用vue3以及elementplus写一个增删改查的页面,列表含有分页功能
时间: 2024-05-04 22:20:26 浏览: 192
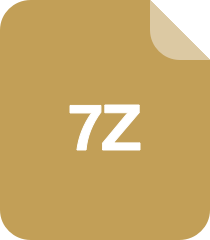
springboot+vue+elementui实现增删改查和分页查询

好的,下面是一个简单的示例代码,包含增删改查以及分页功能:
```html
<!-- 页面结构 -->
<template>
<div class="container">
<el-form :inline="true" class="search-form">
<el-form-item label="关键字">
<el-input v-model="searchKeyword" placeholder="请输入关键字"></el-input>
</el-form-item>
<el-form-item>
<el-button type="primary" @click="search">搜索</el-button>
</el-form-item>
</el-form>
<el-button class="add-btn" type="primary" @click="showAddDialog">添加数据</el-button>
<el-table :data="tableData" border stripe>
<el-table-column label="ID" prop="id"></el-table-column>
<el-table-column label="名称" prop="name"></el-table-column>
<el-table-column label="操作">
<template slot-scope="scope">
<el-button type="text" @click="showEditDialog(scope.row)">编辑</el-button>
<el-button type="text" @click="deleteItem(scope.row)">删除</el-button>
</template>
</el-table-column>
</el-table>
<el-pagination
@current-change="handleCurrentChange"
:current-page="currentPage"
:page-size="pageSize"
:total="total"
layout="total, sizes, prev, pager, next, jumper"
></el-pagination>
<el-dialog :visible.sync="isAddDialogVisible" title="添加数据">
<el-form ref="addForm" :model="addForm" :rules="addFormRules" label-width="80px">
<el-form-item label="名称" prop="name">
<el-input v-model="addForm.name"></el-input>
</el-form-item>
<el-form-item>
<el-button type="primary" @click="add">添加</el-button>
<el-button @click="resetAddForm">重置</el-button>
</el-form-item>
</el-form>
</el-dialog>
<el-dialog :visible.sync="isEditDialogVisible" title="编辑数据">
<el-form ref="editForm" :model="editForm" :rules="editFormRules" label-width="80px">
<el-form-item label="ID" prop="id">
<el-input v-model="editForm.id" disabled></el-input>
</el-form-item>
<el-form-item label="名称" prop="name">
<el-input v-model="editForm.name"></el-input>
</el-form-item>
<el-form-item>
<el-button type="primary" @click="edit">保存</el-button>
<el-button @click="resetEditForm">重置</el-button>
</el-form-item>
</el-form>
</el-dialog>
</div>
</template>
<script>
import { reactive, ref } from 'vue'
import { ElMessage, ElMessageBox } from 'element-plus'
// 模拟数据
const data = [
{ id: 1, name: '数据1' },
{ id: 2, name: '数据2' },
{ id: 3, name: '数据3' },
{ id: 4, name: '数据4' },
{ id: 5, name: '数据5' },
{ id: 6, name: '数据6' },
{ id: 7, name: '数据7' },
{ id: 8, name: '数据8' },
{ id: 9, name: '数据9' },
{ id: 10, name: '数据10' },
{ id: 11, name: '数据11' },
{ id: 12, name: '数据12' },
{ id: 13, name: '数据13' },
{ id: 14, name: '数据14' },
{ id: 15, name: '数据15' },
{ id: 16, name: '数据16' },
{ id: 17, name: '数据17' },
{ id: 18, name: '数据18' },
{ id: 19, name: '数据19' },
{ id: 20, name: '数据20' },
{ id: 21, name: '数据21' },
{ id: 22, name: '数据22' },
{ id: 23, name: '数据23' },
{ id: 24, name: '数据24' },
{ id: 25, name: '数据25' },
]
export default {
setup() {
// 响应式数据
const searchKeyword = ref('')
const tableData = reactive([])
const currentPage = ref(1)
const pageSize = ref(5)
const total = ref(0)
const isAddDialogVisible = ref(false)
const isEditDialogVisible = ref(false)
const addForm = reactive({ name: '' })
const editForm = reactive({ id: '', name: '' })
// 表单验证规则
const addFormRules = {
name: [{ required: true, message: '请输入名称', trigger: 'blur' }],
}
const editFormRules = {
name: [{ required: true, message: '请输入名称', trigger: 'blur' }],
}
// 获取数据
const fetchData = () => {
// 模拟异步获取数据
setTimeout(() => {
const offset = (currentPage.value - 1) * pageSize.value
const filteredData = searchKeyword.value
? data.filter(item => item.name.includes(searchKeyword.value))
: data
tableData.splice(0, tableData.length, ...filteredData.slice(offset, offset + pageSize.value))
total.value = filteredData.length
}, 500)
}
// 搜索
const search = () => {
currentPage.value = 1
fetchData()
}
// 切换页码
const handleCurrentChange = (newPage) => {
currentPage.value = newPage
fetchData()
}
// 显示添加对话框
const showAddDialog = () => {
isAddDialogVisible.value = true
}
// 添加数据
const add = () => {
// 表单验证
const valid = ref(true)
const addFormRef = ref(null)
addFormRef.value.validate(valid => {
if (valid) {
// 模拟异步添加数据
setTimeout(() => {
const newId = data[data.length - 1].id + 1
data.push({ id: newId, name: addForm.name })
fetchData()
isAddDialogVisible.value = false
addFormRef.value.resetFields()
ElMessage.success('添加成功')
}, 500)
}
})
}
// 重置添加表单
const resetAddForm = () => {
const addFormRef = ref(null)
addFormRef.value.resetFields()
}
// 显示编辑对话框
const showEditDialog = (row) => {
editForm.id = row.id
editForm.name = row.name
isEditDialogVisible.value = true
}
// 编辑数据
const edit = () => {
// 表单验证
const valid = ref(true)
const editFormRef = ref(null)
editFormRef.value.validate(valid => {
if (valid) {
// 模拟异步编辑数据
setTimeout(() => {
const index = data.findIndex(item => item.id === editForm.id)
data[index].name = editForm.name
fetchData()
isEditDialogVisible.value = false
editFormRef.value.resetFields()
ElMessage.success('编辑成功')
}, 500)
}
})
}
// 重置编辑表单
const resetEditForm = () => {
const editFormRef = ref(null)
editFormRef.value.resetFields()
}
// 删除数据
const deleteItem = (row) => {
ElMessageBox.confirm('确认删除该数据吗?', '提示', {
confirmButtonText: '确定',
cancelButtonText: '取消',
type: 'warning'
}).then(() => {
// 模拟异步删除数据
setTimeout(() => {
const index = data.findIndex(item => item.id === row.id)
data.splice(index, 1)
fetchData()
ElMessage.success('删除成功')
}, 500)
}).catch(() => {})
}
// 初始化数据
fetchData()
return {
searchKeyword,
tableData,
currentPage,
pageSize,
total,
isAddDialogVisible,
isEditDialogVisible,
addForm,
editForm,
addFormRules,
editFormRules,
search,
handleCurrentChange,
showAddDialog,
add,
resetAddForm,
showEditDialog,
edit,
resetEditForm,
deleteItem,
}
}
}
</script>
<style scoped>
.container {
margin: 20px;
}
.search-form {
margin-bottom: 20px;
}
.add-btn {
margin-bottom: 20px;
}
</style>
```
在这个示例代码中,我们使用了 Vue 3 的 Composition API 来实现页面逻辑,并使用了 Element Plus UI 组件库来实现页面 UI。具体实现细节请见代码注释。
需要注意的是,在这个示例代码中,我们使用了一个模拟数据 `data` 来模拟从后端获取数据的过程。在实际开发中,我们需要根据具体的业务需求,使用合适的方式来获取后端数据,并进行相应的处理。
阅读全文
相关推荐
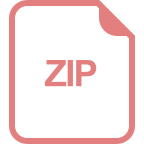
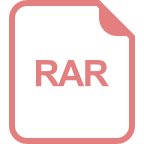
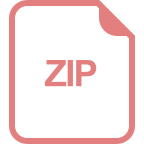
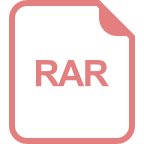
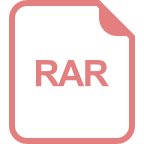
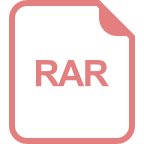
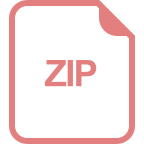
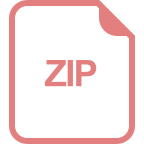
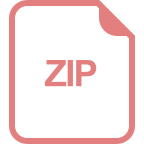
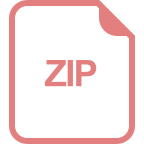
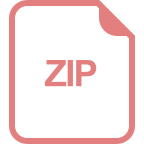
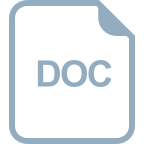
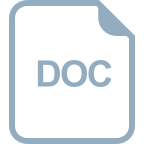
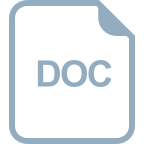
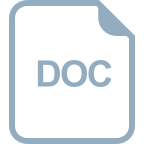
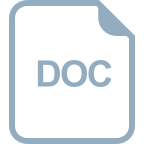
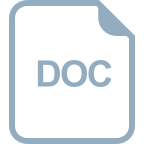