Python 代码示例,实现了一个包含文档管理、搜索引擎和统计报表的电子文档管理系统:
时间: 2024-03-24 08:38:11 浏览: 65
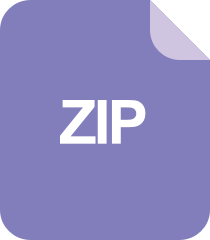
基于python开发的一套内容管理系统+源代码+文档说明
以下是一个简单的Python代码示例,实现了一个包含文档管理、搜索引擎和统计报表的电子文档管理系统。
```python
import os
import re
import collections
# 定义文档类
class Document:
def __init__(self, name, content):
self.name = name
self.content = content
# 定义文档管理类
class DocumentManager:
def __init__(self):
self.documents = []
# 添加文档
def add_document(self, document):
self.documents.append(document)
# 删除文档
def remove_document(self, document):
self.documents.remove(document)
# 获取所有文档名称
def get_all_document_names(self):
return [doc.name for doc in self.documents]
# 定义搜索引擎类
class SearchEngine:
# 实现简单的单词分割和去重
@staticmethod
def split_words(text):
return list(set(re.findall(r'\w+', text.lower())))
# 根据关键词搜索文档
@classmethod
def search_documents(cls, documents, keyword):
results = []
for doc in documents:
if keyword in cls.split_words(doc.content):
results.append(doc)
return results
# 定义统计报表类
class ReportGenerator:
# 统计所有文档中单词出现频率的前N项
@staticmethod
def get_top_n_words(documents, n=10):
word_counter = collections.Counter()
for doc in documents:
words = SearchEngine.split_words(doc.content)
word_counter.update(words)
return word_counter.most_common(n)
# 测试代码
if __name__ == '__main__':
# 创建文档管理器
doc_manager = DocumentManager()
# 添加文档
doc_manager.add_document(Document('Document 1', 'Python is a popular programming language.'))
doc_manager.add_document(Document('Document 2', 'Python is easy to learn and use.'))
doc_manager.add_document(Document('Document 3', 'Python is used for web development and data analysis.'))
# 搜索文档
search_results = SearchEngine.search_documents(doc_manager.documents, 'python')
for result in search_results:
print(result.name)
# 统计词频
top_words = ReportGenerator.get_top_n_words(doc_manager.documents)
for word in top_words:
print(word[0], word[1])
```
该代码示例中,我们定义了三个类:`Document`、`DocumentManager`和`SearchEngine`以及一个静态方法的类`ReportGenerator`。其中,`Document`类表示一个文档,包含名称和内容两个属性;`DocumentManager`类实现对文档的添加、删除和获取所有文档名称等操作;`SearchEngine`类实现了一个简单的单词分割和去重算法,并提供了根据关键词搜索文档的方法;`ReportGenerator`类实现了一个统计所有文档中单词出现频率的前N项的方法。
在测试代码中,我们创建了一个文档管理器,并添加了三个文档。然后,我们使用搜索引擎搜索包含关键词“python”的文档,并输出结果。最后,我们使用统计报表类统计了所有文档中单词出现频率的前N项,并输出结果。
阅读全文
相关推荐
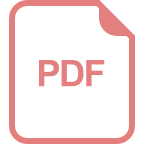
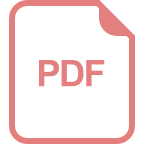

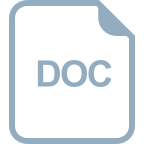
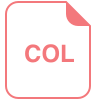
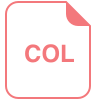
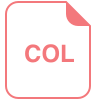
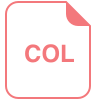
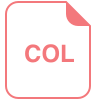
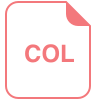
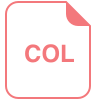
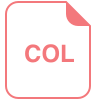
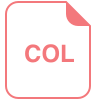
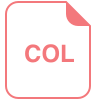
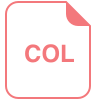
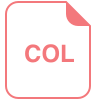
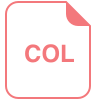
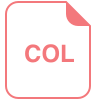