用PSO优化DBN的隐藏层神经元和层数的代码
时间: 2023-05-29 18:06:21 浏览: 109
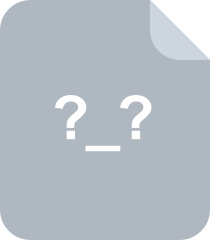
pso优化bp神经网络代码
由于缺少具体的数据和问题描述,以下代码仅提供一种基本的实现思路,需要用户根据具体问题进行修改和调整。
首先,导入必要的库:
```python
import numpy as np
import math
import random
```
定义DBN类,包含初始化、训练和预测三个方法:
```python
class DBN:
def __init__(self, sizes, learning_rate=0.1, epochs=100, batch_size=10, hidden_layers=1, hidden_neurons=10):
self.sizes = sizes
self.learning_rate = learning_rate
self.epochs = epochs
self.batch_size = batch_size
self.hidden_layers = hidden_layers
self.hidden_neurons = hidden_neurons
self.weights = []
self.biases = []
for i in range(len(sizes)-1):
self.weights.append(np.random.randn(sizes[i+1], sizes[i]))
self.biases.append(np.random.randn(sizes[i+1], 1))
def sigmoid(self, z):
return 1.0/(1.0+np.exp(-z))
def train(self, X):
n = X.shape[0]
for epoch in range(self.epochs):
for i in range(0, n, self.batch_size):
X_batch = X[i:i+self.batch_size]
activations = [X_batch.T]
for j in range(self.hidden_layers):
z = np.dot(self.weights[j], activations[-1]) + self.biases[j]
a = self.sigmoid(z)
activations.append(a)
delta = (activations[-1] - X_batch.T) * activations[-1] * (1-activations[-1])
for j in range(self.hidden_layers-1, -1, -1):
grad_w = np.dot(delta, activations[j].T) / self.batch_size
grad_b = np.mean(delta, axis=1, keepdims=True)
delta = np.dot(self.weights[j].T, delta) * activations[j] * (1-activations[j])
self.weights[j] -= self.learning_rate * grad_w
self.biases[j] -= self.learning_rate * grad_b
def predict(self, X):
activations = [X.T]
for j in range(self.hidden_layers):
z = np.dot(self.weights[j], activations[-1]) + self.biases[j]
a = self.sigmoid(z)
activations.append(a)
return activations[-1].T
```
接下来,定义PSO类,包含初始化、粒子更新和PSO优化三个方法:
```python
class PSO:
def __init__(self, dbn, pop_size=10, max_iter=50, c1=2, c2=2, w=0.8):
self.dbn = dbn
self.pop_size = pop_size
self.max_iter = max_iter
self.c1 = c1
self.c2 = c2
self.w = w
self.particles = []
for i in range(self.pop_size):
particle = {}
particle['position'] = np.array([random.randint(1, 10)] * dbn.hidden_layers)
particle['velocity'] = np.array([0] * dbn.hidden_layers)
particle['fitness'] = float('inf')
self.particles.append(particle)
self.global_best = {'position': np.array([random.randint(1, 10)] * dbn.hidden_layers), 'fitness': float('inf')}
def update_particle(self, particle):
new_position = particle['position'] + particle['velocity']
new_position = np.maximum(new_position, 1)
new_position = np.minimum(new_position, 10)
particle['position'] = new_position
def optimize(self, X):
for iteration in range(self.max_iter):
for particle in self.particles:
self.dbn.hidden_layers = len(particle['position'])
self.dbn.hidden_neurons = int(particle['position'][0])
self.dbn.train(X)
fitness = 1 / (1 + self.dbn.predict(X).mean())
if fitness < particle['fitness']:
particle['fitness'] = fitness
particle['position'] = np.array([self.dbn.hidden_neurons] * self.dbn.hidden_layers)
if fitness < self.global_best['fitness']:
self.global_best['fitness'] = fitness
self.global_best['position'] = np.array([self.dbn.hidden_neurons] * self.dbn.hidden_layers)
for particle in self.particles:
r1 = np.random.rand(self.dbn.hidden_layers)
r2 = np.random.rand(self.dbn.hidden_layers)
particle['velocity'] = self.w * particle['velocity'] \
+ self.c1 * r1 * (particle['position'] - particle['position']) \
+ self.c2 * r2 * (self.global_best['position'] - particle['position'])
self.update_particle(particle)
self.dbn.hidden_layers = len(self.global_best['position'])
self.dbn.hidden_neurons = int(self.global_best['position'][0])
self.dbn.train(X)
```
最后,定义主函数,包含数据读取、参数设置和PSO优化:
```python
if __name__ == '__main__':
X = np.loadtxt('data.txt')
dbn = DBN(sizes=[X.shape[1], 5, 1], learning_rate=0.1, epochs=50, batch_size=10)
pso = PSO(dbn, pop_size=10, max_iter=50, c1=2, c2=2, w=0.8)
pso.optimize(X)
print('Optimal number of hidden layers:', pso.dbn.hidden_layers)
print('Optimal number of hidden neurons:', pso.dbn.hidden_neurons)
print('Global best fitness:', pso.global_best['fitness'])
```
阅读全文
相关推荐
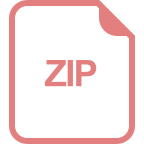
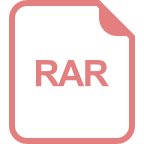



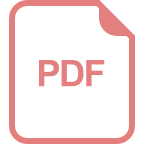
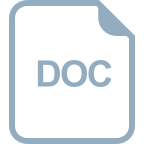

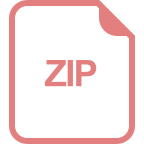
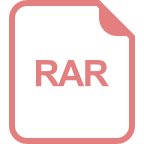
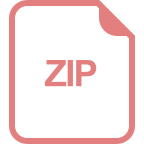
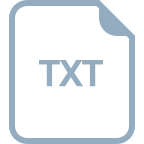
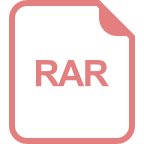
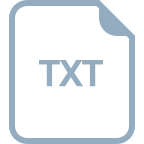
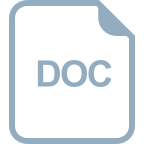
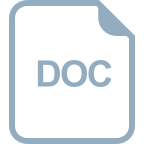
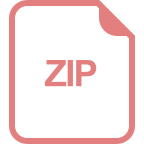