uniapp 登录代码
时间: 2023-09-11 07:05:22 浏览: 45
### 回答1:
以下是 uni-app 实现登录功能的示例代码:
```
<template>
<view class="container">
<view class="form-group">
<label for="username">用户名</label>
<input type="text" id="username" v-model="username" />
</view>
<view class="form-group">
<label for="password">密码</label>
<input type="password" id="password" v-model="password" />
</view>
<view class="form-group">
<button @click="login">登录</button>
</view>
</view>
</template>
<script>
export default {
data() {
return {
username: '',
password: '',
};
},
methods: {
async login() {
// 假设已经通过 axios 发送请求到后台登录接口
const res = await this.$axios.post('/login', {
username: this.username,
password: this.password,
});
if (res.data.code === 200) {
// 登录成功,可以做一些其他操作,比如存储 token
this.$store.commit('setToken', res.data.token);
this.$uni.showToast({
title: '登录成功',
});
this.$uni.navigateBack();
} else {
this.$uni.showToast({
title: '登录失败,请检查用户名和密码',
icon: 'none',
});
}
},
},
};
</script>
```
请注意,这只是一个示例代码,在实际项目中可能需要根据需求进行调整。
### 回答2:
uniapp作为一款跨平台的开发框架,可以使用vue进行开发,在实现登录功能的代码中,需要涉及到前端和后端的交互。下面是一个简单的登录代码示例。
前端代码:
```vue
<template>
<div>
<input v-model="username" placeholder="请输入用户名" />
<input v-model="password" type="password" placeholder="请输入密码" />
<button @click="login">登录</button>
</div>
</template>
<script>
export default {
data() {
return {
username: '',
password: ''
};
},
methods: {
login() {
// 发送登录请求到后端
uni.request({
url: 'http://example.com/login', // 后端登录接口地址
method: 'POST',
data: {
username: this.username,
password: this.password
},
success: (res) => {
// 登录成功,跳转到首页
uni.navigateTo({
url: '/pages/home/home'
});
},
fail: (res) => {
// 登录失败,处理错误信息
console.error(res);
}
});
}
}
};
</script>
```
后端接口代码示例(示例使用Node.js和Express框架):
```javascript
const express = require('express');
const app = express();
// 登录接口
app.post('/login', (req, res) => {
const username = req.body.username;
const password = req.body.password;
// 根据用户名和密码进行登录验证
// 如果验证通过,可以将用户信息存储在session或者生成token返回给前端
if (username === 'admin' && password === '123456') {
res.status(200).json({
message: '登录成功'
});
} else {
res.status(401).json({
message: '登录失败,用户名或密码错误'
});
}
});
app.listen(3000, () => {
console.log('后端服务启动成功');
});
```
通过以上代码示例,前端通过uni.request发送登录请求到后端的/login接口,后端根据用户名和密码进行验证,返回相应的登录结果。登录成功时,前端使用uni.navigateTo跳转到首页;登录失败时,前端可以处理错误信息。需要注意的是,以上示例仅供参考,实际项目中可能会有更多的验证和安全措施。
相关推荐
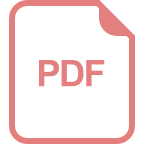
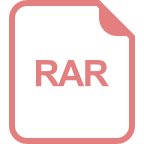














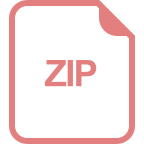