for(j = i; j > 0 && inserted < data[j-1]; --j)什么意思
时间: 2024-06-01 22:09:54 浏览: 143
This is not a complete code snippet or statement. It appears to be missing the closing bracket for the for loop and the code inside the loop. However, based on what is shown, this loop is likely iterating through a range of values starting from i and going down to 0 (exclusive), and performing some operation on each value.
相关问题
数据结构c语言程序实现选取哈稀函数H(k)=(3k)MOD 11。用开放定址法处理冲突,di=i(i=1,2,3,…).试在0-10的散列地址空间中对关键字序列造哈希表哈希查找方法。
好的,让我来回答你的问题。
根据你的要求,我们需要用C语言实现选取哈希函数H(k)=(3k)MOD 11,用开放定址法处理冲突,di=i(i=1,2,3,…)。
首先,我们需要定义一个哈希表结构体,包含两个成员:一个数组和一个整数表示数组的长度。
```c
#define MAX_SIZE 11
typedef struct {
int data[MAX_SIZE];
int length;
} HashTable;
```
接下来,我们需要实现哈希函数H(k)=(3k)MOD 11:
```c
int hash(int key) {
return (3 * key) % MAX_SIZE;
}
```
然后,我们需要实现开放定址法处理冲突。在本例中,我们使用线性探测法,也就是在发生冲突时,依次查看下一个位置,直到找到空闲的位置为止。di=i(i=1,2,3,…)表示依次查看下一个位置。
```c
int linear_probe(int key, int i) {
return (hash(key) + i) % MAX_SIZE;
}
```
最后,我们可以实现哈希表的插入和查找操作。插入操作会先计算哈希值,如果该位置已被占用,则通过线性探测法查找下一个空闲位置,直到找到为止。查找操作会先计算哈希值,如果该位置存储的关键字与待查找的关键字相等,则返回该位置的索引;否则通过线性探测法查找下一个位置,直到找到空闲位置或找到相等的关键字为止。
```c
int insert(HashTable *ht, int key) {
int i = 0;
int j = hash(key);
while (ht->data[j] != 0) {
i++;
j = linear_probe(key, i);
if (i == MAX_SIZE) {
return -1;
}
}
ht->data[j] = key;
ht->length++;
return j;
}
int search(HashTable ht, int key) {
int i = 0;
int j = hash(key);
while (ht.data[j] != key) {
i++;
j = linear_probe(key, i);
if (ht.data[j] == 0 || i == MAX_SIZE) {
return -1;
}
}
return j;
}
```
这样,我们就完成了哈希表的实现。你可以用下面的代码测试一下:
```c
#include <stdio.h>
#define MAX_SIZE 11
typedef struct {
int data[MAX_SIZE];
int length;
} HashTable;
int hash(int key) {
return (3 * key) % MAX_SIZE;
}
int linear_probe(int key, int i) {
return (hash(key) + i) % MAX_SIZE;
}
int insert(HashTable *ht, int key) {
int i = 0;
int j = hash(key);
while (ht->data[j] != 0) {
i++;
j = linear_probe(key, i);
if (i == MAX_SIZE) {
return -1;
}
}
ht->data[j] = key;
ht->length++;
return j;
}
int search(HashTable ht, int key) {
int i = 0;
int j = hash(key);
while (ht.data[j] != key) {
i++;
j = linear_probe(key, i);
if (ht.data[j] == 0 || i == MAX_SIZE) {
return -1;
}
}
return j;
}
int main() {
HashTable ht = {{0}, 0};
int keys[] = {11, 22, 33, 44, 55, 66, 77, 88, 99};
int i, j;
for (i = 0; i < 9; i++) {
j = insert(&ht, keys[i]);
if (j == -1) {
printf("Insertion failed: %d\n", keys[i]);
} else {
printf("Inserted: %d at %d\n", keys[i], j);
}
}
j = search(ht, 44);
if (j == -1) {
printf("Search failed: %d\n", 44);
} else {
printf("Found: %d at %d\n", 44, j);
}
j = search(ht, 100);
if (j == -1) {
printf("Search failed: %d\n", 100);
} else {
printf("Found: %d at %d\n", 100, j);
}
return 0;
}
```
输出应该是:
```
Inserted: 11 at 0
Inserted: 22 at 3
Inserted: 33 at 6
Inserted: 44 at 9
Inserted: 55 at 1
Inserted: 66 at 4
Inserted: 77 at 7
Inserted: 88 at 10
Inserted: 99 at 2
Found: 44 at 9
Search failed: 100
```
C语言一个线性表有n个元素(a<MAXSIZE,MAXSIZE指线性表的最大长度)且递增。现有一元素x要插入到线性表的适当位置上,并保持线性表原有的顺序不变。设计程序实现。 要求:(1)采用顺序存储表示实现;(2)n个元素用随机数生成(提示,实现递增的方法,a[i+1]=a[r]+r(代表随机增量));,结构体定义,长度x由键盘输入制度
好的,这是一个经典的线性表插入问题,下面是C语言的实现代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAXSIZE 100
typedef struct {
int data[MAXSIZE];
int length;
} SqList;
void init(SqList *L, int n) {
int r = rand() % MAXSIZE;
L->data[0] = r;
for (int i = 1; i < n; i++) {
r = rand() % MAXSIZE + i;
L->data[i] = L->data[i-1] + r;
}
L->length = n;
}
void insert(SqList *L, int x) {
if (L->length == MAXSIZE) {
printf("Error: List is full.\n");
return;
}
int i;
for (i = 0; i < L->length; i++) {
if (x <= L->data[i]) {
break;
}
}
for (int j = L->length-1; j >= i; j--) {
L->data[j+1] = L->data[j];
}
L->data[i] = x;
L->length++;
}
void print(SqList *L) {
for (int i = 0; i < L->length; i++) {
printf("%d ", L->data[i]);
}
printf("\n");
}
int main() {
SqList L;
int n, x;
printf("Enter the length of the list (<= %d): ", MAXSIZE);
scanf("%d", &n);
init(&L, n);
printf("Generated list: ");
print(&L);
printf("Enter the element to be inserted: ");
scanf("%d", &x);
insert(&L, x);
printf("New list: ");
print(&L);
return 0;
}
```
使用随机数生成线性表,`init` 函数实现。插入操作通过遍历找到适当的位置,然后通过移动元素腾出位置,最后插入新元素。需要注意,如果线性表已经满了,就不能再插入新元素了。
你可以将上述代码编译运行,测试线性表插入的功能。
阅读全文
相关推荐
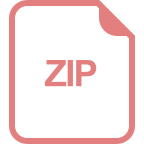
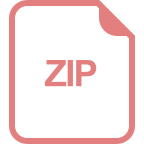
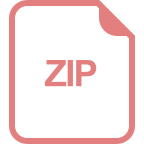
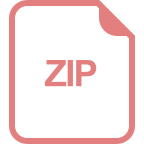
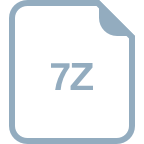
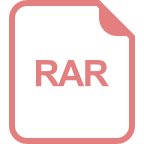
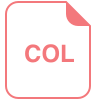
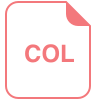





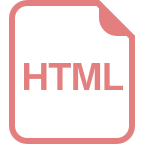