用python实现感知器的 AND 和 OR 操作算法
时间: 2024-05-15 21:19:42 浏览: 76
下面是Python实现感知器的AND和OR操作算法的示例代码:
```python
class Perceptron:
def __init__(self, input_num, activator):
self.activator = activator
self.weights = [0.0] * input_num
self.bias = 0.0
def __str__(self):
return 'weights: %s, bias: %f' % (self.weights, self.bias)
def predict(self, input_vec):
return self.activator(
reduce(lambda a, b: a + b,
map(lambda x, w: x * w,
input_vec, self.weights)) + self.bias)
def train(self, input_vecs, labels, iteration, rate):
for i in range(iteration):
self._one_iteration(input_vecs, labels, rate)
def _one_iteration(self, input_vecs, labels, rate):
samples = zip(input_vecs, labels)
for (input_vec, label) in samples:
output = self.predict(input_vec)
self._update_weights(input_vec, output, label, rate)
def _update_weights(self, input_vec, output, label, rate):
delta = label - output
self.weights = list(map(
lambda x, w: w + rate * delta * x,
input_vec, self.weights))
self.bias += rate * delta
def f(x):
return 1 if x > 0 else 0
def get_training_dataset():
input_vecs = [[1, 1], [1, 0], [0, 1], [0, 0]]
labels = [1, 0, 0, 0]
return input_vecs, labels
def train_and_perceptron():
input_vecs, labels = get_training_dataset()
p = Perceptron(2, f)
p.train(input_vecs, labels, 10, 0.1)
return p
def train_or_perceptron():
input_vecs, labels = get_training_dataset()
p = Perceptron(2, f)
p.train(input_vecs, labels, 10, 0.1)
return p
if __name__ == '__main__':
and_perceptron = train_and_perceptron()
print(and_perceptron)
print('1 and 1 = %d' % and_perceptron.predict([1, 1]))
print('1 and 0 = %d' % and_perceptron.predict([1, 0]))
print('0 and 1 = %d' % and_perceptron.predict([0, 1]))
print('0 and 0 = %d' % and_perceptron.predict([0, 0]))
or_perceptron = train_or_perceptron()
print(or_perceptron)
print('1 or 1 = %d' % or_perceptron.predict([1, 1]))
print('1 or 0 = %d' % or_perceptron.predict([1, 0]))
print('0 or 1 = %d' % or_perceptron.predict([0, 1]))
print('0 or 0 = %d' % or_perceptron.predict([0, 0]))
```
在该示例代码中,我们定义了一个Perceptron类,其中包含了感知器的权重、偏置、预测和训练方法。我们还定义了一个激活函数f,该函数在输入大于0时返回1,在输入小于等于0时返回0。我们也定义了一个get_training_dataset函数,该函数返回我们的训练数据集。我们使用train_and_perceptron函数和train_or_perceptron函数来训练AND感知器和OR感知器。最后,在主函数中我们用训练出的感知器来测试它们的预测能力。
阅读全文
相关推荐
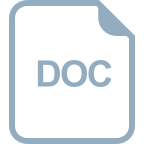
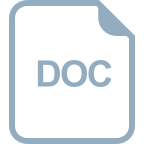
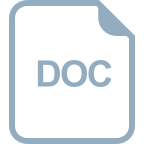



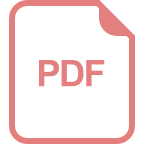



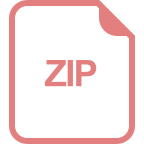


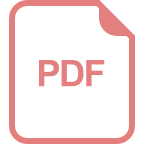
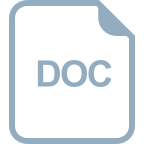
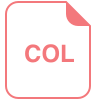
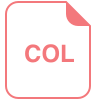
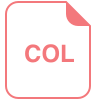
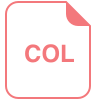