补齐以下代码#include"stdio.h" #include"stdlib.h" typedef struct node{ char data; struct node *next; }Node; void creatList(Node * head); int delByPos(Node *h,int pos); void output(Node *h); void destroyList(Node *h); main() { Node *head=(Node *)malloc(sizeof(Node)); //创建头结点,不存放有效数据 head->next=NULL; int pos; //尾插法创建链表 creatList( head); //输出链表 output(head); //输入删除数据信息 scanf("%d",&pos); //删除数据,成功则再输出链表,失败则报错 if(delByPos(head,pos)) output(head); else printf("delete by pos failed!\n"); //销毁链表 destroyList(head); } @@1 @@1
时间: 2024-01-16 08:04:26 浏览: 32
代码中缺少函数 `creatList`,需要补充。
以下是完整代码:
```
#include "stdio.h"
#include "stdlib.h"
typedef struct node{
char data;
struct node *next;
}Node;
void creatList(Node *head);
int delByPos(Node *h, int pos);
void output(Node *h);
void destroyList(Node *h);
int main() {
Node *head = (Node *)malloc(sizeof(Node)); // 创建头结点,不存放有效数据
head->next = NULL;
int pos;
// 尾插法创建链表
creatList(head);
// 输出链表
output(head);
// 输入删除数据信息
scanf("%d", &pos);
// 删除数据,成功则再输出链表,失败则报错
if (delByPos(head, pos))
output(head);
else
printf("delete by pos failed!\n");
// 销毁链表
destroyList(head);
return 0;
}
void creatList(Node *head) {
Node *p = head;
char data;
printf("请输入字符,以#结束:\n");
scanf("%c", &data);
while (data != '#') {
Node *newNode = (Node *)malloc(sizeof(Node));
newNode->data = data;
newNode->next = NULL;
p->next = newNode;
p = p->next;
scanf("%c", &data);
}
}
int delByPos(Node *h, int pos) {
Node *p = h;
int i = 0;
while (p != NULL && i < pos - 1) {
p = p->next;
i++;
}
if (p == NULL || p->next == NULL) {
return 0;
}
Node *q = p->next;
p->next = q->next;
free(q);
return 1;
}
void output(Node *h) {
Node *p = h->next;
while (p != NULL) {
printf("%c ", p->data);
p = p->next;
}
printf("\n");
}
void destroyList(Node *h) {
Node *p = h;
while (p != NULL) {
Node *q = p->next;
free(p);
p = q;
}
}
```
相关推荐
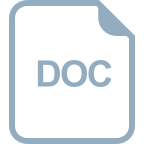
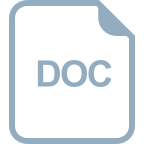
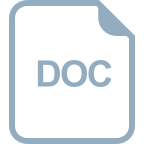










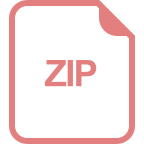
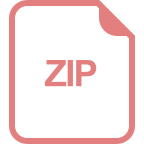