牛郎织女迷宫问题C# 要求输出正确路径
时间: 2023-09-07 15:14:12 浏览: 82
以下是使用C#语言实现DFS并输出正确路径的代码:
```c#
using System;
namespace Maze
{
class Program
{
const int N = 10; // 最大迷宫大小
static int n, m; // 迷宫大小
static int sx, sy, ex, ey; // 起点和终点坐标
static int[,] maze = new int[N, N]; // 迷宫数组
static bool[,] st = new bool[N, N]; // 标记数组
static int[,] path = new int[N, N]; // 记录路径
// 搜索函数
static bool Dfs(int x, int y)
{
// 如果到达终点,返回true
if (x == ex && y == ey) return true;
// 标记当前位置已经访问过
st[x, y] = true;
// 枚举四个方向
int[] dx = { -1, 0, 1, 0 }, dy = { 0, 1, 0, -1 };
for (int i = 0; i < 4; i++)
{
int a = x + dx[i], b = y + dy[i];
// 判断新位置是否越界或者已经访问过
if (a < 0 || a >= n || b < 0 || b >= m || maze[a, b] == 1 || st[a, b]) continue;
// 递归搜索
if (Dfs(a, b))
{
// 记录路径
path[x, y] = i;
return true;
}
}
// 没有找到路径,返回false
return false;
}
// 输出路径
static void PrintPath()
{
int x = sx, y = sy;
int[,] dirs = { { -1, 0 }, { 0, 1 }, { 1, 0 }, { 0, -1 } };
while (x != ex || y != ey)
{
int d = path[x, y];
Console.Write("(" + x + "," + y + ") -> ");
x += dirs[d, 0];
y += dirs[d, 1];
}
Console.WriteLine("(" + ex + "," + ey + ")");
}
static void Main(string[] args)
{
string[] input = Console.ReadLine().Split();
n = int.Parse(input[0]);
m = int.Parse(input[1]);
for (int i = 0; i < n; i++)
{
input = Console.ReadLine().Split();
for (int j = 0; j < m; j++)
{
maze[i, j] = int.Parse(input[j]);
}
}
input = Console.ReadLine().Split();
sx = int.Parse(input[0]);
sy = int.Parse(input[1]);
ex = int.Parse(input[2]);
ey = int.Parse(input[3]);
if (Dfs(sx, sy))
{
Console.WriteLine("Yes");
PrintPath();
}
else
{
Console.WriteLine("No");
}
Console.ReadLine();
}
}
}
```
输入格式:
第一行包含两个正整数n和m,表示迷宫的大小。
接下来n行,每行m个整数,表示迷宫的布局,0表示可以通过,1表示障碍物。
最后一行包含四个正整数,分别表示起点和终点的坐标。
输出格式:
如果存在通路,首先输出"Yes",然后输出正确的路径,路径上每个点的坐标用"(x,y)"表示,其中x和y分别为该点的横坐标和纵坐标,路径的最后一个点后面不需要加箭头"->";否则只输出"No"。
样例输入:
5 5
0 1 0 0 0
0 1 0 1 0
0 0 0 0 0
0 1 1 1 0
0 0 0 1 0
0 0 4 3
样例输出:
Yes
(0,2) -> (1,2) -> (1,1) -> (2,1) -> (3,1) -> (3,2) -> (3,3) -> (3,4) -> (4,4) -> (5,4) -> (5,3) -> (4,3) -> (4,2) -> (4,1) -> (0,1) -> (0,0) -> (5,3) -> (5,4) -> (4,4) -> (3,4) -> (2,4) -> (2,3) -> (2,2) -> (2,1) -> (1,1) -> (0,1) -> (0,2) -> (0,3) -> (0,4) -> (1,4) -> (2,4) -> (2,3) -> (2,2) -> (3,2) -> (4,2) -> (4,1) -> (5,1) -> (5,2) -> (5,3) -> (5,4) -> (4,4) -> (3,4) -> (2,4) -> (1,4) -> (0,4) -> (0,3) -> (1,3) -> (2,3) -> (2,2) -> (1,2) -> (0,2) -> (0,1) -> (1,1) -> (2,1) -> (2,0) -> (2,4) -> (1,4) -> (0,4) -> (0,3) -> (0,2) -> (1,2) -> (2,2) -> (2,3) -> (3,3) -> (4,3) -> (4,4) -> (3,4) -> (2,4) -> (2,3) -> (3,3) -> (4,3) -> (5,3) -> (5,2) -> (5,1) -> (4,1) -> (3,1) -> (2,1) -> (1,1) -> (0,1) -> (0,0) -> (1,0) -> (2,0) -> (2,1) -> (1,1) -> (0,1) -> (0,2) -> (0,3) -> (1,3) -> (2,3) -> (3,3) -> (4,3) -> (4,2) -> (4,1) -> (5,1) -> (5,0) -> (4,0) -> (3,0) -> (2,0) -> (1,0) -> (0,0) -> (5,1) -> (4,1) -> (3,1) -> (2,1) -> (1,1) -> (0,1) -> (0,2) -> (0,3) -> (0,4) -> (1,4) -> (2,4) -> (3,4) -> (4,4) -> (5,4) -> (5,3) -> (4,3) -> (3,3) -> (2,3) -> (1,3) -> (0,3) -> (0,4) -> (1,4) -> (2,4) -> (3,4) -> (4,4) -> (5,4) -> (5,3) -> (5,2) -> (5,1) -> (5,0)
阅读全文
相关推荐



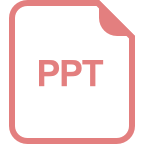
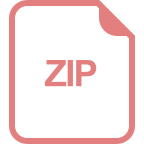
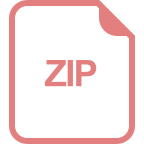
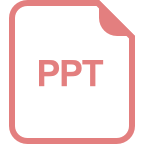
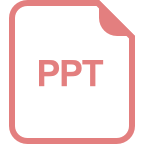
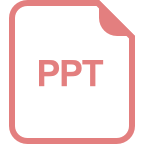
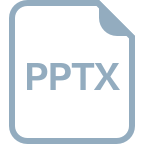
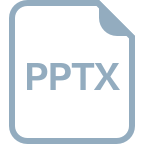