每类人员的信息记录在“类名_info.txt”文件中,例如兼职技术人员的信息记录在 Technician_info.txt文件中。能够根据编号对每类人员的信息进行增加、查找、删除和修改。
时间: 2024-04-08 18:34:58 浏览: 11
是的,根据您的描述,每类人员的信息记录在以类名命名的文件中,例如兼职技术人员的信息记录在 Technician_info.txt 文件中。您可以使用文件操作来实现根据编号对每类人员信息进行增加、查找、删除和修改的功能。
下面是一个简单的示例代码,展示如何实现这些功能:
```cpp
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
// 增加人员信息
void addPerson(string fileName, string personInfo) {
ofstream outFile;
outFile.open(fileName, ios::app);
outFile << personInfo << endl;
outFile.close();
}
// 查找人员信息
void findPerson(string fileName, int personId) {
ifstream inFile;
inFile.open(fileName);
string line;
bool found = false;
while (getline(inFile, line)) {
// 假设每行记录的格式为:编号,姓名,年龄,...
// 您可以根据实际情况修改解析逻辑
int id = stoi(line.substr(0, line.find(',')));
if (id == personId) {
cout << "找到匹配的人员信息:" << line << endl;
found = true;
break;
}
}
if (!found) {
cout << "未找到匹配的人员信息。" << endl;
}
inFile.close();
}
// 删除人员信息
void deletePerson(string fileName, int personId) {
ifstream inFile;
inFile.open(fileName);
ofstream tempFile;
tempFile.open("temp.txt");
string line;
bool found = false;
while (getline(inFile, line)) {
// 假设每行记录的格式为:编号,姓名,年龄,...
// 您可以根据实际情况修改解析逻辑
int id = stoi(line.substr(0, line.find(',')));
if (id != personId) {
tempFile << line << endl;
} else {
cout << "已删除编号为 " << personId << " 的人员信息。" << endl;
found = true;
}
}
if (!found) {
cout << "未找到匹配的人员信息。" << endl;
}
inFile.close();
tempFile.close();
// 删除原文件并重命名临时文件
remove(fileName.c_str());
rename("temp.txt", fileName.c_str());
}
// 修改人员信息
void modifyPerson(string fileName, int personId, string newInfo) {
ifstream inFile;
inFile.open(fileName);
ofstream tempFile;
tempFile.open("temp.txt");
string line;
bool found = false;
while (getline(inFile, line)) {
// 假设每行记录的格式为:编号,姓名,年龄,...
// 您可以根据实际情况修改解析逻辑
int id = stoi(line.substr(0, line.find(',')));
if (id != personId) {
tempFile << line << endl;
} else {
tempFile << newInfo << endl;
cout << "已修改编号为 " << personId << " 的人员信息。" << endl;
found = true;
}
}
if (!found) {
cout << "未找到匹配的人员信息。" << endl;
}
inFile.close();
tempFile.close();
// 删除原文件并重命名临时文件
remove(fileName.c_str());
rename("temp.txt", fileName.c_str());
}
int main() {
string fileName = "Technician_info.txt";
// 增加人员信息
addPerson(fileName, "1001,张三,25,技术员");
// 查找人员信息
findPerson(fileName, 1001);
// 删除人员信息
deletePerson(fileName, 1001);
// 修改人员信息
modifyPerson(fileName, 1001, "1001,李四,28,高级技术员");
return 0;
}
```
请注意,上述代码仅为示例,您需要根据实际情况进行修改和完善。另外,为了简化示例,我假设每行记录的格式为:“编号,姓名,年龄,...”,您可以根据实际情况修改解析逻辑。
希望这个示例能够帮助您实现根据编号对每类人员信息进行增加、查找、删除和修改的功能。如果您有任何其他问题,请随时提问!
相关推荐
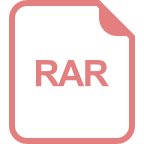
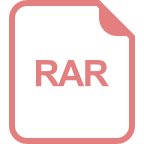
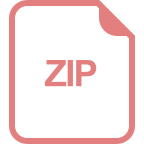














