java 怎么调用python函数
时间: 2023-09-25 22:06:23 浏览: 156
### 回答1:
在Java中调用Python函数的方法有很多种。一种方法是使用Java调用Python脚本的方式来执行Python代码。这需要使用到Java中的 `Runtime.getRuntime().exec()` 方法。
例如:
```
String pythonScriptPath = "path/to/python/script.py";
String[] cmd = new String[2];
cmd[0] = "python"; // check version of installed python: python -V
cmd[1] = pythonScriptPath;
// create runtime to execute external command
Runtime rt = Runtime.getRuntime();
Process pr = rt.exec(cmd);
// retrieve output from python script
BufferedReader bfr = new BufferedReader(new InputStreamReader(pr.getInputStream()));
String line = "";
while((line = bfr.readLine()) != null) {
// process each output line form python script
System.out.println(line);
}
```
另一种方法是使用第三方库,例如 Jython 或 JPype,来在Java代码中直接调用Python函数。
使用Jython,你可以这样调用Python函数:
```
import org.python.util.PythonInterpreter;
PythonInterpreter interpreter = new PythonInterpreter();
interpreter.exec("from module_name import function_name");
interpreter.exec("result = function_name(param1, param2)");
PyObject result = interpreter.get("result");
```
使用JPype,你可以这样调用Python函数:
```
import JPype;
JPype.startJVM(JPype.getDefaultJVMPath());
JPype.importModule("module_name");
function = JPype.JClass("module_name").function_name;
result = function(param1, param2);
JPype.shutdownJVM();
```
请注意,在使用上述方法之前,你需要确保在你的系统中已经安装了Python解释器和所需的Python模块。
### 回答2:
在Java中调用Python函数通常有以下几种方法:
1. 使用Java的Runtime类来执行Python脚本。可以使用Runtime类的exec方法调用python命令,并在命令中指定要执行的Python脚本文件路径。例如:
```java
import java.io.*;
public class CallPythonFunc {
public static void main(String[] args) throws IOException {
// 执行Python脚本
Process process = Runtime.getRuntime().exec("python /path/to/python_script.py");
// 读取Python脚本的输出
BufferedReader reader = new BufferedReader(new InputStreamReader(process.getInputStream()));
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
reader.close();
}
}
```
2. 使用Jython库,它是Java与Python的集成引擎。可以在Java代码中直接调用Python函数或执行Python脚本。首先需要导入Jython库,然后创建一个Python解释器,通过Interpreter类的exec方法执行Python脚本或通过Interpreter类的get方法获取Python函数并调用。例如:
```java
import org.python.util.PythonInterpreter;
public class CallPythonFunc {
public static void main(String[] args) {
// 创建Python解释器
PythonInterpreter interpreter = new PythonInterpreter();
// 执行Python脚本
interpreter.exec("print('Hello, Python!')");
// 调用Python函数
interpreter.exec("def add(a, b):\n" +
" return a + b\n");
interpreter.exec("result = add(2, 3)");
Object result = interpreter.get("result");
System.out.println("Result: " + result);
}
}
```
3. 使用第三方库例如JPYTHON或Py4J。这些库可以在Java中直接调用Python函数,并提供了更多高级特性和操作方式。
这些方法根据具体需求可以选择适合自己的方式来调用Python函数。
### 回答3:
要在Java中调用Python函数,可以使用Java提供的"ProcessBuilder"类来执行Python脚本。
以下是一个简单的示例代码:
```java
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class PythonCaller {
public static void main(String[] args) {
try {
// 创建ProcessBuilder对象,并指定要调用的Python脚本路径
ProcessBuilder pb = new ProcessBuilder("python", "path/to/python_script.py");
// 执行脚本
Process process = pb.start();
// 获取脚本输出
BufferedReader reader = new BufferedReader(new InputStreamReader(process.getInputStream()));
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
// 等待脚本执行完成
int exitCode = process.waitFor();
System.out.println("脚本执行完成,退出码:" + exitCode);
} catch (IOException e) {
e.printStackTrace();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
```
请替换代码中的"path/to/python_script.py"为你实际的Python脚本路径。
注意,调用Python函数时,需要确保Java运行环境中已经安装了Python,并且Python的可执行文件路径已经加入到了系统的PATH环境变量中。
以上的代码仅是一个简单示例,如果需要传递参数给Python函数,可以通过修改ProcessBuilder对象的命令行参数来实现。具体可以参考ProcessBuilder的文档。
另外,也可以使用类似jython这样的库,它可以在Java中直接解析和执行Python代码,而无需依赖外部进程。
阅读全文
相关推荐
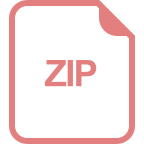
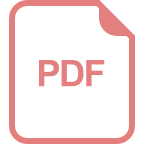
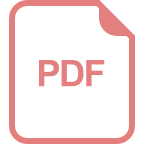















