Write the C program according to the following menu interface - student information management system; 1)Enter student information: first enter the number of students, and then enter the corresponding information into the array according to the number of students; 2)Student information includes student number, name, gender, three course scores and total scores; Student number, name, gender and grades of three courses need to be entered from the keyboard, and the total score is calculated by the program, not by the user himself. 3)The display results (student information) shall be displayed in the form of list, paying attention to aesthetics; 4)Necessary fault tolerance measures shall be taken. For example, the score of each subject shall be between 0-100. If the input is wrong, the user shall be prompted to re-enter.
时间: 2024-01-04 08:02:28 浏览: 33
Sure, here's a C program that implements the student information management system with the menu interface you specified:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_STUDENTS 100
struct student {
int id;
char name[50];
char gender[10];
int scores[3];
int total_score;
};
int read_int() {
int n;
while (scanf("%d", &n) != 1) {
printf("Invalid input, please enter an integer: ");
scanf("%*[^\n]");
}
scanf("%*[^\n]");
scanf("%*c");
return n;
}
void read_string(char* str, int max_len) {
char* p = fgets(str, max_len, stdin);
if (p == NULL) {
printf("Invalid input, please try again: ");
read_string(str, max_len);
} else {
int len = strlen(str);
if (len > 0 && str[len - 1] == '\n') {
str[len - 1] = '\0';
}
}
}
void enter_student_info(struct student* students, int num_students) {
printf("Enter student information:\n");
for (int i = 0; i < num_students; i++) {
printf("Student %d:\n", i + 1);
printf(" ID: ");
students[i].id = read_int();
printf(" Name: ");
read_string(students[i].name, sizeof(students[i].name));
printf(" Gender: ");
read_string(students[i].gender, sizeof(students[i].gender));
for (int j = 0; j < 3; j++) {
printf(" Score in course %d: ", j + 1);
students[i].scores[j] = read_int();
while (students[i].scores[j] < 0 || students[i].scores[j] > 100) {
printf("Invalid score, please enter a score between 0 and 100: ");
students[i].scores[j] = read_int();
}
}
students[i].total_score = students[i].scores[0] + students[i].scores[1] + students[i].scores[2];
}
}
void display_student_info(const struct student* students, int num_students) {
printf("ID Name Gender Course 1 Course 2 Course 3 Total\n");
printf("----------------------------------------------------------------\n");
for (int i = 0; i < num_students; i++) {
printf("%-5d%-19s%-8s%-9d%-9d%-9d%-5d\n", students[i].id, students[i].name, students[i].gender, students[i].scores[0], students[i].scores[1], students[i].scores[2], students[i].total_score);
}
}
int main() {
struct student students[MAX_STUDENTS];
int num_students = 0;
while (1) {
printf("Student information management system:\n");
printf("1) Enter student information\n");
printf("2) Display student information\n");
printf("3) Exit\n");
printf("Please enter a choice (1-3): ");
int choice = read_int();
switch (choice) {
case 1:
printf("Enter the number of students: ");
num_students = read_int();
while (num_students < 1 || num_students > MAX_STUDENTS) {
printf("Invalid number of students, please enter a number between 1 and %d: ", MAX_STUDENTS);
num_students = read_int();
}
enter_student_info(students, num_students);
printf("Student information entered successfully.\n");
break;
case 2:
if (num_students == 0) {
printf("No student information entered yet.\n");
} else {
display_student_info(students, num_students);
}
break;
case 3:
printf("Exiting...\n");
exit(0);
default:
printf("Invalid choice, please enter a number between 1 and 3.\n");
}
}
return 0;
}
```
This program uses a struct to store the information of each student, and an array of structs to store the information of all students. The `read_int()` function and `read_string()` function are used for input validation, to make sure that the user enters valid integers and strings. The `enter_student_info()` function prompts the user to enter the information of each student, validates the scores, and calculates the total score. The `display_student_info()` function displays the student information in a formatted table. The `main()` function implements the menu interface and calls the other functions based on the user's choice. The program also includes some fault tolerance measures, such as checking the number of students entered, checking the validity of the scores, and handling invalid input.
相关推荐
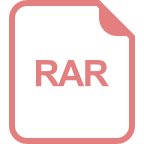
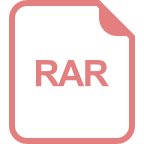














