python五子棋
时间: 2023-06-21 22:24:41 浏览: 76
下面是一个简单的 Python 代码实现五子棋游戏:
```python
import numpy as np
def create_board():
board = np.zeros((15,15))
return board
def place_piece(board, row, col, piece):
board[row][col] = piece
def is_valid_move(board, row, col):
if row < 0 or row >= 15 or col < 0 or col >= 15:
return False
if board[row][col] != 0:
return False
return True
def is_win(board, piece):
# Check rows
for i in range(15):
if np.all(board[i:i+5, :] == piece):
return True
# Check columns
for i in range(15):
if np.all(board[:, i:i+5] == piece):
return True
# Check diagonals
for i in range(11):
for j in range(11):
if np.all(board[i:i+5, j:j+5].diagonal() == piece):
return True
if np.all(board[i:i+5, j:j+5][::-1].diagonal() == piece):
return True
return False
def print_board(board):
symbols = {0: '.', 1: 'X', 2: 'O'}
print(' ' + ' '.join([str(i) for i in range(15)]))
for i in range(15):
row = [symbols[int(x)] for x in board[i]]
print(str(i) + ' ' + ' '.join(row))
def play_game():
board = create_board()
current_player = 1
print_board(board)
while True:
row = int(input("Player " + str(current_player) + ", enter row: "))
col = int(input("Player " + str(current_player) + ", enter column: "))
if is_valid_move(board, row, col):
place_piece(board, row, col, current_player)
print_board(board)
if is_win(board, current_player):
print("Player " + str(current_player) + " wins!")
break
if np.count_nonzero(board) == 225:
print("Game over, it's a tie!")
break
current_player = 3 - current_player
else:
print("Invalid move, try again.")
play_game()
```
这个代码实现了一个简单的五子棋游戏,包括创建棋盘、下棋、判断输赢等功能。您可以运行代码试试看。
相关推荐
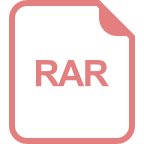
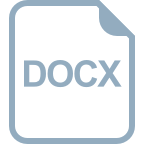










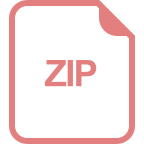
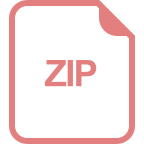