vue3有多张图片,根据左右箭头点击去切换图片,类似于轮播
时间: 2023-08-22 13:08:58 浏览: 113
假设你有三张图片,分别为 `img1.jpg`、`img2.jpg` 和 `img3.jpg`,并且有两个按钮 `prev` 和 `next`,点击这两个按钮可以切换这三张图片。你可以使用 Vue 3 的组件实现如下:
HTML:
```html
<template>
<div>
<button @click="prev">上一张</button>
<button @click="next">下一张</button>
<div class="images" ref="images">
<img v-for="(img, index) in imgList" :key="index" :src="img" />
</div>
</div>
</template>
```
CSS:
```css
.images {
display: flex;
flex-wrap: nowrap;
overflow-x: hidden;
}
.images img {
flex-shrink: 0;
width: 100%;
}
```
JavaScript:
```javascript
import { ref } from "vue";
export default {
setup() {
const imgList = ["img1.jpg", "img2.jpg", "img3.jpg"];
const imgCount = imgList.length;
const currentIndex = ref(0);
const images = ref(null);
function prev() {
currentIndex.value = (currentIndex.value - 1 + imgCount) % imgCount;
images.value.style.transform = `translateX(${-currentIndex.value * 100}%)`;
}
function next() {
currentIndex.value = (currentIndex.value + 1) % imgCount;
images.value.style.transform = `translateX(${-currentIndex.value * 100}%)`;
}
return {
imgList,
currentIndex,
prev,
next,
images,
};
},
};
```
首先,使用 `import` 命令导入 Vue 3 的 `ref` 函数。然后,在组件的 `setup` 函数中,定义一个数组 `imgList` 来存储图片的路径,以及一个整数 `imgCount` 来记录图片的数量。使用 `ref` 函数定义一个响应式变量 `currentIndex` 来记录当前显示的图片的索引,初始值为 `0`。使用 `ref` 函数定义一个响应式变量 `images` 来引用图片容器的 DOM 元素。定义两个函数 `prev` 和 `next` 分别用于处理上一张和下一张按钮的点击事件。当上一张按钮被点击时,将 `currentIndex` 减 `1`,如果 `currentIndex` 小于 `0`,则将其加上图片数量,然后根据 `currentIndex` 来计算图片容器的 `transform` 属性的值,从而实现向左滑动的效果。当下一张按钮被点击时,将 `currentIndex` 加 `1`,并根据 `currentIndex` 来计算图片容器的 `transform` 属性的值,从而实现向右滑动的效果。注意,在计算 `currentIndex` 时,使用了取模运算,这样可以实现循环切换图片的效果。最后,将 `imgList`、`currentIndex`、`prev`、`next` 和 `images` 等变量和函数暴露给模板系统。在模板中,使用 `v-for` 指令和 `img` 元素来循环渲染图片,使用 `ref` 指令来引用图片容器的 DOM 元素。另外,使用了 CSS3 的 Flexbox 布局来实现图片容器的自适应布局和水平滑动效果。
相关推荐
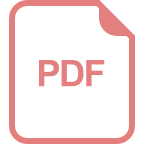
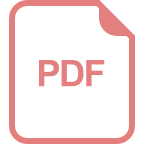














