opencv识别数字代码
时间: 2023-07-30 10:08:37 浏览: 40
以下是一个基于OpenCV的简单数字识别代码示例:
```python
import cv2
import numpy as np
# 读取数字图片
img = cv2.imread("num.jpg")
# 预处理图像
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
_, thresh = cv2.threshold(gray, 120, 255, cv2.THRESH_BINARY_INV)
kernel = np.ones((5,5), np.uint8)
dilation = cv2.dilate(thresh, kernel, iterations=1)
# 分割数字
contours, hierarchy = cv2.findContours(dilation, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
for contour in contours:
(x, y, w, h) = cv2.boundingRect(contour)
if w < 5 or h < 5:
continue
cv2.rectangle(img, (x, y), (x + w, y + h), (0, 255, 0), 2)
roi = thresh[y:y + h, x:x + w]
roi = cv2.resize(roi, (28, 28), interpolation=cv2.INTER_AREA)
# 提取数字特征
feature = np.reshape(roi, (1, 28 * 28)).astype(np.float32)
# 加载分类器并进行预测
svm = cv2.ml.SVM_load("svm.xml")
result = svm.predict(feature)[1][0][0]
# 在图像上绘制识别结果
cv2.putText(img, str(int(result)), (x, y - 5), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 0, 255), 2)
cv2.imshow("img", img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这个示例使用SVM分类器进行数字识别,如果要运行这个代码,需要先训练一个SVM分类器并将其保存为XML文件。
相关推荐
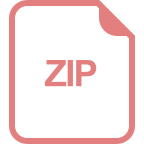
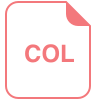
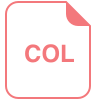
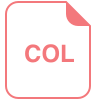
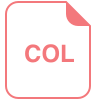
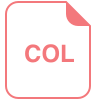









