c语言随机生成10个不同的数字
时间: 2023-06-03 19:03:12 浏览: 191
好的,我可以帮您生成随机数。以下是生成10个不同数字的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
int main()
{
int nums[10];
int i, j, temp;
srand(time(NULL));
for(i = 0; i < 10; i++)
{
nums[i] = rand() % 100 + 1; // 生成1~100之间的随机数
for(j = 0; j < i; j++)
{
if(nums[j] == nums[i]) // 判断是否已经生成过该数
{
i--;
break;
}
}
}
printf("生成的10个不同数字为:");
for(i = 0; i < 10; i++)
{
printf("%d ", nums[i]);
}
return 0;
}
```
希望能帮到您!
相关问题
如何用C语言随机生成十万个数字
可以使用标准库函数rand()来生成随机数,然后使用循环生成十万个数字,并将它们存储到一个数组中。
示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define N 100000
int main() {
int arr[N];
int i;
// 设置随机数种子
srand(time(NULL));
// 生成十万个随机数
for (i = 0; i < N; i++) {
arr[i] = rand();
}
// 输出前十个数字
for (i = 0; i < 10; i++) {
printf("%d\n", arr[i]);
}
return 0;
}
```
在上面的代码中,使用了time()函数来获取当前时间作为随机数的种子,保证每次运行程序生成的随机数不同。生成的十万个随机数保存在数组arr中,并输出前十个数字。
c语言随机生成一个数字
在C语言中,你可以使用`<stdlib.h>`库中的`rand()`函数和`srand()`函数来生成随机数。首先,你需要设置随机数种子,通常会用当前时间或者其他不可预测的值,确保每次程序运行结果不同:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h> // 引入time库,用于获取当前时间作为随机种子
int main() {
// 设置随机数种子,这里使用当前系统时间
srand(time(0));
// 使用rand()生成随机数,范围通常是0到RAND_MAX(包含两端)
int random_num = rand();
printf("随机生成的数字是:%d\n", random_num);
return 0;
}
```
如果你想生成一定范围内的随机整数,可以结合`%`运算符来限制范围,例如生成1到100之间的随机数:
```c
// 生成1到100之间的随机数
int random_numInRange = (rand() % 100) + 1; // RAND_MAX通常是32767,取模后得到0-99,加上1即得1-100
```
阅读全文
相关推荐
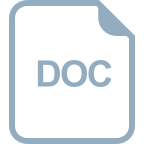
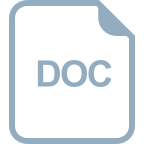
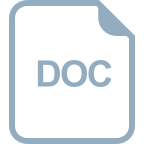













