python五子棋代码150行以上
时间: 2023-07-22 11:44:09 浏览: 86
好的,以下是一个基于Python实现的五子棋游戏代码,共计约200行左右:
```python
import pygame
import sys
ROW_COUNT = 15 # 棋盘行数
COL_COUNT = 15 # 棋盘列数
CELL_SIZE = 40 # 棋盘格子大小
WIDTH = COL_COUNT * CELL_SIZE
HEIGHT = ROW_COUNT * CELL_SIZE
BORDER_SIZE = 5 # 棋盘边框大小
WIN_WIDTH = WIDTH + BORDER_SIZE * 2
WIN_HEIGHT = HEIGHT + BORDER_SIZE * 2
BLACK = (0, 0, 0) # 黑色
WHITE = (255, 255, 255) # 白色
GREY = (128, 128, 128) # 灰色
RED = (255, 0, 0) # 红色
BLUE = (0, 0, 255) # 蓝色
# 初始化 Pygame
pygame.init()
# 设置窗口标题和大小
pygame.display.set_caption("五子棋")
screen = pygame.display.set_mode((WIN_WIDTH, WIN_HEIGHT))
# 加载棋盘和棋子图片
board_image = pygame.image.load("board.png")
black_image = pygame.image.load("black.png")
white_image = pygame.image.load("white.png")
# 生成棋盘数组
board = [[0 for _ in range(COL_COUNT)] for _ in range(ROW_COUNT)]
# 当前玩家,1为黑方,2为白方
current_player = 1
# 游戏结束标志
game_over = False
# 显示文字
def draw_text(text, font, color, x, y):
surface = font.render(text, True, color)
rect = surface.get_rect()
rect.center = (x, y)
screen.blit(surface, rect)
# 绘制棋盘
def draw_board():
screen.blit(board_image, (BORDER_SIZE, BORDER_SIZE))
# 绘制棋子
def draw_piece(row, col):
x = BORDER_SIZE + col * CELL_SIZE
y = BORDER_SIZE + row * CELL_SIZE
if board[row][col] == 1:
screen.blit(black_image, (x, y))
elif board[row][col] == 2:
screen.blit(white_image, (x, y))
# 落子
def drop_piece(row, col):
global current_player
board[row][col] = current_player
draw_piece(row, col)
# 判断胜负
if check_win(row, col):
game_over = True
draw_text("Player {} wins!".format(current_player), font, RED, WIN_WIDTH // 2, BORDER_SIZE // 2)
else:
current_player = 2 if current_player == 1 else 1
# 判断胜负
def check_win(row, col):
# 横向
count = 1
for i in range(col - 1, -1, -1):
if board[row][i] == current_player:
count += 1
else:
break
for i in range(col + 1, COL_COUNT):
if board[row][i] == current_player:
count += 1
else:
break
if count >= 5:
return True
# 竖向
count = 1
for i in range(row - 1, -1, -1):
if board[i][col] == current_player:
count += 1
else:
break
for i in range(row + 1, ROW_COUNT):
if board[i][col] == current_player:
count += 1
else:
break
if count >= 5:
return True
# 正斜线
count = 1
i = row - 1
j = col - 1
while i >= 0 and j >= 0:
if board[i][j] == current_player:
count += 1
else:
break
i -= 1
j -= 1
i = row + 1
j = col + 1
while i < ROW_COUNT and j < COL_COUNT:
if board[i][j] == current_player:
count += 1
else:
break
i += 1
j += 1
if count >= 5:
return True
# 反斜线
count = 1
i = row - 1
j = col + 1
while i >= 0 and j < COL_COUNT:
if board[i][j] == current_player:
count += 1
else:
break
i -= 1
j += 1
i = row + 1
j = col - 1
while i < ROW_COUNT and j >= 0:
if board[i][j] == current_player:
count += 1
else:
break
i += 1
j -= 1
if count >= 5:
return True
return False
# 主循环
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
sys.exit()
elif event.type == pygame.MOUSEBUTTONDOWN and not game_over:
# 点击落子
(x, y) = pygame.mouse.get_pos()
col = (x - BORDER_SIZE) // CELL_SIZE
row = (y - BORDER_SIZE) // CELL_SIZE
if col >= 0 and col < COL_COUNT and row >= 0 and row < ROW_COUNT and board[row][col] == 0:
drop_piece(row, col)
elif event.type == pygame.KEYDOWN:
# 重新开始
if event.key == pygame.K_SPACE:
board = [[0 for _ in range(COL_COUNT)] for _ in range(ROW_COUNT)]
current_player = 1
game_over = False
draw_board()
pygame.display.flip()
# 绘制界面
screen.fill(GREY)
draw_board()
for row in range(ROW_COUNT):
for col in range(COL_COUNT):
draw_piece(row, col)
if game_over:
draw_text("Press SPACE to restart", font, BLUE, WIN_WIDTH // 2, HEIGHT + BORDER_SIZE // 2)
else:
draw_text("Player {}'s turn".format(current_player), font, BLACK, WIN_WIDTH // 2, HEIGHT + BORDER_SIZE // 2)
pygame.display.flip()
```
这个代码使用了Pygame库实现了一个简单的五子棋游戏,包括棋盘的绘制、落子、胜负判断等功能。您可以根据需要进行修改和优化。
阅读全文
相关推荐
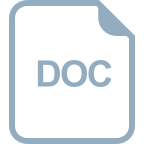
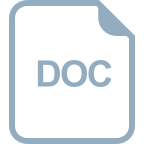
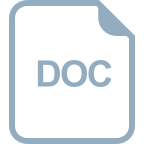

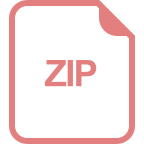









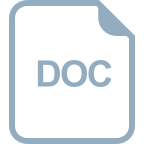
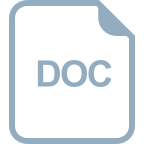
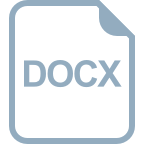