使用tkinter制作一个简单的计算器,能完成浮点数的加减乘除计算。
时间: 2023-12-06 22:45:05 浏览: 64
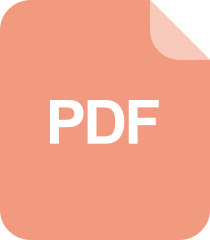
python使用tkinter实现简单计算器
好的,以下是一个简单的基于tkinter的计算器代码:
```python
import tkinter as tk
class Calculator(tk.Frame):
def __init__(self, master=None):
super().__init__(master)
self.grid()
self.create_widgets()
self.result = 0
self.current = ""
self.operator = ""
def create_widgets(self):
self.display = tk.Entry(self, width=20, font=("Arial", 16))
self.display.grid(row=0, column=0, columnspan=4, pady=5)
self.button_1 = tk.Button(self, text="1", width=5, font=("Arial", 16), command=lambda: self.add_number("1"))
self.button_1.grid(row=1, column=0, pady=5)
self.button_2 = tk.Button(self, text="2", width=5, font=("Arial", 16), command=lambda: self.add_number("2"))
self.button_2.grid(row=1, column=1, pady=5)
self.button_3 = tk.Button(self, text="3", width=5, font=("Arial", 16), command=lambda: self.add_number("3"))
self.button_3.grid(row=1, column=2, pady=5)
self.button_4 = tk.Button(self, text="4", width=5, font=("Arial", 16), command=lambda: self.add_number("4"))
self.button_4.grid(row=2, column=0, pady=5)
self.button_5 = tk.Button(self, text="5", width=5, font=("Arial", 16), command=lambda: self.add_number("5"))
self.button_5.grid(row=2, column=1, pady=5)
self.button_6 = tk.Button(self, text="6", width=5, font=("Arial", 16), command=lambda: self.add_number("6"))
self.button_6.grid(row=2, column=2, pady=5)
self.button_7 = tk.Button(self, text="7", width=5, font=("Arial", 16), command=lambda: self.add_number("7"))
self.button_7.grid(row=3, column=0, pady=5)
self.button_8 = tk.Button(self, text="8", width=5, font=("Arial", 16), command=lambda: self.add_number("8"))
self.button_8.grid(row=3, column=1, pady=5)
self.button_9 = tk.Button(self, text="9", width=5, font=("Arial", 16), command=lambda: self.add_number("9"))
self.button_9.grid(row=3, column=2, pady=5)
self.button_0 = tk.Button(self, text="0", width=5, font=("Arial", 16), command=lambda: self.add_number("0"))
self.button_0.grid(row=4, column=1, pady=5)
self.button_plus = tk.Button(self, text="+", width=5, font=("Arial", 16), command=lambda: self.add_operator("+"))
self.button_plus.grid(row=1, column=3, pady=5)
self.button_minus = tk.Button(self, text="-", width=5, font=("Arial", 16), command=lambda: self.add_operator("-"))
self.button_minus.grid(row=2, column=3, pady=5)
self.button_multiply = tk.Button(self, text="*", width=5, font=("Arial", 16), command=lambda: self.add_operator("*"))
self.button_multiply.grid(row=3, column=3, pady=5)
self.button_divide = tk.Button(self, text="/", width=5, font=("Arial", 16), command=lambda: self.add_operator("/"))
self.button_divide.grid(row=4, column=3, pady=5)
self.button_clear = tk.Button(self, text="C", width=5, font=("Arial", 16), command=self.clear)
self.button_clear.grid(row=4, column=0, pady=5)
self.button_dot = tk.Button(self, text=".", width=5, font=("Arial", 16), command=lambda: self.add_number("."))
self.button_dot.grid(row=4, column=2, pady=5)
self.button_equal = tk.Button(self, text="=", width=5, font=("Arial", 16), command=self.calculate)
self.button_equal.grid(row=5, column=0, columnspan=4, pady=5)
def add_number(self, number):
self.current += str(number)
self.display.delete(0, tk.END)
self.display.insert(0, self.current)
def add_operator(self, operator):
self.operator = operator
self.result = float(self.current)
self.current = ""
def clear(self):
self.result = 0
self.current = ""
self.operator = ""
self.display.delete(0, tk.END)
def calculate(self):
if self.operator == "+":
self.result += float(self.current)
elif self.operator == "-":
self.result -= float(self.current)
elif self.operator == "*":
self.result *= float(self.current)
elif self.operator == "/":
self.result /= float(self.current)
self.display.delete(0, tk.END)
self.display.insert(0, self.result)
self.current = ""
root = tk.Tk()
calculator = Calculator(root)
calculator.mainloop()
```
这个计算器使用grid布局,包含数字、运算符、小数点和清除键。`add_number()` 方法将数字添加到当前输入中,`add_operator()` 方法将运算符记录下来并计算当前结果,`calculate()` 方法根据当前运算符计算结果。`clear()` 方法清除所有输入并重置结果和运算符。
阅读全文
相关推荐



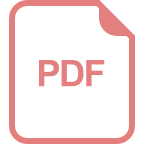
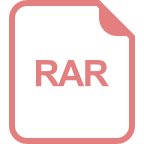
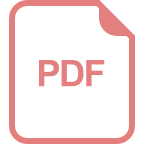
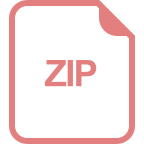
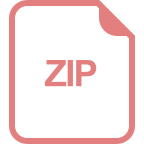
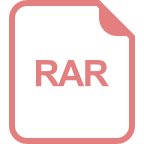
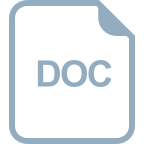
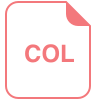


