gdiplusToken,gdiplusStartupInput这两个的定义是什么
时间: 2024-04-06 11:28:52 浏览: 78
gdiplusToken和gdiplusStartupInput是GDI+图形库中的数据类型,用于在应用程序中初始化和关闭GDI+图形库。
gdiplusStartupInput是一个结构体,包含了GDI+图形库的启动参数,例如GDI+版本号、是否启用调试模式等。gdiplusToken是一个整型变量,用于存储GDI+图形库的初始化状态。当GDI+成功启动后,gdiplusToken会被分配一个非零值,表示GDI+已经成功初始化;当关闭GDI+图形库时,需要使用gdiplusToken来释放相关资源。
相关问题
4.位图直方图均衡 a)涉及知识点:文件读写、结构体定义、基本图像处理算法、命令行参数 b)要求: 编写一个程序,可以在命令行输入参数,完成指定文件的直方图均衡,并存储到新文件,命令行参数如下 hist file1.bmp file2.bmp
好的,这是一个具体的问题。这里提供一个 C语言的实现示例。请注意,这个示例代码中使用了 Windows.h 和 Gdiplus.h 这两个库来读取位图文件和存储位图文件。如果你在其他操作系统上运行此代码,可能需要使用不同的库来实现相应的功能。
```c
#include <stdio.h>
#include <stdlib.h>
#include <Windows.h>
#include <Gdiplus.h>
using namespace Gdiplus;
void HistogramEqualization(Bitmap* bmp, int* r_hist, int* g_hist, int* b_hist)
{
int width = bmp->GetWidth();
int height = bmp->GetHeight();
int total_pixels = width * height;
// 计算 RGB 直方图
for (int y = 0; y < height; y++)
{
for (int x = 0; x < width; x++)
{
Color color;
bmp->GetPixel(x, y, &color);
r_hist[color.GetR()]++;
g_hist[color.GetG()]++;
b_hist[color.GetB()]++;
}
}
// 计算 RGB 累积直方图
int r_cum_hist[256] = { 0 };
int g_cum_hist[256] = { 0 };
int b_cum_hist[256] = { 0 };
r_cum_hist[0] = r_hist[0];
g_cum_hist[0] = g_hist[0];
b_cum_hist[0] = b_hist[0];
for (int i = 1; i < 256; i++)
{
r_cum_hist[i] = r_cum_hist[i - 1] + r_hist[i];
g_cum_hist[i] = g_cum_hist[i - 1] + g_hist[i];
b_cum_hist[i] = b_cum_hist[i - 1] + b_hist[i];
}
// 计算 RGB 均衡化映射表
int r_map[256] = { 0 };
int g_map[256] = { 0 };
int b_map[256] = { 0 };
for (int i = 0; i < 256; i++)
{
r_map[i] = (int)(255.0f * r_cum_hist[i] / total_pixels + 0.5f);
g_map[i] = (int)(255.0f * g_cum_hist[i] / total_pixels + 0.5f);
b_map[i] = (int)(255.0f * b_cum_hist[i] / total_pixels + 0.5f);
}
// 应用 RGB 均衡化映射表
for (int y = 0; y < height; y++)
{
for (int x = 0; x < width; x++)
{
Color color;
bmp->GetPixel(x, y, &color);
int r = r_map[color.GetR()];
int g = g_map[color.GetG()];
int b = b_map[color.GetB()];
bmp->SetPixel(x, y, Color(r, g, b));
}
}
}
int main(int argc, char* argv[])
{
if (argc != 3)
{
printf("Usage: hist input_file output_file\n");
return 1;
}
GdiplusStartupInput gdiplusStartupInput;
ULONG_PTR gdiplusToken;
GdiplusStartup(&gdiplusToken, &gdiplusStartupInput, NULL);
// 读取输入文件
Bitmap* bmp = new Bitmap(ToWideChar(argv[1]).c_str());
if (bmp->GetLastStatus() != Ok)
{
printf("Failed to read input file %s\n", argv[1]);
return 1;
}
// 创建输出文件
Bitmap* new_bmp = new Bitmap(bmp->GetWidth(), bmp->GetHeight(), PixelFormat24bppRGB);
if (new_bmp->GetLastStatus() != Ok)
{
printf("Failed to create output file %s\n", argv[2]);
return 1;
}
// 直方图均衡化
int r_hist[256] = { 0 };
int g_hist[256] = { 0 };
int b_hist[256] = { 0 };
HistogramEqualization(bmp, r_hist, g_hist, b_hist);
// 保存输出文件
Graphics* graphics = Graphics::FromImage(new_bmp);
graphics->DrawImage(bmp, 0, 0);
if (new_bmp->Save(ToWideChar(argv[2]).c_str(), &CLSID_Bmp, NULL) != Ok)
{
printf("Failed to save output file %s\n", argv[2]);
return 1;
}
// 释放资源
delete graphics;
delete new_bmp;
delete bmp;
GdiplusShutdown(gdiplusToken);
return 0;
}
```
在这个示例代码中,我们使用了 `Bitmap` 类来读取和存储位图文件,使用了 `Color` 类来获取位图中每个像素的 RGB 值。我们先读取输入文件,然后创建一个与输入文件大小相同的新位图文件。接着,我们计算输入文件中的 RGB 直方图,并根据直方图计算出 RGB 均衡化映射表。最后,我们将均衡化映射表应用到输入文件的每个像素上,并将处理后的结果保存到输出文件中。
注意,这个代码中使用了一个 `ToWideChar` 函数,这个函数是用来将命令行参数从 `char*` 类型转换成 `wchar_t*` 类型的。这是因为 `Bitmap` 类的构造函数和 `Save` 函数都需要 `wchar_t*` 类型的字符串作为参数。如果你在使用这个代码时遇到了编译错误,请检查是否需要修改这个函数的实现。
希望这个示例代码可以帮助你完成这个作业。如果你有任何问题或疑问,欢迎随时提出。
阅读全文
相关推荐
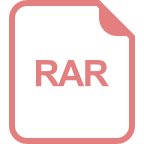
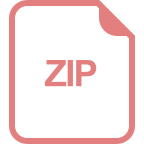
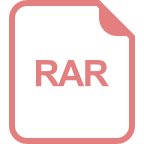
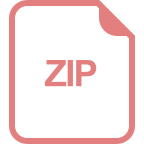
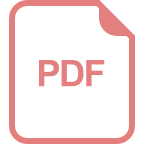
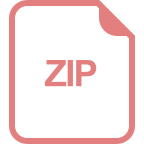
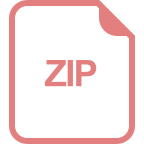
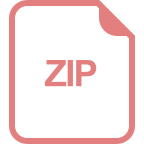
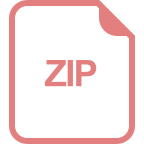
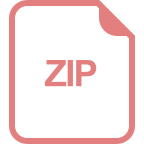
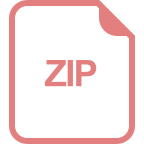