write a c++ program to calculate the GPA of each students input in the total average score
时间: 2024-10-10 11:16:44 浏览: 17
在C++中编写一个程序来计算每个学生输入的总平均分数及GPA,首先需要了解GPA通常是基于课程成绩点数(Grade Point Average)来计算的,通常美国系统使用4.0分制。下面是一个简单的示例,它会提示用户输入学生成绩及其对应学分,并计算GPA:
```cpp
#include <iostream>
using namespace std;
// 定义一个结构体表示一门课程
struct Course {
string name;
double score; // 成绩
int credit_hours; // 学分小时
};
// 计算单门课程的GPA
double calculate_gpa(double score, int credit_hours) {
return (score * credit_hours) / 4.0;
}
int main() {
const int MAX_STUDENTS = 5;
Course students[MAX_STUDENTS];
for (int i = 0; i < MAX_STUDENTS; ++i) {
cout << "请输入第" << i+1 << "位学生的课程信息(课程名、成绩和学分小时,按空格分隔):" << endl;
cin >> students[i].name >> students[i].score >> students[i].credit_hours;
// 计算并打印GPA
double gpa = calculate_gpa(students[i].score, students[i].credit_hours);
cout << "学生 " << students[i].name << " 的课程 " << students[i].name << " 的GPA是: " << gpa << endl;
}
// 计算所有课程的总平均分
double total_score = 0;
double total_credits = 0;
for (const auto& student : students) {
total_score += student.score * student.credit_hours;
total_credits += student.credit_hours;
}
if (total_credits != 0) {
double overall_gpa = total_score / total_credits;
cout << "所有课程的总平均GPA是: " << overall_gpa << endl;
} else {
cout << "没有输入任何课程,无法计算总GPA。\n";
}
return 0;
}
```
相关推荐
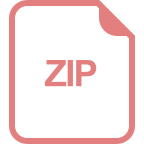
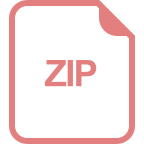
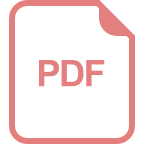















