输入n个朋友的信息,包括姓名、生日、电话号码,本题要求编写程序,按照年龄从大到小的顺序依次输出通讯录。题目保证所有人的生日均不相同
时间: 2023-05-31 10:18:36 浏览: 227
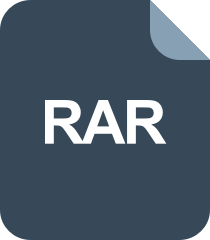
GuessBirthDate.rar_Visual_C++_
### 回答1:
可以使用 Python 编写一个程序,首先定义一个存储朋友信息的列表,每个元素为一个字典,存储姓名、生日、电话号码的信息。然后读入 n 个朋友的信息,将信息存入列表中。最后,将列表按照生日的先后顺序排序,从大到小输出通讯录。代码示例如下:
```
def sort_by_birthday(elem):
return elem['birthday']
friends = []
n = int(input('请输入朋友个数:'))
for i in range(n):
name = input('请输入姓名:')
birthday = input('请输入生日:')
phone = input('请输入电话号码:')
friends.append({'name': name, 'birthday': birthday, 'phone': phone})
friends.sort(key=sort_by_birthday, reverse=True)
print('通讯录:')
for friend in friends:
print('姓名:%s,生日:%s,电话号码:%s' % (friend['name'], friend['birthday'], friend['phone']))
```
### 回答2:
这道题目要求我们编写一个程序,输入n个朋友的信息,包括姓名、生日、电话号码,然后按照年龄从大到小的顺序依次输出通讯录。为了实现这个功能,我们可以采用以下步骤:
1. 定义一个结构体,用于存储每个朋友的信息,包括姓名、生日和电话号码。可以定义如下:
struct Friend {
string name; // 姓名
string birthday; // 生日
string phone; // 电话号码
};
2. 定义一个比较函数,用于按照年龄从大到小排序。我们可以根据生日计算每个朋友的年龄,然后将年龄较大的朋友排在前面。比较函数可以定义如下:
bool cmp(Friend f1, Friend f2) {
// 计算年龄
int age1 = // 计算f1的年龄
int age2 = // 计算f2的年龄
// 按照年龄从大到小排序
return age1 > age2;
}
3. 输入n个朋友的信息,并将其存储到一个数组中。可以使用循环来读入每个朋友的信息,然后将其存储到数组中。输入代码可以定义如下:
int n; // 朋友的个数
cin >> n; // 输入n
Friend friends[n]; // 定义一个数组来存储朋友的信息
for (int i = 0; i < n; i++) {
// 读入每个朋友的信息
cin >> friends[i].name >> friends[i].birthday >> friends[i].phone;
}
4. 使用sort函数按照年龄从大到小排序。可以将存储朋友信息的数组和比较函数作为参数传递给sort函数,然后使用迭代器输出排好序后的通讯录。排序和输出代码可以定义如下:
sort(friends, friends + n, cmp); // 按照年龄从大到小排序
for (auto it = friends; it != friends + n; it++) {
// 输出排序后的通讯录
cout << it->name << " " << it->birthday << " " << it->phone << endl;
}
综上所述,通过以上步骤,我们可以编写一个程序,按照年龄从大到小的顺序依次输出通讯录。
### 回答3:
在编写程序前,需要先明确一下数据结构。本题要求按照年龄从大到小的顺序依次输出通讯录,因此需要一个用于存储朋友信息的结构体,以及一个用于存储所有朋友信息的数组。具体代码实现如下:
```
#include <iostream>
#include <algorithm>
using namespace std;
struct Friend {
string name;
string birthday;
string phone;
int age;
} friends[100];
bool cmp(Friend f1, Friend f2) {
return f1.age > f2.age;
}
int main() {
int n;
cin >> n;
for (int i = 0; i < n; i++) {
cin >> friends[i].name >> friends[i].birthday >> friends[i].phone;
friends[i].age = 2021 - stoi(friends[i].birthday.substr(0, 4));
}
sort(friends, friends + n, cmp);
for (int i = 0; i < n; i++) {
cout << friends[i].name << " " << friends[i].birthday << " " << friends[i].phone << endl;
}
return 0;
}
```
程序首先定义了一个结构体Friend,包括姓名、生日、电话号码和年龄。接下来定义了一个用于存储所有朋友信息的数组friends,最大长度为100。在主函数中,先读入朋友个数n,然后循环读入每个朋友的信息,并计算其年龄。注意年龄需要通过出生年份计算,可以通过string的substr方法截取出生年份。
接着利用STL提供的sort函数,按照年龄从大到小的顺序对所有朋友进行排序。排序使用了一个自定义比较函数cmp,其中"f1.age > f2.age"表示按年龄从大到小排序。最后循环输出排序后的朋友信息即可。
综上,本题需要利用结构体存储朋友信息,通过计算年龄和排序功能实现按照年龄从大到小的顺序输出通讯录。
阅读全文
相关推荐
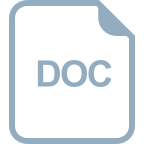
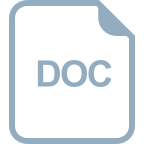
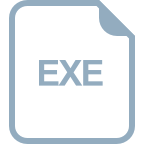





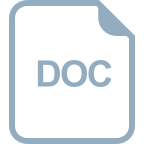
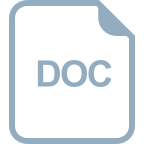
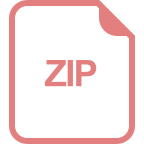
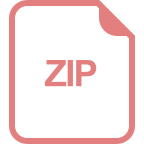
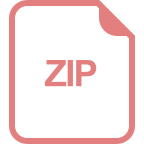
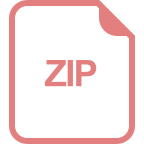
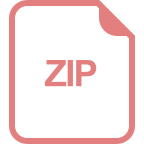
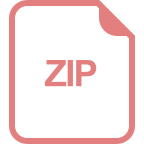
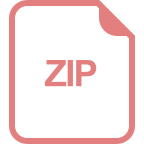
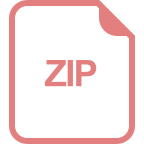