flink 批处理写入数据库
时间: 2023-07-25 12:06:58 浏览: 52
在 Flink 批处理中将数据写入数据库可以通过 Flink 的 `OutputFormat` 接口来实现。下面是一个简单的示例,假设有一个数据集 `DataSet<Tuple2<String, Integer>>`,其中每个元素是一个字符串和一个整数,需要将其写入到 MySQL 数据库中:
1. 首先需要引入对应的依赖,例如:
```xml
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.25</version>
</dependency>
```
2. 然后定义一个实现了 `OutputFormat` 接口的类,例如:
```java
public class MySQLOutputFormat implements OutputFormat<Tuple2<String, Integer>> {
private Connection connection;
private PreparedStatement statement;
@Override
public void configure(Configuration configuration) {
// 获取数据库连接等配置信息
String jdbcUrl = configuration.getString("jdbc.url", "jdbc:mysql://localhost:3306/test");
String username = configuration.getString("jdbc.username", "root");
String password = configuration.getString("jdbc.password", "root");
try {
connection = DriverManager.getConnection(jdbcUrl, username, password);
statement = connection.prepareStatement("INSERT INTO table_name (col1, col2) VALUES (?, ?)");
} catch (SQLException e) {
e.printStackTrace();
}
}
@Override
public void open(int i, int i1) throws IOException {
// 打开连接,并设置事务等配置信息
try {
connection.setAutoCommit(false);
} catch (SQLException e) {
e.printStackTrace();
}
}
@Override
public void writeRecord(Tuple2<String, Integer> tuple2) throws IOException {
// 将数据写入 PreparedStatement 中
try {
statement.setString(1, tuple2.f0);
statement.setInt(2, tuple2.f1);
statement.addBatch();
} catch (SQLException e) {
e.printStackTrace();
}
}
@Override
public void close() throws IOException {
// 执行批量写入,并提交事务
try {
statement.executeBatch();
connection.commit();
} catch (SQLException e) {
e.printStackTrace();
} finally {
// 关闭连接等资源
try {
if (statement != null) {
statement.close();
}
if (connection != null) {
connection.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
```
3. 最后在 Flink 程序中使用该类进行数据写入,例如:
```java
DataSet<Tuple2<String, Integer>> dataSet = ... // 获取数据集
Configuration config = new Configuration();
config.setString("jdbc.url", "jdbc:mysql://localhost:3306/test");
config.setString("jdbc.username", "root");
config.setString("jdbc.password", "root");
dataSet.output(new MySQLOutputFormat()).configure(config);
env.execute();
```
以上是一个简单的示例,需要根据具体情况进行适当的调整。
相关推荐
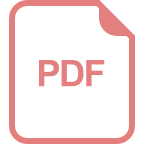














