pycharm把h5文件转为图片
时间: 2023-05-12 15:04:42 浏览: 67
可以使用Python中的h5py库来读取h5文件,然后使用Pillow库将其转换为图片格式。以下是示例代码:
```python
import h5py
from PIL import Image
# 读取h5文件
with h5py.File('file.h5', 'r') as f:
data = f['dataset_name'][:]
# 转换为图片
img = Image.fromarray(data)
img.save('image.png')
```
注意,需要将`dataset_name`替换为h5文件中的数据集名称。
相关问题
用pycharm从h5文件中把AUU-sewer数据集转换为图片
你可以使用以下代码将AUU-sewer数据集从h5文件转换为图片:
```python
import h5py
import numpy as np
import cv2
# Load the h5 file
with h5py.File('path/to/auu-sewer.h5', 'r') as f:
images = np.array(f['images'])
labels = np.array(f['labels'])
# Convert the images to RGB format and save as png files
for i, image in enumerate(images):
image = cv2.cvtColor(image, cv2.COLOR_GRAY2RGB)
cv2.imwrite(f'path/to/save/image_{i}.png', image)
```
请注意,你需要将代码中的路径替换为你自己的路径。
.h5文件转换为.mat文件并保存在自定义的文件夹下的完整代码 pycharm
以下是一个示例代码,假设您已经安装了h5py和scipy库:
```python
import h5py
import scipy.io as sio
import os
# 定义h5文件路径
h5_file_path = './example.h5'
# 定义.mat文件保存路径
mat_file_path = './mat_files'
# 创建.mat文件保存目录
if not os.path.exists(mat_file_path):
os.makedirs(mat_file_path)
# 读取h5文件
h5_file = h5py.File(h5_file_path, 'r')
# 转换为mat格式并保存
for key in h5_file.keys():
mat_file_name = os.path.join(mat_file_path, key + '.mat')
sio.savemat(mat_file_name, {key: h5_file[key][:]})
```
这段代码将读取名为 `example.h5` 的h5文件,然后将其中的所有数据转换为.mat格式并保存在名为 `mat_files` 的文件夹中。您只需要更改 `h5_file_path` 和 `mat_file_path` 变量来设置相应的路径即可。
阅读全文
相关推荐
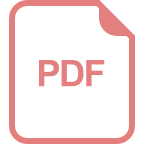


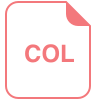
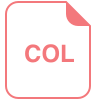
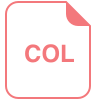



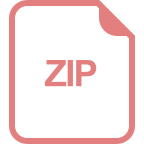
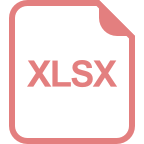
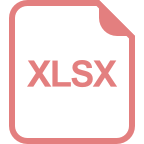
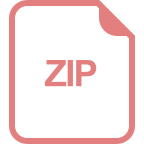