c语言输出两句话中最长的公共单词
时间: 2024-04-10 14:28:16 浏览: 19
你好!对于你的问题,你可以使用以下步骤来找到两句话中最长的公共单词:
1. 将两句话分别存储在两个字符串变量中。
2. 使用 strtok 函数将第一句话分割成单词,并将每个单词与第二句话逐个比较。
3. 如果找到一个相同的单词,检查它的长度是否大于当前记录的最长公共单词长度,如果是,则更新最长公共单词和最长长度。
4. 重复步骤 2 和 3,直到第一句话中的所有单词都被比较完毕。
5. 输出最长公共单词。
下面是一个示例代码,演示了如何实现这个功能:
```c
#include <stdio.h>
#include <string.h>
int main() {
char sentence1[] = "Hello world, this is a sentence.";
char sentence2[] = "This is another sentence with some words.";
char* word1 = strtok(sentence1, " .,");
char* longestWord = "";
int longestLength = 0;
while (word1 != NULL) {
char* word2 = strtok(sentence2, " .,");
while (word2 != NULL) {
if (strcmp(word1, word2) == 0) {
int length = strlen(word1);
if (length > longestLength) {
longestWord = word1;
longestLength = length;
}
}
word2 = strtok(NULL, " .,");
}
word1 = strtok(NULL, " .,");
}
printf("最长的公共单词是: %s\n", longestWord);
return 0;
}
```
这段代码会输出最长的公共单词。请注意,这里的实现假设单词之间以空格、句点和逗号作为分隔符。你可以根据实际情况进行适当的修改和调整。希望能对你有所帮助!如果还有其他问题,请随时提问。
相关推荐
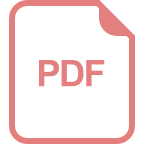
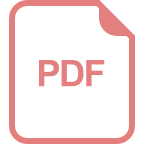
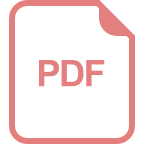














