All method binding in Java uses late binding unless the method is static or final (private methods are implicitly final).
时间: 2024-02-20 15:57:25 浏览: 114
Yes, that's correct. In Java, all non-static and non-final methods use late binding (also known as dynamic binding or run-time binding) by default. This means that the method implementation to be called is decided at runtime based on the actual type of the object that the method is being called on.
For example, consider the following code:
```
class Animal {
public void makeSound() {
System.out.println("Animal is making a sound");
}
}
class Cat extends Animal {
public void makeSound() {
System.out.println("Meow!");
}
}
class Dog extends Animal {
public void makeSound() {
System.out.println("Woof!");
}
}
public class Main {
public static void main(String[] args) {
Animal animal1 = new Cat();
Animal animal2 = new Dog();
animal1.makeSound(); // output: Meow!
animal2.makeSound(); // output: Woof!
}
}
```
In this example, we have a parent class `Animal` and two child classes `Cat` and `Dog`. Each class has its own implementation of the `makeSound()` method. In the `main()` method, we create two objects of type `Animal`, but their actual types are `Cat` and `Dog`, respectively. When we call the `makeSound()` method on these objects, the implementation that gets called is based on the actual type of the object, not the declared type of the variable.
However, if a method is declared as static or final, it uses early binding (also known as static binding or compile-time binding) instead of late binding. In the case of a static method, the method implementation to be called is determined at compile time based on the declared type of the variable. In the case of a final method, the method implementation is also determined at compile time, but cannot be overridden by any subclasses.
阅读全文
相关推荐
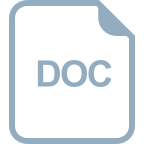




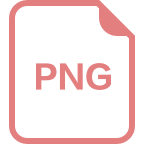


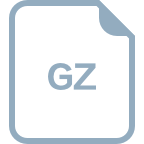


