np.zeros((n - 1,))
时间: 2023-05-29 17:02:59 浏览: 158
This code creates a numpy array of zeros with n-1 elements. The shape of the array is (n-1, ). The dtype of the array is float64 by default.
For example, if n=5, then the code creates an array of shape (4,) with all elements set to 0.0:
```
import numpy as np
n = 5
arr = np.zeros((n - 1,))
print(arr)
Output:
[0. 0. 0. 0.]
```
相关问题
Y1定义为LABEL输出,Y1 = np.zeros((self.n - s, 1)), Y2是特征输出 :Y2 = np.zeros((self.n - s, self.m - 1))
好的,按照您的要求,修改后的函数如下所示:
```python
def _slice_multi(self):
# self.n 是数据集总行数
# self.m 是列
s = self.ps * self.skip + self.Ck - 1 + self.h - 1
X1 = np.zeros((self.n - s, self.w, self.m)) # 短期数据集
X2 = np.zeros((self.n - s, self.ps * self.Ck, self.m - 1)) # 长期数据集
Y1 = np.zeros((self.n - s, 1)) # LABEL输出
Y2 = np.zeros((self.n - s, self.m - 1)) # 特征输出
for i in range(s, self.n):
t = i - self.h + 1
X1[i - s] = self.raw[t - self.w:t].copy()
idx = []
for k in range(self.ps):
# 执行self.ps次,每次间隔self.skip长,取self.Ck=6, total = self.ps * self.Ck
idx = list(range(t - self.Ck - k * self.skip, t - k * self.skip)) + idx
idx = np.array(idx, dtype=int)
X2[i - s] = self.raw[idx, :-1].copy() # 去掉最后一列
Y1[i - s] = self.raw[i, -1].copy() # 最后一列为LABEL
Y2[i - s] = self.raw[i, :-1].copy() # 去掉最后一列
# 返回特征和标签
return (X1, X2), (Y1, Y2)
```
修改后的函数输出了两个元组,每个元组包含了两个数组,分别对应两个输出。其中Y1为LABEL输出,维度为 (n-s, 1),Y2为特征输出,维度为 (n-s, m-1)。
请把这段代码改成jupyter环境中适用的代码:import numpy as np import matplotlib.pyplot as plt def gauss_1d_c(): # Basis of 1D Gaussians, with varying centers, # all with same width n = 101 nu = 1.0 h2m = 0.5 # Calculate the centers for the Gaussians s = np.zeros(n) for i in range(n): s[i] = -25.0 + (i - 1) * 0.5 # Setup the Hamiltonian h = np.zeros((n, n)) o = np.zeros((n, n)) f = np.zeros((n, n)) for i in range(n): for j in range(n): ss = (s[i] - s[j])**2 o[i, j] = np.exp(-0.5 * nu * ss) t = np.exp(-0.5 * nu * ss) * nu * h2m * (1.0 - nu * ss) p = 0.5 * np.exp(-0.5 * nu * ss) * 0.25 * (1.0 / nu + (s[i] + s[j])**2) h[i, j] = t + p # Diagonalize f=np.dot(h,np.linalg.inv(o)) eigenvalues, eigenvectors = np.linalg.eig(f) print(eigenvalues) def paint_eigenvalues(): # 在图中绘制特征值 plt.plot(eigenvalues) # 设置图的标题和坐标轴标签 plt.title("Eigenvalues") plt.xlabel("Index") plt.ylabel("Value") # 显示图 plt.show() gauss_1d_c()
代码已经是适用于jupyter环境的,但如果要在jupyter中显示图像,需要添加以下代码:
```python
%matplotlib inline
```
将其添加到代码的开头即可。完整的代码如下所示:
```python
%matplotlib inline
import numpy as np
import matplotlib.pyplot as plt
def gauss_1d_c():
# Basis of 1D Gaussians, with varying centers,
# all with same width
n = 101
nu = 1.0
h2m = 0.5
# Calculate the centers for the Gaussians
s = np.zeros(n)
for i in range(n):
s[i] = -25.0 + (i - 1) * 0.5
# Setup the Hamiltonian
h = np.zeros((n, n))
o = np.zeros((n, n))
f = np.zeros((n, n))
for i in range(n):
for j in range(n):
ss = (s[i] - s[j])**2
o[i, j] = np.exp(-0.5 * nu * ss)
t = np.exp(-0.5 * nu * ss) * nu * h2m * (1.0 - nu * ss)
p = 0.5 * np.exp(-0.5 * nu * ss) * 0.25 * (1.0 / nu + (s[i] + s[j])**2)
h[i, j] = t + p
# Diagonalize
f=np.dot(h,np.linalg.inv(o))
eigenvalues, eigenvectors = np.linalg.eig(f)
print(eigenvalues)
def paint_eigenvalues():
# 在图中绘制特征值
plt.plot(eigenvalues)
# 设置图的标题和坐标轴标签
plt.title("Eigenvalues")
plt.xlabel("Index")
plt.ylabel("Value")
# 显示图
plt.show()
gauss_1d_c()
paint_eigenvalues()
```
注意,需要先运行gauss_1d_c()函数,才能在paint_eigenvalues()函数中使用eigenvalues变量。
阅读全文
相关推荐
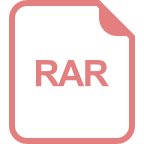
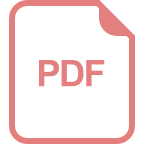
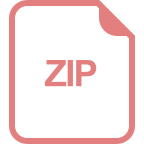













