C语言中search的用法
时间: 2023-09-22 12:06:58 浏览: 175
C语言中的search函数用于在一个数组中查找指定元素。它的原型如下:
```
void *search(const void *key, const void *base, size_t nmemb, size_t size, int (*compar)(const void *, const void *));
```
参数解释:
- key:要查找的元素。
- base:要查找的数组。
- nmemb:数组中元素的个数。
- size:每个元素的大小。
- compar:用于比较元素的函数指针。
函数返回值:
- 如果找到了指定的元素,返回指向该元素的指针。
- 如果没有找到,返回NULL。
使用search函数的步骤如下:
1.编写比较函数,用于在数组中比较元素,返回值为0表示相等,返回值大于0表示第一个参数大于第二个参数,返回值小于0表示第一个参数小于第二个参数。
2.调用search函数,在参数中传入要查找的元素、数组、元素个数、元素大小和比较函数。
3.根据返回值判断是否找到了指定元素。
举例:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 比较函数
int cmp(const void *a, const void *b)
{
return strcmp((char *)a, (char *)b);
}
int main()
{
char *names[] = {"Alice", "Bob", "Charlie", "David", "Eva", "Frank", "Grace", "Helen"};
int len = sizeof(names) / sizeof(names[0]);
char *target = "David";
char **result = search(&target, names, len, sizeof(char *), cmp);
if (result == NULL) {
printf("Not found\n");
} else {
printf("Found: %s\n", *result);
}
return 0;
}
```
这段代码中,我们定义了一个字符串数组,然后使用search函数在其中查找指定的字符串。比较函数使用了标准库函数strcmp来比较字符串。最后根据返回值判断是否找到了指定字符串。
相关推荐
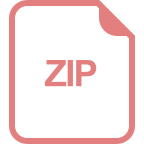
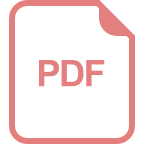
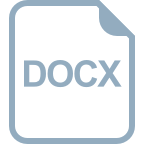
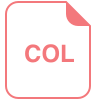
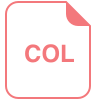
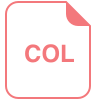
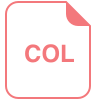
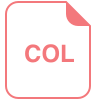









