#include <stdio.h> #include <string.h> #define MAX_STUDENT_NUM 30 // #define MAX_COURSE_NUM 6 // #define NAME_LEN 20 // struct Student { char id[10];//学生学号 char name[NAME_LEN];//姓名 int scores[MAX_COURSE_NUM];//各科分数 int total_score;//总分 float avg_score;//平均分 }; void statistic_analysis(struct Student students[], int num, int course_num);//对每门课程分别统计每个类别的人数以及所占的百分比 void list_record(struct Student students[], int num, int course_num);//输出每个学生的学号、姓名、各科考试成绩以及每门课程的总分和平均分 请用c语言分别补充函数内容
时间: 2023-10-22 22:06:28 浏览: 47
statistic_analysis函数内容如下:
```
void statistic_analysis(struct Student students[], int num, int course_num) {
int i, j;
int count[MAX_COURSE_NUM][3] = {0}; // 统计每门课程的A、B、C类人数
float percent[MAX_COURSE_NUM][3] = {0}; // 统计每门课程的A、B、C类人数所占百分比
// 遍历每个学生
for (i = 0; i < num; i++) {
// 遍历每门课程
for (j = 0; j < course_num; j++) {
if (students[i].scores[j] >= 90) {
count[j][0]++; // A类人数+1
} else if (students[i].scores[j] >= 80) {
count[j][1]++; // B类人数+1
} else if (students[i].scores[j] >= 70) {
count[j][2]++; // C类人数+1
}
}
}
// 计算每门课程的A、B、C类人数所占百分比
for (i = 0; i < course_num; i++) {
for (j = 0; j < 3; j++) {
percent[i][j] = (float) count[i][j] / num * 100;
}
}
// 输出统计结果
printf("Course\tA\tB\tC\n");
for (i = 0; i < course_num; i++) {
printf("%d\t%.2f%%\t%.2f%%\t%.2f%%\n", i+1, percent[i][0], percent[i][1], percent[i][2]);
}
}
```
list_record函数内容如下:
```
void list_record(struct Student students[], int num, int course_num) {
int i, j;
printf("ID\tName\t");
for (i = 0; i < course_num; i++) {
printf("Course%d\t", i+1);
}
printf("Total\tAverage\n");
// 输出每个学生的信息
for (i = 0; i < num; i++) {
printf("%s\t%s\t", students[i].id, students[i].name);
int total_score = 0;
for (j = 0; j < course_num; j++) {
printf("%d\t", students[i].scores[j]);
total_score += students[i].scores[j];
}
students[i].total_score = total_score;
students[i].avg_score = (float) total_score / course_num;
printf("%d\t%.2f\n", students[i].total_score, students[i].avg_score);
}
}
```
相关推荐
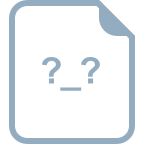
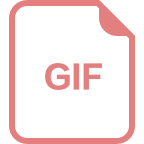
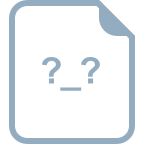
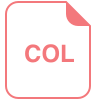
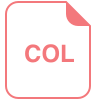









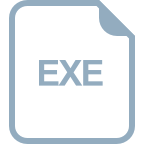
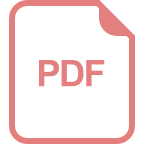
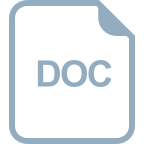
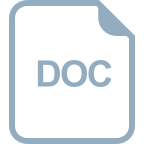
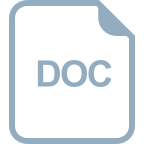
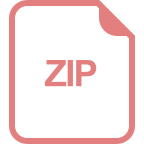