基于pytorch的lstm时间序列预测
时间: 2023-09-30 19:03:23 浏览: 47
首先,你需要准备好你的数据集。数据集应该包括时间序列数据和相应的标签。接下来,你需要将数据集分为训练集和测试集。
然后,你需要定义你的LSTM模型。在PyTorch中,你可以使用`nn.LSTM`模块来定义LSTM网络。你需要指定输入特征的数量、隐藏层的数量和输出特征的数量。
接下来,你需要定义损失函数和优化器。对于时间序列预测,通常使用均方误差(MSE)损失函数。你可以使用`nn.MSELoss`模块来定义MSE损失函数。对于优化器,你可以使用`optim.Adam`模块来定义Adam优化器。
然后,你需要编写训练代码。在每个epoch中,你需要将数据输入到LSTM模型中,并计算损失。然后,你需要使用优化器来更新模型参数。
最后,你需要编写测试代码。在测试中,你需要将测试数据输入到LSTM模型中,并使用训练好的模型计算预测值。然后,你可以使用预测值和实际值之间的MSE来评估模型的性能。
下面是一个基于PyTorch的LSTM时间序列预测的示例代码:
```python
import torch
import torch.nn as nn
import torch.optim as optim
import numpy as np
# 准备数据
# 时间序列数据
data = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
# 标签
labels = np.array([2, 4, 6, 8, 10, 12, 14, 16, 18, 20])
# 将数据集划分为训练集和测试集
train_data = data[:8]
train_labels = labels[:8]
test_data = data[8:]
test_labels = labels[8:]
# 定义LSTM模型
class LSTM(nn.Module):
def __init__(self, input_size, hidden_size, output_size):
super(LSTM, self).__init__()
self.hidden_size = hidden_size
self.lstm = nn.LSTM(input_size, hidden_size)
self.linear = nn.Linear(hidden_size, output_size)
def forward(self, input):
output, (hidden, cell) = self.lstm(input.view(len(input), 1, -1))
output = self.linear(output.view(len(input), -1))
return output[-1]
# 定义损失函数和优化器
model = LSTM(1, 10, 1)
criterion = nn.MSELoss()
optimizer = optim.Adam(model.parameters(), lr=0.01)
# 训练模型
for epoch in range(1000):
inputs = torch.from_numpy(train_data).float()
labels = torch.from_numpy(train_labels).float()
optimizer.zero_grad()
outputs = model(inputs)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
if epoch % 100 == 0:
print("Epoch {} Loss: {:.4f}".format(epoch, loss.item()))
# 测试模型
test_inputs = torch.from_numpy(test_data).float()
expected_outputs = torch.from_numpy(test_labels).float()
with torch.no_grad():
predicted_outputs = model(test_inputs)
test_loss = criterion(predicted_outputs, expected_outputs)
print("Test Loss: {:.4f}".format(test_loss.item()))
print("Expected Outputs: {}".format(expected_outputs))
print("Predicted Outputs: {}".format(predicted_outputs))
```
这段代码演示了如何使用PyTorch实现基于LSTM的时间序列预测。在这个示例中,我们使用一个简单的时间序列数据集来训练和测试模型。你可以将这个示例代码作为参考,根据你的数据集进行必要的修改。
相关推荐
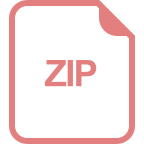














