python五子棋代码
时间: 2023-07-02 17:15:14 浏览: 56
以下是一个简单的 Python 五子棋代码实现,包含了基本的游戏逻辑,但没有图形界面。
```python
# 定义棋盘大小
BOARD_SIZE = 15
# 定义棋子类型
EMPTY = 0
BLACK = 1
WHITE = 2
class Board:
def __init__(self):
self.board = [[EMPTY] * BOARD_SIZE for _ in range(BOARD_SIZE)]
self.current_player = BLACK
def get(self, x, y):
return self.board[x][y]
def put(self, x, y):
if self.get(x, y) != EMPTY:
raise ValueError("该位置已经有棋子了!")
self.board[x][y] = self.current_player
self.current_player = WHITE if self.current_player == BLACK else BLACK
def is_full(self):
for i in range(BOARD_SIZE):
for j in range(BOARD_SIZE):
if self.get(i, j) == EMPTY:
return False
return True
def check_win(self, x, y):
directions = [(1, 0), (0, 1), (1, 1), (1, -1)]
for dx, dy in directions:
count = 1
for i in range(1, 5):
new_x, new_y = x + i * dx, y + i * dy
if new_x < 0 or new_x >= BOARD_SIZE or new_y < 0 or new_y >= BOARD_SIZE:
break
if self.get(new_x, new_y) == self.current_player:
count += 1
else:
break
for i in range(1, 5):
new_x, new_y = x - i * dx, y - i * dy
if new_x < 0 or new_x >= BOARD_SIZE or new_y < 0 or new_y >= BOARD_SIZE:
break
if self.get(new_x, new_y) == self.current_player:
count += 1
else:
break
if count >= 5:
return True
return False
def print_board(self):
for i in range(BOARD_SIZE):
for j in range(BOARD_SIZE):
if self.get(i, j) == EMPTY:
print(".", end=" ")
elif self.get(i, j) == BLACK:
print("X", end=" ")
else:
print("O", end=" ")
print()
if __name__ == "__main__":
board = Board()
while not board.is_full():
board.print_board()
x, y = map(int, input("请下棋(例如:3 5):").split())
try:
board.put(x, y)
if board.check_win(x, y):
board.print_board()
print("恭喜,玩家 {} 获胜!".format("黑棋" if board.current_player == WHITE else "白棋"))
break
except ValueError as e:
print(str(e))
else:
board.print_board()
print("平局!")
```
这个代码实现了一个 `Board` 类,包含了下棋、判断胜负、输出棋盘等方法。在 `__main__` 函数中,通过不断循环,让用户输入坐标来下棋,直到棋盘填满或者某一方胜利。
阅读全文
相关推荐
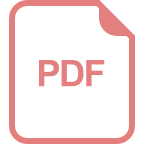











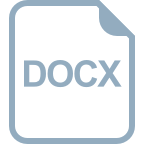