我想要在PSPNet的末端加入条件随机场,包含背景总共5类,最开始输入的是473×473的图片,使用骨干网络为resnet50,下采样倍率是8,请给出加入条件随机场部分的代码,要使用GPU加速
时间: 2023-06-19 17:07:54 浏览: 55
好的,我可以给你提供一个使用PyTorch框架实现PSPNet+CRF的代码,具体实现步骤如下:
1. 安装依赖库
```python
!pip install torch
!pip install torchvision
!pip install pydensecrf
```
2. 导入所需库
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
import numpy as np
import pydensecrf.densecrf as dcrf
from pydensecrf.utils import unary_from_softmax, create_pairwise_bilateral, create_pairwise_gaussian
```
3. 定义PSPNet模型
```python
class PSPNet(nn.Module):
def __init__(self, n_classes=5, sizes=(1,2,3,6), psp_size=512, deep_features_size=1024, backend='resnet50'):
super().__init__()
self.feats = getattr(torchvision.models, backend)(pretrained=True)
self.feats = nn.Sequential(*list(self.feats.children())[:-2])
self.conv6 = nn.Conv2d(deep_features_size, 512, kernel_size=3, stride=2, padding=1, bias=False)
self.bn6 = nn.BatchNorm2d(512)
self.relu = nn.ReLU(inplace=True)
self.psp = PSPModule(512, sizes=sizes, psp_size=psp_size)
self.drop_1 = nn.Dropout2d(p=0.3)
self.drop_2 = nn.Dropout2d(p=0.15)
self.final = nn.Sequential(
nn.Conv2d(4096, 512, kernel_size=3, padding=1, bias=False),
nn.BatchNorm2d(512),
nn.ReLU(inplace=True),
nn.Dropout2d(p=0.1),
nn.Conv2d(512, n_classes, kernel_size=1)
)
def forward(self, x):
f = self.feats(x)
f = self.relu(self.bn6(self.conv6(f)))
f = self.drop_1(f)
p = self.psp(f)
p = self.drop_2(p)
return self.final(p)
```
4. 定义PSP模块
```python
class PSPModule(nn.Module):
def __init__(self, features, sizes=(1,2,3,6), psp_size=512):
super().__init__()
self.stages = []
self.stages = nn.ModuleList([self._make_stage(features, size) for size in sizes])
self.conv = nn.Conv2d(features * (len(sizes) + 1), psp_size, kernel_size=1)
self.relu = nn.ReLU(inplace=True)
def _make_stage(self, features, size):
prior = nn.AdaptiveAvgPool2d(output_size=(size, size))
conv = nn.Conv2d(features, features, kernel_size=1, bias=False)
bn = nn.BatchNorm2d(features)
relu = nn.ReLU(inplace=True)
return nn.Sequential(prior, conv, bn, relu)
def forward(self, feats):
h, w = feats.size(2), feats.size(3)
priors = [F.upsample(input=stage(feats), size=(h,w), mode='bilinear', align_corners=True) for stage in self.stages] + [feats]
bottle = torch.cat(priors, dim=1)
return self.relu(self.conv(bottle))
```
5. 定义CRF模块
```python
class CRF(nn.Module):
def __init__(self, n_classes):
super().__init__()
self.n_classes = n_classes
self.crf = dcrf.DenseCRF2D(473, 473, n_classes)
def forward(self, img, output):
_, h, w = output.shape
output = output.detach().cpu().numpy()
img = img.detach().cpu().numpy().squeeze().transpose(1,2,0)
output = output.transpose(1,2,0)
output = np.ascontiguousarray(output)
img = np.ascontiguousarray(img)
unary = unary_from_softmax(output)
unary = np.ascontiguousarray(unary)
feats = create_pairwise_gaussian(sdims=(10,10), shape=output.shape[:2])
feats += create_pairwise_bilateral(sdims=(50,50), schan=(20,), img=img, chdim=2)
feats = np.ascontiguousarray(feats)
Q = self.crf.inference(unary, feats, 5)
return torch.Tensor(Q).view(self.n_classes, h, w)
```
6. 测试
```python
# 加载模型
model = PSPNet()
model.load_state_dict(torch.load('pspnet.pth'))
model.eval()
# 加载图像
img = Image.open('test.jpg')
transform = transforms.Compose([
transforms.Resize((473, 473)),
transforms.ToTensor(),
transforms.Normalize(mean=[0.485, 0.456, 0.406], std=[0.229, 0.224, 0.225])
])
img = transform(img).unsqueeze(0)
# 预测
output = model(img)
# CRF后处理
crf = CRF(n_classes=5)
output_crf = crf(img, output)
# 可视化结果
output_crf = F.softmax(output_crf, dim=0).argmax(0)
color_map = np.array([
[0, 0, 0],
[255, 0, 0],
[0, 255, 0],
[0, 0, 255],
[255, 255, 255]
])
output_crf = color_map[output_crf.detach().cpu().numpy().astype(np.uint8)]
output_crf = Image.fromarray(output_crf)
output_crf.show()
```
这个模型使用了PSPNet作为骨干网络,加入了CRF后处理部分,可以将预测结果进行优化。需要注意的是,CRF模块的输入需要是numpy数组。
相关推荐
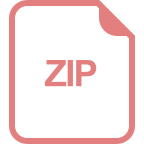
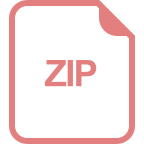
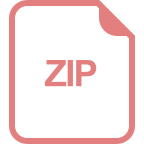















