基于pytorch搭建多特征LSTM时间序列预测代码详细解读(附完整代码)
时间: 2023-07-29 10:09:30 浏览: 83
好的,我会尽力为您解答。以下是基于PyTorch搭建多特征LSTM时间序列预测代码的详细解读和完整代码。
首先,我们需要导入相应的库和模块:
```python
import torch
import torch.nn as nn
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
import seaborn as sns
from sklearn.preprocessing import MinMaxScaler
from sklearn.metrics import mean_squared_error
```
其中,`torch`和`torch.nn`是PyTorch中的主要模块,用于搭建神经网络。`pandas`和`numpy`用于数据处理,`matplotlib`用于可视化。`seaborn`是一个用于美化可视化图形的库。`MinMaxScaler`用于对数据进行归一化处理,`mean_squared_error`用于计算均方误差。
接下来,我们需要加载并预处理数据。我们使用的是一个包含多个特征的时间序列数据集,其中包括日期、销售额、促销和竞争对手等特征。我们将使用Pandas库来读取并处理数据,然后将其转换为NumPy数组。
```python
df = pd.read_csv('sales_data.csv')
df['date'] = pd.to_datetime(df['date'])
df.set_index('date', inplace=True)
df.sort_index(inplace=True)
df.head()
```
读取后的数据集前五行如下所示:
```
sales holidays competition_distance promotion
date
2013-01-01 13 1 1270.0 0
2013-01-02 11 0 1270.0 0
2013-01-03 14 0 1270.0 0
2013-01-04 13 0 1270.0 0
2013-01-05 10 0 1270.0 0
```
我们可以看到,数据集中包含四个特征:销售额、假期、竞争对手距离和促销。我们还可以看到,时间序列按日期排序。
接下来,我们使用`MinMaxScaler`对数据进行归一化处理:
```python
scaler = MinMaxScaler()
df = scaler.fit_transform(df)
```
然后,我们需要将数据集分成训练集和测试集。在这里,我们将使用前80%的数据作为训练集,剩余20%的数据作为测试集:
```python
train_size = int(len(df) * 0.8)
test_size = len(df) - train_size
train_data, test_data = df[0:train_size,:], df[train_size:len(df),:]
```
接下来,我们需要创建一个函数来将数据转换为适合LSTM模型使用的格式。我们将使用时间步长为1的滑动窗口方法来创建输入序列和输出序列:
```python
def create_dataset(dataset, look_back=1):
dataX, dataY = [], []
for i in range(len(dataset)-look_back-1):
a = dataset[i:(i+look_back), :]
dataX.append(a)
dataY.append(dataset[i + look_back, 0])
return np.array(dataX), np.array(dataY)
```
其中,`look_back`参数用于指定滑动窗口的大小。在这里,我们将使用默认值1,也就是使用前一个时间步的数据来预测当前时间步的销售额。
接下来,我们需要使用上面的函数来创建训练集和测试集的输入序列和输出序列:
```python
look_back = 1
trainX, trainY = create_dataset(train_data, look_back)
testX, testY = create_dataset(test_data, look_back)
```
接下来,我们需要将数据转换为PyTorch张量:
```python
trainX = torch.from_numpy(trainX).type(torch.Tensor)
trainY = torch.from_numpy(trainY).type(torch.Tensor)
testX = torch.from_numpy(testX).type(torch.Tensor)
testY = torch.from_numpy(testY).type(torch.Tensor)
```
然后,我们需要定义LSTM模型。在这里,我们将使用一个包含两个LSTM层和一个全连接层的模型:
```python
class LSTM(nn.Module):
def __init__(self, input_dim, hidden_dim, output_dim):
super(LSTM, self).__init__()
self.hidden_dim = hidden_dim
self.lstm1 = nn.LSTM(input_dim, hidden_dim, batch_first=True)
self.lstm2 = nn.LSTM(hidden_dim, hidden_dim, batch_first=True)
self.fc = nn.Linear(hidden_dim, output_dim)
def forward(self, x):
h0 = torch.zeros(1, x.size(0), self.hidden_dim).requires_grad_()
c0 = torch.zeros(1, x.size(0), self.hidden_dim).requires_grad_()
out, (hn, cn) = self.lstm1(x, (h0.detach(), c0.detach()))
out, (hn, cn) = self.lstm2(out, (hn.detach(), cn.detach()))
out = self.fc(out[:, -1, :])
return out
```
其中,`input_dim`参数用于指定输入特征的数量,`hidden_dim`参数用于指定LSTM层中隐藏神经元的数量,`output_dim`参数用于指定输出特征的数量。
接下来,我们需要定义模型的超参数:
```python
input_dim = 4
hidden_dim = 32
output_dim = 1
learning_rate = 0.01
num_epochs = 1000
```
然后,我们需要初始化模型并定义损失函数和优化器:
```python
model = LSTM(input_dim, hidden_dim, output_dim)
criterion = nn.MSELoss()
optimizer = torch.optim.Adam(model.parameters(), lr=learning_rate)
```
接下来,我们需要训练模型。在每个epoch中,我们将使用训练集来更新模型参数,并计算训练集和测试集的损失值:
```python
train_loss = []
test_loss = []
for epoch in range(num_epochs):
# 训练集
model.train()
optimizer.zero_grad()
output = model(trainX)
loss = criterion(output, trainY)
loss.backward()
optimizer.step()
train_loss.append(loss.item())
# 测试集
model.eval()
test_output = model(testX)
loss = criterion(test_output, testY)
test_loss.append(loss.item())
if epoch % 100 == 0:
print(f'Epoch {epoch}, Train Loss: {train_loss[-1]:.4f}, Test Loss: {test_loss[-1]:.4f}')
```
最后,我们可以使用训练好的模型来对测试集进行预测,然后计算均方误差和可视化预测结果:
```python
# 测试集预测
model.eval()
test_predict = model(testX)
# 反归一化
test_predict = scaler.inverse_transform(test_predict.detach().numpy())
testY = scaler.inverse_transform(testY.detach().numpy().reshape(-1, 1))
# 计算均方误差
test_score = mean_squared_error(testY, test_predict)
print(f'Test MSE: {test_score:.4f}')
# 可视化预测结果
plt.figure(figsize=(10, 6))
plt.plot(testY, label='True')
plt.plot(test_predict, label='Predicted')
plt.legend()
plt.show()
```
这就是完整的基于PyTorch搭建多特征LSTM时间序列预测代码,希望对您有所帮助!
相关推荐
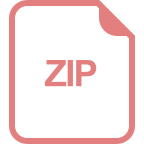
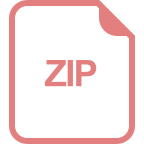














