新标准c++程序设计第8章文件操作
时间: 2023-06-20 15:06:25 浏览: 119
第8章主要介绍了C++中的文件操作,包括文件的打开、读写、关闭等基本操作。下面是一些重点内容:
1. 文件的打开
可以使用fstream库中的ifstream、ofstream、fstream三个类分别表示输入文件流、输出文件流、输入输出文件流。它们的构造函数可以传入文件名和打开模式,例如:
```c++
#include <fstream>
using namespace std;
int main() {
// 打开一个文本文件用于输入
ifstream input("input.txt");
if (!input) {
cout << "打开文件失败!" << endl;
return 1;
}
// 打开一个文本文件用于输出
ofstream output("output.txt");
if (!output) {
cout << "打开文件失败!" << endl;
return 1;
}
// 打开一个文本文件用于输入输出
fstream io("data.txt", ios::in | ios::out);
if (!io) {
cout << "打开文件失败!" << endl;
return 1;
}
// ...
}
```
2. 文件的读写
使用文件流对象的<<、>>、getline等操作符进行读写。例如:
```c++
#include <fstream>
#include <iostream>
using namespace std;
int main() {
// 打开一个文本文件用于输入
ifstream input("input.txt");
if (!input) {
cout << "打开文件失败!" << endl;
return 1;
}
// 打开一个文本文件用于输出
ofstream output("output.txt");
if (!output) {
cout << "打开文件失败!" << endl;
return 1;
}
int x;
input >> x; // 从文件中读取一个整数
output << "x = " << x << endl; // 将x输出到文件中
string line;
getline(input, line); // 从文件中读取一行字符串
output << line << endl; // 将字符串输出到文件中
// ...
}
```
3. 文件的关闭
使用文件流对象的close()方法来关闭文件。例如:
```c++
#include <fstream>
using namespace std;
int main() {
// 打开一个文本文件用于输入
ifstream input("input.txt");
if (!input) {
cout << "打开文件失败!" << endl;
return 1;
}
// 关闭文件
input.close();
}
```
以上是新标准C++程序设计第8章文件操作的一些重点内容,希望能对你有所帮助。
阅读全文
相关推荐
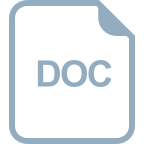
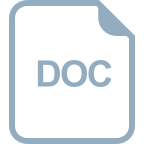
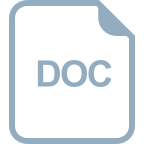
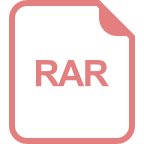
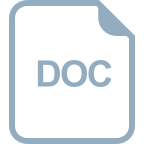
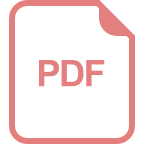
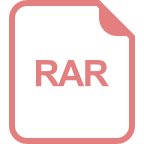
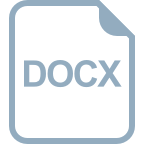
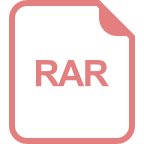
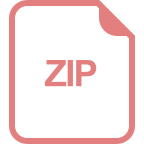
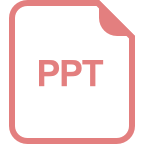
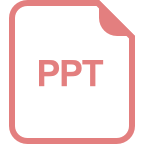
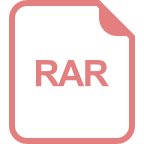
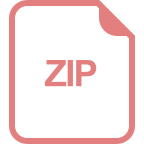
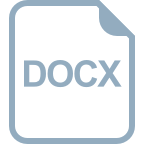
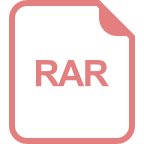
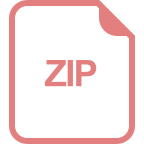
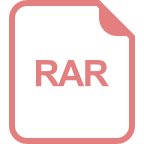