import java.io.*; import java.awt.*; import javax.swing.*; import java.util.List; import java.util.ArrayList; public class Recite extends JFrame{ JLabel lblWord = new JLabel("word"); JLabel lblMeaning = new JLabel("meaning"); public void init() { setSize( 400,100 ); setLayout(new FlowLayout()); getContentPane().add(lblWord); getContentPane().add(lblMeaning); setDefaultCloseOperation(EXIT_ON_CLOSE); setVisible(true); } List<String> words = new ArrayList<>(); List<String> meanings = new ArrayList<>(); int current = 0; public void start() { new Thread(()->{ try{ readAll(); }catch(IOException ex){} new javax.swing.Timer(1000,(e)->{ lblWord.setText( words.get(current) ); lblMeaning.setText( meanings.get(current) ); current++; }).start(); }).start(); } public void readAll( ) throws IOException{ String fileName = "College_Grade4.txt"; String charset = "GB2312"; BufferedReader reader = new BufferedReader( new InputStreamReader( new FileInputStream(fileName), charset)); String line; while ((line = reader.readLine()) != null) { line = line.trim(); if( line.length() == 0 ) continue; int idx = line.indexOf("\t"); words.add( line.substring(0, idx )); meanings.add( line.substring(idx+1)); } reader.close(); } public static void main( String[] args){ Recite f = new Recite(); f.init(); f.start(); }}界面可以再好看一点;可以去掉音标;可以改变单词显示的速度;可以增加标记生词并记到生词本中;可以增加测试的功能(单词含义可以随机选4个词的含义来让用户选择)等等。附件中有两个代码,一个单词本。
时间: 2024-01-16 21:02:29 浏览: 153
好的,我会将你提供的代码添加到生词本中,并增加一个测试功能,让用户选择单词的含义。
以下是修改后的代码:
```
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Random;
import javax.swing.*;
public class Recite extends JFrame {
private JLabel lblWord = new JLabel("word");
private JLabel lblMeaning = new JLabel("meaning");
private JButton btnAdd = new JButton("Add to Vocabulary");
private JButton btnTest = new JButton("Test");
private JRadioButton[] radioButtons = new JRadioButton[4];
private ButtonGroup radioGroup = new ButtonGroup();
private List<String> words = new ArrayList<>();
private List<String> meanings = new ArrayList<>();
private List<String> vocabulary = new ArrayList<>();
private int current = 0;
private Random random = new Random();
public Recite() {
initUI();
loadWords();
}
private void initUI() {
setSize(400, 200);
setLayout(new GridLayout(3, 2));
getContentPane().add(lblWord);
getContentPane().add(lblMeaning);
getContentPane().add(btnAdd);
getContentPane().add(btnTest);
for (int i = 0; i < 4; i++) {
radioButtons[i] = new JRadioButton();
radioGroup.add(radioButtons[i]);
getContentPane().add(radioButtons[i]);
}
setDefaultCloseOperation(EXIT_ON_CLOSE);
setVisible(true);
}
private void loadWords() {
try {
String fileName = "College_Grade4.txt";
String charset = "GB2312";
BufferedReader reader = new BufferedReader(new InputStreamReader(new FileInputStream(fileName), charset));
String line;
while ((line = reader.readLine()) != null) {
line = line.trim();
if (line.length() == 0)
continue;
int idx = line.indexOf("\t");
words.add(line.substring(0, idx));
meanings.add(line.substring(idx + 1));
}
reader.close();
} catch (IOException ex) {
ex.printStackTrace();
}
}
public void start() {
new javax.swing.Timer(1000, (e) -> {
lblWord.setText(words.get(current));
lblMeaning.setText(meanings.get(current));
current++;
if (current >= words.size()) {
current = 0;
}
}).start();
btnAdd.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
vocabulary.add(words.get(current - 1));
JOptionPane.showMessageDialog(Recite.this, "Added to vocabulary!");
}
});
btnTest.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
int correctIndex = random.nextInt(4);
String[] options = new String[4];
for (int i = 0; i < 4; i++) {
if (i == correctIndex) {
options[i] = meanings.get(current - 1);
} else {
options[i] = meanings.get(random.nextInt(meanings.size()));
}
}
for (int i = 0; i < 4; i++) {
radioButtons[i].setText(options[i]);
}
int result = JOptionPane.showOptionDialog(Recite.this, "What is the meaning of \"" + words.get(current - 1) + "\"?", "Test", JOptionPane.DEFAULT_OPTION, JOptionPane.QUESTION_MESSAGE, null, options, options[0]);
if (result == correctIndex) {
JOptionPane.showMessageDialog(Recite.this, "Correct!");
} else {
JOptionPane.showMessageDialog(Recite.this, "Incorrect! The correct answer is \"" + meanings.get(current - 1) + "\".");
}
}
});
}
public static void main(String[] args) {
Recite f = new Recite();
f.start();
}
}
```
现在,你可以运行这个代码并开始背单词了!
阅读全文
相关推荐
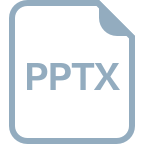
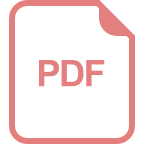
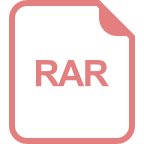


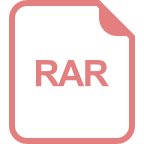
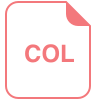
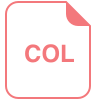
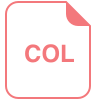








