一个21点游戏
时间: 2024-05-03 20:19:36 浏览: 82
下面是一个简单的C语言实现21点游戏的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
int main()
{
int player_total = 0;
int dealer_total = 0;
int card;
char choice;
srand(time(NULL)); // 初始化随机数种子
// 玩家抽第一张牌
card = rand() % 10 + 1;
printf("你抽到了一张 %d 点的牌\n", card);
player_total += card;
// 庄家抽第一张牌
card = rand() % 10 + 1;
printf("庄家抽到了一张 %d 点的牌\n", card);
dealer_total += card;
// 玩家抽第二张牌
card = rand() % 10 + 1;
printf("你抽到了一张 %d 点的牌\n", card);
player_total += card;
// 庄家抽第二张牌
card = rand() % 10 + 1;
printf("庄家抽到了一张未翻开的牌\n");
// 玩家决定是否继续抽牌
printf("你的点数总和为 %d 点,是否继续抽牌?(y/n)\n", player_total);
scanf(" %c", &choice);
while (choice == 'y' || choice == 'Y')
{
card = rand() % 10 + 1;
printf("你抽到了一张 %d 点的牌\n", card);
player_total += card;
if (player_total > 21)
{
printf("你的点数总和为 %d 点,已经超过了21点,你输了!\n", player_total);
return 0;
}
printf("你的点数总和为 %d 点,是否继续抽牌?(y/n)\n", player_total);
scanf(" %c", &choice);
}
// 庄家决定是否继续抽牌
printf("庄家翻开了他的第二张牌,是 %d 点\n", card);
while (dealer_total < 17)
{
card = rand() % 10 + 1;
printf("庄家抽到了一张 %d 点的牌\n", card);
dealer_total += card;
}
printf("庄家的点数总和为 %d 点\n", dealer_total);
// 判断胜负
if (dealer_total > 21)
{
printf("庄家的点数总和为 %d 点,已经超过了21点,你赢了!\n", dealer_total);
}
else if (dealer_total >= player_total)
{
printf("你的点数总和为 %d 点,庄家的点数总和为 %d 点,你输了!\n", player_total, dealer_total);
}
else
{
printf("你的点数总和为 %d 点,庄家的点数总和为 %d 点,你赢了!\n", player_total, dealer_total);
}
return 0;
}
```
这个程序实现了一个简单的21点游戏,首先玩家和庄家各抽两张牌,然后玩家决定是否继续抽牌,直到点数超过21点或者选择不再抽牌。接着庄家按照规定继续抽牌,直到达到17点或者超过21点。最后判断双方点数大小,决定胜负。
阅读全文
相关推荐
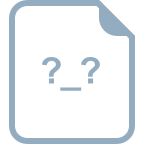
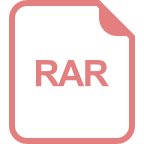
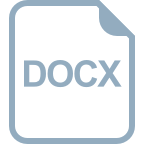
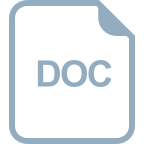
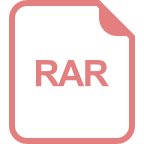
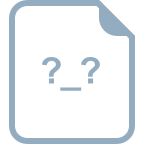
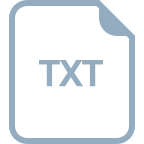
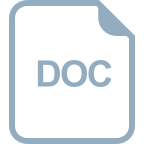
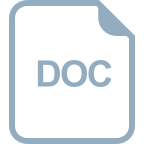


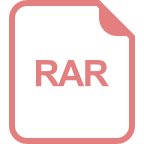
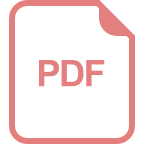
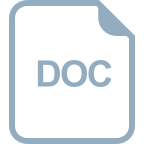
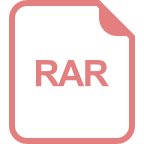
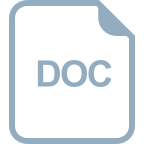
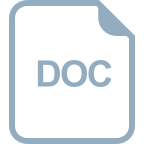